基于Android的通讯录代码
时间: 2024-01-08 14:52:55 浏览: 37
以下是一个简单的基于 Android 平台的通讯录应用程序的代码示例:
MainActivity.java
```
import android.content.Intent;
import android.database.Cursor;
import android.os.Bundle;
import android.provider.ContactsContract;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ListView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
private ListView listView;
private ArrayList<Contact> contacts = new ArrayList<>();
private ContactAdapter contactAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView = findViewById(R.id.listView);
Cursor cursor = getContentResolver().query(ContactsContract.CommonDataKinds.Phone.CONTENT_URI, null, null, null, null);
while (cursor.moveToNext()) {
String name = cursor.getString(cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.DISPLAY_NAME));
String phoneNumber = cursor.getString(cursor.getColumnIndex(ContactsContract.CommonDataKinds.Phone.NUMBER));
contacts.add(new Contact(name, phoneNumber));
}
cursor.close();
contactAdapter = new ContactAdapter(this, contacts);
listView.setAdapter(contactAdapter);
listView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(MainActivity.this, ContactActivity.class);
intent.putExtra("name", contacts.get(position).getName());
intent.putExtra("phoneNumber", contacts.get(position).getPhoneNumber());
startActivity(intent);
}
});
}
}
```
Contact.java
```
public class Contact {
private String name;
private String phoneNumber;
public Contact(String name, String phoneNumber) {
this.name = name;
this.phoneNumber = phoneNumber;
}
public String getName() {
return name;
}
public String getPhoneNumber() {
return phoneNumber;
}
}
```
ContactAdapter.java
```
import android.content.Context;
import android.support.annotation.NonNull;
import android.support.annotation.Nullable;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ArrayAdapter;
import android.widget.TextView;
import java.util.ArrayList;
public class ContactAdapter extends ArrayAdapter<Contact> {
private Context context;
private ArrayList<Contact> contacts;
public ContactAdapter(@NonNull Context context, ArrayList<Contact> contacts) {
super(context, 0, contacts);
this.context = context;
this.contacts = contacts;
}
@NonNull
@Override
public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) {
View listItemView = convertView;
if (listItemView == null) {
listItemView = LayoutInflater.from(context).inflate(R.layout.contact_item, parent, false);
}
Contact currentContact = contacts.get(position);
TextView nameTextView = listItemView.findViewById(R.id.nameTextView);
nameTextView.setText(currentContact.getName());
TextView phoneNumberTextView = listItemView.findViewById(R.id.phoneNumberTextView);
phoneNumberTextView.setText(currentContact.getPhoneNumber());
return listItemView;
}
}
```
activity_main.xml
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingTop="16dp"
tools:context=".MainActivity">
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</RelativeLayout>
```
contact_item.xml
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<TextView
android:id="@+id/nameTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/phoneNumberTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/nameTextView"
android:textSize="14sp" />
</RelativeLayout>
```
ContactActivity.java
```
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class ContactActivity extends AppCompatActivity {
private TextView nameTextView;
private TextView phoneNumberTextView;
private Button callButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_contact);
nameTextView = findViewById(R.id.nameTextView);
phoneNumberTextView = findViewById(R.id.phoneNumberTextView);
callButton = findViewById(R.id.callButton);
Intent intent = getIntent();
String name = intent.getStringExtra("name");
String phoneNumber = intent.getStringExtra("phoneNumber");
nameTextView.setText(name);
phoneNumberTextView.setText(phoneNumber);
callButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String phoneNumber = phoneNumberTextView.getText().toString();
Intent intent = new Intent(Intent.ACTION_DIAL);
intent.setData(Uri.parse("tel:" + phoneNumber));
startActivity(intent);
}
});
}
}
```
activity_contact.xml
```
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingTop="16dp"
tools:context=".ContactActivity">
<TextView
android:id="@+id/nameTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:textStyle="bold" />
<TextView
android:id="@+id/phoneNumberTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/nameTextView"
android:textSize="14sp" />
<Button
android:id="@+id/callButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/phoneNumberTextView"
android:text="Call" />
</RelativeLayout>
```
相关推荐
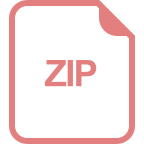
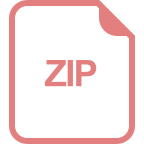














