设计模式在C语言中的应用与实践
发布时间: 2024-02-03 14:37:37 阅读量: 45 订阅数: 25 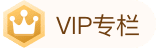
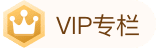
# 1. 设计模式基础
## 1.1 设计模式概述
设计模式是一套被广泛接受的经验总结,它提供了解决软件设计问题的已验证的方法和解决方案。设计模式可以帮助开发人员以一种可靠和可复用的方式构建软件。它们提供了一种共享的词汇和知识体系,使团队成员更容易沟通和理解彼此的设计决策。设计模式并非通用解决方案,而是针对特定问题给出的一种经过验证的解决方法。
## 1.2 设计模式的分类
设计模式可以分为三大类别:创建型模式、结构型模式和行为型模式。
1. **创建型模式**关注如何创建对象,这些模式提供了创建对象的机制,以降低系统中对象的实例化的复杂性。常见的创建型模式有:单例模式、工厂模式、原型模式和抽象工厂模式。
2. **结构型模式**关注如何将对象和类组装成更大的结构,并同时保持结构的灵活和高效性。常见的结构型模式有:适配器模式、桥接模式和组合模式。
3. **行为型模式**关注对象之间的通信和协作,以及分配职责和行为的方式。常见的行为型模式有:策略模式、观察者模式和迭代器模式。
## 1.3 设计模式在软件开发中的重要性
设计模式在软件开发中扮演着重要角色,具有以下几点重要性:
1. **提高代码重用性**:设计模式通过提供经过验证的解决方案,使开发人员能够重用代码,从而节省时间和资源。
2. **增强代码可读性**:设计模式提供了一套共享的词汇和知识体系,使得团队成员更容易沟通和理解彼此的设计决策。
3. **降低软件维护成本**:设计模式通过提供一种可靠且可复用的方式构建软件,使得软件易于维护和扩展。
4. **加速开发过程**:设计模式提供了经过验证的解决方案,使开发人员能够快速构建软件,减少了重复的思考和试错过程。
设计模式的正确应用能够提高软件开发的效率和质量,因此在 C 语言项目中深入理解和应用设计模式是非常必要的。在接下来的章节中,我们将重点介绍 C 语言中常用的设计模式,并提供实际的应用案例。
# 2. C 语言中常用的设计模式
在 C 语言项目中,设计模式是非常常见且有用的。接下来,我们将介绍一些在 C 语言中常用的设计模式,并提供实际的代码示例来帮助读者更好地理解这些模式的应用和实践。
### 2.1 单例模式在 C 语言中的实现
单例模式是一种常见的设计模式,它保证一个类仅有一个实例,并提供一个访问该实例的全局访问点。在 C 语言中,可以通过静态变量和静态方法来实现单例模式。
```c
#include <stdio.h>
typedef struct {
int data;
} Singleton;
static Singleton instance; // 静态变量作为单例实例
Singleton* getSingletonInstance() {
return &instance;
}
void singletonExample() {
Singleton* singleton1 = getSingletonInstance();
Singleton* singleton2 = getSingletonInstance();
singleton1->data = 10;
printf("singleton1->data: %d\n", singleton1->data); // 输出为 10
printf("singleton2->data: %d\n", singleton2->data); // 输出为 10,因为是同一个实例
}
int main() {
singletonExample();
return 0;
}
```
**代码总结:** 上述代码演示了如何在 C 语言中实现单例模式。通过静态变量 `instance` 和静态方法 `getSingletonInstance` 来创建单例实例,并在 `singletonExample` 函数中演示了单例实例的使用。
**结果说明:** 执行 `singletonExample` 函数后,可以看到 `singleton1->data` 和 `singleton2->data` 的值相同,因为它们是同一个实例的不同引用。
### 2.2 工厂模式在 C 语言中的应用
工厂模式是一种创建型模式,它提供一个创建对象的接口,但允许子类决定实例化哪个类。在 C 语言中,使用函数指针和条件判断可以实现工厂模式。
```c
#include <stdio.h>
typedef struct {
int type;
void (*print)(void);
} Product;
void product1_print() {
printf("This is product1\n");
}
void product2_print() {
printf("This is product2\n");
}
Product* createProduct(int type) {
Product* product = (Product*)malloc(sizeof(Product));
product->type = type;
if (type == 1) {
product->print = product1_print;
} else {
product->print = product2_print;
}
return product;
}
void factoryExample() {
Product* product1 = createProduct(1);
Product* product2 = createProduct(2);
product1->print(); // 输出 "This is product1"
product2->print(); // 输出 "This is product2"
}
int main() {
factoryExample();
return 0;
}
```
**代码总结:** 上述代码演示了如何在 C 语言中实现工厂模式。通过 `createProduct` 函数来创建产品实例,并根据需求设置不同的类型和打印函数,然后在 `factoryExample` 函数中演示了产品实例的使用。
**结果说明:** 执行 `factoryExample` 函数后,可以看到根据类型创建的产品实例,分别调用对应的打印函数进行输出。
### 2.3 装饰器模式的 C 语言实践
装饰器模式允许向对象动态添加新功能,是一种结构型设计模式。在 C 语言中,可以通过函数指针和组合来实现装饰器模式。
```c
#include <stdio.h>
typedef struct {
void (*print)(void);
} Component;
void component_print() {
printf("This is the original component\n");
}
typedef struct {
Component component;
void (*print)(void);
} Decorator;
void decorator_print() {
printf("This is the added functionality\n");
}
void addDecorator(Component* component, void (*decorator)()) {
