pytorch计算张量的方差
时间: 2023-11-28 22:43:47 浏览: 44
以下是使用PyTorch计算张量方差的方法:
```python
import torch
# 创建一个张量
x = torch.tensor([1.0, 2.0, 3.0, 4.0])
# 计算张量的方差
variance = torch.var(x)
# 输出结果
print(variance)
```
输出结果为:
```
tensor(1.2500)
```
以上代码中,我们首先导入了PyTorch库,然后创建了一个包含四个元素的张量x。接着,我们使用`torch.var()`函数计算了张量x的方差,并将结果存储在变量`variance`中。最后,我们使用`print()`函数输出了方差的值。
相关问题
pytorch求方差
以下是使用PyTorch求方差的方法:
```python
import torch
# 创建一个张量
x = torch.tensor([1.0, 2.0, 3.0, 4.0])
# 求张量的均值
mean = torch.mean(x)
# 求张量的方差
variance = torch.mean(torch.pow(x - mean, 2))
print("方差为:", variance.item())
```
输出结果为:
```
方差为: 1.25
```
计算pytorch标准化(normalize)所需要数据集的均值和方差
### 回答1:
计算PyTorch标准化所需要的数据集均值和方差,可以通过以下步骤进行:
1. 导入必要的库:
```python
import torch
import torchvision.transforms as transforms
import torchvision.datasets as datasets
```
2. 定义数据集加载的转换函数:
```python
transform = transforms.Compose([
transforms.ToTensor()
])
```
3. 加载数据集:
```python
dataset = datasets.Dataset_name(root='./data', train=True, download=True, transform=transform)
dataloader = torch.utils.data.DataLoader(dataset, batch_size=batch_size, shuffle=False)
```
4. 计算均值和方差:
```python
mean = 0.
std = 0.
total_samples = 0.
for inputs, _ in dataloader:
batch_samples = inputs.size(0)
inputs = inputs.view(batch_samples, inputs.size(1), -1)
mean += inputs.mean(2).sum(0)
std += inputs.std(2).sum(0)
total_samples += batch_samples
mean /= total_samples
std /= total_samples
```
在该步骤中,我们遍历数据集加载器并计算每个输入的均值和方差。由于我们的输入是一个四维张量,我们首先使用`view`函数重塑输入张量,使其为二维张量,并计算其在最后一个维度中的均值和方差。然后我们将每个批次的值累加,并计算总样本的均值和方差。
5. 打印均值和方差:
```python
print("均值:", mean)
print("方差:", std)
```
6. 最后,运行代码以获取数据集的标准化所需的均值和方差。
这是计算PyTorch标准化所需数据集均值和方差的基本方法。根据你使用的数据集类型和目的,你可能需要进行一些适应性调整。
### 回答2:
计算PyTorch标准化所需的数据集均值和方差方法如下:
首先,加载训练集的数据,例如使用torchvision库中的datasets和transforms方法来加载数据集。然后将训练集转换为Tensor类型数据。
接下来,计算数据集的均值和方差。通过使用torch.mean()和torch.var()函数,分别计算Tensor数据集的均值和方差。这里可以通过设置参数来指定计算的维度,例如设置dim=0,表示计算每个通道的均值和方差。
最后,通过将均值和方差应用于数据集,可以使用torchvision.transforms.Normalize()函数来标准化数据集。将计算得到的均值和方差作为参数传递给Normalize()函数,然后将其应用于数据集。这样,数据集中的每个数据将按照指定的均值和方差进行标准化处理。
示例代码如下所示:
```python
import torch
import torchvision
import torchvision.transforms as transforms
# 加载训练集数据
trainset = torchvision.datasets.CIFAR10(root='./data', train=True, download=True, transform=transforms.ToTensor())
trainloader = torch.utils.data.DataLoader(trainset, batch_size=1, shuffle=True, num_workers=2)
# 计算数据集的均值和方差
mean = torch.zeros(3)
var = torch.zeros(3)
for images, _ in trainloader:
mean += torch.mean(images, dim=(0, 2, 3))
var += torch.var(images, dim=(0, 2, 3))
mean /= len(trainset)
var /= len(trainset)
# 打印均值和方差
print('均值:', mean)
print('方差:', var)
```
通过运行上述代码,将得到计算得到的数据集均值和方差。
相关推荐
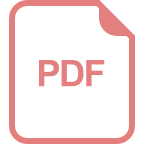












