C#如何使用反射获取Unity Debug类
时间: 2024-10-04 15:01:53 浏览: 20
在C#中,反射是一种强大的工具,允许你在运行时检查和操作程序的结构。如果你想要通过反射获取Unity的Debug类,可以按照以下步骤进行:
```csharp
// 使用Assembly类查找Unity引擎的类型
Type debugType = Assembly.GetAssembly(typeof(UnityEngine.Object)).GetType("UnityEngine.Debug");
// 确保找到的是Debug类并且不是其派生类
if (debugType == typeof(UnityEngine.Debug)) {
// 可以使用Type对象访问Debug类的静态成员或方法
Console.WriteLine(debugType.InvokeMember("Log", BindingFlags.Public | BindingFlags.Static, null, null, new object[] { "This is a debug message." }));
}
```
这段代码首先从包含UnityEngine命名空间的Assembly中获取类型,然后确认获取到的就是UnityEngine.Debug类。如果成功,你可以像操作普通类一样使用`InvokeMember`或`GetProperty`等方法来调用Debug类的方法或获取属性。
相关问题
反射获取Unity的Debug类
反射在Unity中是一种强大的工具,它允许你在运行时检查、修改和动态地创建对象,包括系统类如`Debug`。要通过反射获取Unity的`Debug`类,你可以按照以下步骤操作:
1. 首先,你需要导入`System.Reflection`命名空间,这是C#中用于处理反射的库。
```csharp
using System.Reflection;
```
2. 然后,在需要使用`Debug`的地方,你可以使用`Assembly.GetAssembly(typeof(Debug))`获取包含`Debug`类的`Assembly`对象,接着通过`Assembly.GetTypes()`获取所有类型的列表,再在这个列表里查找`Debug`类型。
```csharp
Type debugType = Assembly.GetAssembly(typeof(Debug)).GetType("UnityEngine.Debug");
```
3. 这样,你就得到了`Debug`类型的实例,可以使用它的各种方法,比如`LogError()`或`Assert()`等。
```csharp
MethodInfo logMethod = debugType.GetMethod("LogError", new Type[] { typeof(string) });
logMethod.Invoke(null, new object[] { "This is a logged error message." });
```
unity debug怎么使用
在Unity中,你可以使用以下几种方式进行调试:
1. 使用Debug.Log输出日志信息:在你的代码中使用Debug.Log来输出一些调试信息。例如:
```csharp
Debug.Log("这是一个调试信息");
```
这样会在Unity的控制台窗口中打印出相应的日志信息。
2. 使用断点调试:在代码中设置断点,以便在运行时暂停程序的执行,你可以逐行查看代码的执行情况,并观察变量的值。在Unity中,你可以在脚本中点击行号的左侧空白区域来设置断点。
3. 使用条件断点:条件断点是一种特殊类型的断点,只有当满足特定的条件时才会触发断点。在Unity中,你可以在设置断点后右键点击断点,然后选择“条件”,在弹出的对话框中输入条件表达式。
4. 使用调试器工具:Unity提供了内置的调试器工具,可以帮助你更方便地调试代码。你可以通过菜单栏的“Window -> Analysis -> Profiler”来打开Profiler窗口,它可以帮助你分析代码的性能。
希望这些方法能够帮助你更好地进行Unity的调试!如果有任何问题,请随时提问。
相关推荐
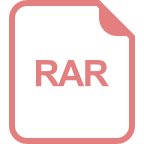
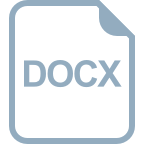
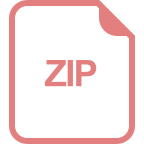












