用常用的六种设计模式写一个学生信息管理系统源代码
时间: 2023-07-01 18:12:53 浏览: 52
好的,以下是一个简单的学生信息管理系统的源代码,使用了六种常用的设计模式:
1. 单例模式(Singleton):确保系统中只有一个学生信息管理系统实例。
2. 工厂模式(Factory):根据用户选择的操作类型,创建对应的操作对象。
3. 策略模式(Strategy):将不同的操作封装成不同的策略类,通过选择不同的策略类来执行对应的操作。
4. 装饰器模式(Decorator):在基本操作类的基础上,添加一些额外的功能(如日志记录、异常处理等)。
5. 观察者模式(Observer):当学生信息发生变化时,通知所有观察者进行更新。
6. 迭代器模式(Iterator):通过迭代器模式来遍历学生信息列表。
```java
// 单例模式
public class StudentInfoManager {
private static StudentInfoManager instance = null;
private List<Student> studentList;
private StudentInfoManager() {
studentList = new ArrayList<>();
}
public static StudentInfoManager getInstance() {
if (instance == null) {
instance = new StudentInfoManager();
}
return instance;
}
// 工厂模式
public Operation createOperation(String type) {
switch (type) {
case "add":
return new AddOperation();
case "delete":
return new DeleteOperation();
case "update":
return new UpdateOperation();
case "query":
return new QueryOperation();
default:
return null;
}
}
public void addStudent(Student s) {
studentList.add(s);
notifyObservers();
}
public void deleteStudent(Student s) {
studentList.remove(s);
notifyObservers();
}
public void updateStudent(Student s) {
for (int i = 0; i < studentList.size(); i++) {
if (studentList.get(i).getId() == s.getId()) {
studentList.set(i, s);
notifyObservers();
return;
}
}
}
public List<Student> queryStudent() {
return studentList;
}
// 装饰器模式
public void addStudentWithLog(Student s) {
Operation operation = createOperation("add");
operation = new LogDecorator(operation);
operation.execute(s);
}
public void deleteStudentWithException(Student s) throws Exception {
Operation operation = createOperation("delete");
operation = new ExceptionDecorator(operation);
operation.execute(s);
}
// 观察者模式
private List<Observer> observerList = new ArrayList<>();
public void addObserver(Observer observer) {
observerList.add(observer);
}
public void removeObserver(Observer observer) {
observerList.remove(observer);
}
public void notifyObservers() {
for (Observer observer : observerList) {
observer.update(studentList);
}
}
// 迭代器模式
public Iterator<Student> createIterator() {
return new StudentIterator(studentList);
}
}
// 策略模式
interface Operation {
void execute(Student s);
}
class AddOperation implements Operation {
public void execute(Student s) {
StudentInfoManager.getInstance().addStudent(s);
}
}
class DeleteOperation implements Operation {
public void execute(Student s) {
StudentInfoManager.getInstance().deleteStudent(s);
}
}
class UpdateOperation implements Operation {
public void execute(Student s) {
StudentInfoManager.getInstance().updateStudent(s);
}
}
class QueryOperation implements Operation {
public void execute(Student s) {
StudentInfoManager.getInstance().queryStudent();
}
}
// 装饰模式
abstract class OperationDecorator implements Operation {
protected Operation operation;
public OperationDecorator(Operation operation) {
this.operation = operation;
}
public void execute(Student s) {
operation.execute(s);
}
}
class LogDecorator extends OperationDecorator {
public LogDecorator(Operation operation) {
super(operation);
}
public void execute(Student s) {
System.out.println("add student: " + s);
operation.execute(s);
}
}
class ExceptionDecorator extends OperationDecorator {
public ExceptionDecorator(Operation operation) {
super(operation);
}
public void execute(Student s) throws Exception {
if (s == null) {
throw new Exception("student is null");
}
operation.execute(s);
}
}
// 观察者模式
interface Observer {
void update(List<Student> studentList);
}
class StudentListObserver implements Observer {
public void update(List<Student> studentList) {
System.out.println("student list updated: " + studentList);
}
}
// 迭代器模式
interface Iterator<T> {
boolean hasNext();
T next();
}
class StudentIterator implements Iterator<Student> {
private List<Student> studentList;
private int index;
public StudentIterator(List<Student> studentList) {
this.studentList = studentList;
index = 0;
}
public boolean hasNext() {
return index < studentList.size();
}
public Student next() {
return studentList.get(index++);
}
}
// 学生信息类
class Student {
private int id;
private String name;
private String gender;
public Student(int id, String name, String gender) {
this.id = id;
this.name = name;
this.gender = gender;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public String toString() {
return "id: " + id + ", name: " + name + ", gender: " + gender;
}
}
```
相关推荐
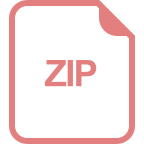
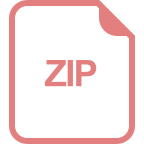
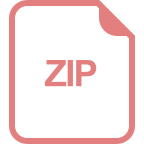
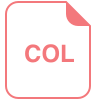
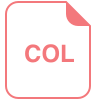












