我正在编写一个基于fer2013数据集的深度学习情绪识别项目
时间: 2024-05-20 09:15:05 浏览: 119
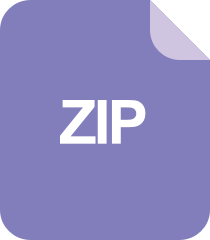
使用带有 keras CNN模型和 openCV 的fer2013 数据集检测面部情绪。

很棒!fer2013数据集是一个非常流行的情绪识别数据集,包含了7种不同的情绪分类,包括愤怒、厌恶、恐惧、快乐、悲伤、惊讶和中性。在这个项目中,您可以使用各种深度学习技术,如卷积神经网络(CNN)或循环神经网络(RNN),来构建一个情绪分类模型。您还可以使用一些预处理技术,如数据增强和标准化,来提高模型的性能。祝您好运!
阅读全文
相关推荐
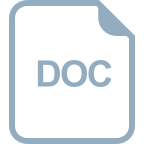
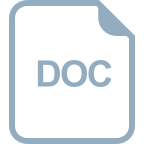
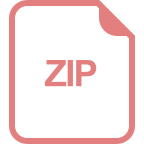
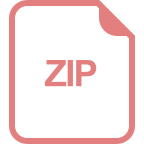
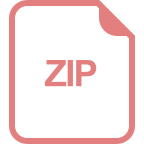
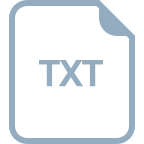
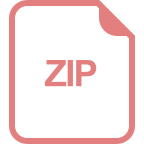
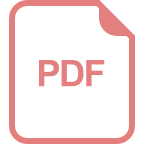
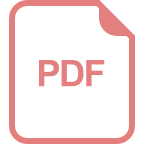
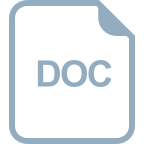
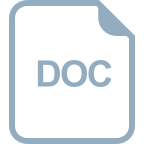
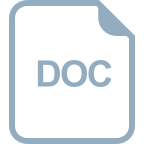
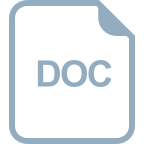




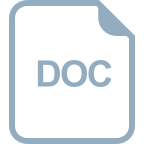