component->print = component_print;
decorator(); // 执行装饰器中的功能
}
void decoratorExample() {
Component component;
addDecorator(&component, decorator_print);
component.print(); // 输出 "This is the original component"
}
int main() {
decoratorExample();
return 0;
}
```
**代码总结:** 上述代码演示了如何在 C 语言中实现装饰器模式。通过 `addDecorator` 函数来向组件动态添加新功能,然后在 `decoratorExample` 函数中演示了装饰后的组件的使用。
**结果说明:** 执行 `decoratorExample` 函数后,可以看到组件在被装饰后,输出了原始组件的内容并执行了装饰器中的功能。
通过以上示例,我们可以清晰地了解在 C 语言中常用的设计模式及其实际的应用场景和代码实现。接下来,我们将深入探讨更多设计模式在 C 语言中的应用与实践。
# 3. C 语言中创建型设计模式的实践
在本章中,将深入探讨在 C 语言中创建型设计模式的具体实践,包括原型模式的应用与实践、建造者模式的实现方式以及抽象工厂模式在 C 语言中的应用案例。我们将通过详细的代码示例和解释,帮助读者深入理解这些设计模式在 C 语言项目中的实际应用。
#### 3.1 原型模式的应用与实践
原型模式是一种对象创建型设计模式,它允许对象在创建另一个对象时,通过复制自身来实现。在 C 语言中,原型模式经常用于通过复制现有对象来创建新对象,而无需依赖对象的具体类。
下面是一个在 C 语言中使用原型模式的简单示例:
```c
#include <stdio.h>
#include <string.h>
// 原型接口
typedef struct {
void* (*clone)(void* self);
void (*printInfo)(void* self);
} Prototype;
// 具体原型
typedef struct {
char name[20];
Prototype prototype; // 嵌入原型接口
} ConcretePrototype;
void* concretePrototypeClone(void* self) {
ConcretePrototype* concrete = (ConcretePrototype*)self;
ConcretePrototype* newPrototype = malloc(sizeof(ConcretePrototype));
memcpy(newPrototype, concrete, sizeof(ConcretePrototype));
return newPrototype;
}
void concretePrototypePrintInfo(void* self) {
ConcretePrototype* concrete = (ConcretePrototype*)self;
printf("Concrete Prototype: %s\n", concrete->name);
}
void concretePrototypeInit(ConcretePrototype* self, char* name) {
strcpy(self->name, name);
self->prototype.clone = concretePrototypeClone;
self->prototype.printInfo = concretePrototypePrintInfo;
}
int main() {
ConcretePrototype concrete1;
concretePrototypeInit(&concrete1, "Prototype 1");
// 克隆原型对象
ConcretePrototype concrete2 = *((ConcretePrototype*)concrete1.prototype.clone(&concrete1));
concrete2.prototype.printInfo(&concrete2);
return 0;
}
```
在上面的示例中,我们定义了一个原型接口 `Prototype`,并实现了具体原型 `ConcretePrototype`。通过使用 `clone` 方法,我们能够复制原型对象来创建新的对象,而无需依赖具体的类。
#### 3.2 建造者模式的实现方式
建造者模式是一种对象创建型设计模式,它允许客户端通过指定需要建造的类型和内容,来创建复杂的对象。在 C 语言中,建造者模式常常用于创建包含多个组件的复杂对象,同时将对象的构建过程与表示分离。
下面是一个在 C 语言中使用建造者模式的简单示例:
```c
#include <stdio.h>
// 产品类
typedef struct {
int part1;
char part2[20];
} Product;
// 建造者接口
typedef struct {
void (*buildPart1)(void* self, int value);
void (*buildPart2)(void* self, char* value);
Product (*getResult)(void* self);
} Builder;
// 具体建造者
typedef struct {
Product product;
Builder builder; // 嵌入建造者接口
} ConcreteBuilder;
void concreteBuilderBuildPart1(void* self, int value) {
ConcreteBuilder* concrete = (ConcreteBuilder*)self;
concrete->product.part1 = value;
}
void concreteBuilderBuildPart2(void* self, char* value) {
ConcreteBuilder* concrete = (ConcreteBuilder*)self;
strcpy(concrete->product.part2, value);
}
Product concreteBuilderGetResult(void* self) {
ConcreteBuilder* concrete = (ConcreteBuilder*)self;
return concrete->product;
}
void c
```
0
0
相关推荐
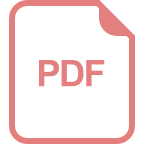
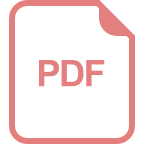
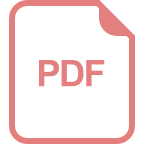
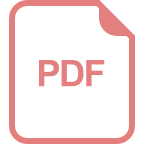
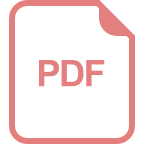
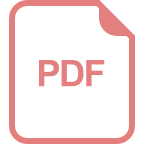
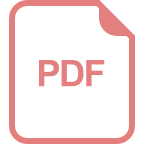
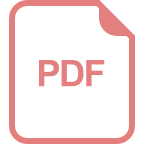