如果一个病人诊断中,他去做检查了,然后要诊断下一个病人,上一个病人检查好了,要继续去诊断,他是插队进入在诊断的这个人的后面对吧 这样怎么实现 java代码
时间: 2024-12-23 18:16:43 浏览: 6
在Java中,如果需要模拟这样的流程,通常会创建一个简单的线程池或者并发模型来处理病人的排队。这里我们可以使用`BlockingQueue`(阻塞队列)来存储病人,并利用多线程来实现医生的工作。下面是一个基本的示例:
```java
import java.util.concurrent.*;
public class Clinic {
private final BlockingQueue<Patient> queue;
private final Thread doctor;
public Clinic() {
this.queue = new LinkedBlockingQueue<>();
this.doctor = new Thread(() -> {
while (true) {
Patient patient = queue.take(); // 病人从队列中取出
System.out.println("Doctor is diagnosing " + patient);
// 这里可以添加实际的诊断过程,例如耗时操作
try {
Thread.sleep(2000); // 模拟诊断时间
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Patient's diagnosis finished");
}
}, "Doctor");
doctor.start(); // 开始医生线程
}
public void registerPatient(Patient patient) {
queue.offer(patient);
System.out.println("Patient " + patient + " added to the queue.");
}
public static void main(String[] args) {
Clinic clinic = new Clinic();
clinic.registerPatient(new Patient("Patient A")); // 先加第一个病人
clinic.registerPatient(new Patient("Patient B")); // 再加第二个病人
}
}
class Patient {
private String name;
public Patient(String name) {
this.name = name;
}
@Override
public String toString() {
return "Patient " + name;
}
}
```
在这个例子中,`registerPatient`方法用于注册病人到队列中,`doctor`线程则不断从队列中取出病人并进行诊断。当有新的病人到来时,他会自动插入队列等待。请注意,这只是一个简化的模型,实际应用中可能还需要考虑其他因素,比如医生休息、病人优先级等。
阅读全文
相关推荐
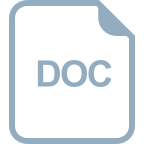
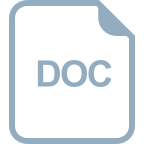
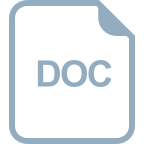
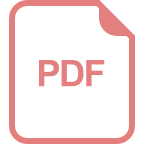
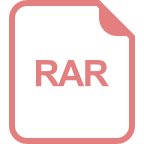
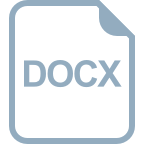
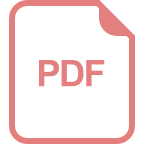
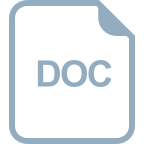
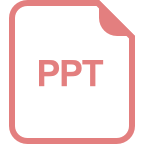
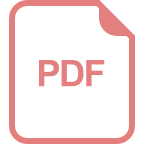
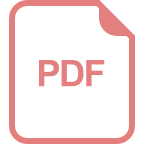
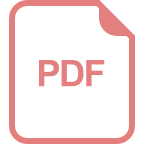
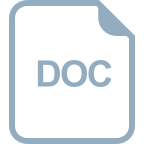
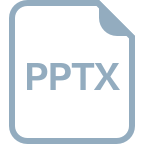
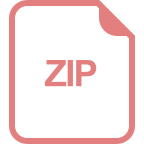