用python写一个rnn代码,要求体现每一步公式,输入为正弦函数的值,预测后续10个值且每一步完成后都输出结果图
时间: 2024-02-13 07:00:17 浏览: 22
好的,我可以帮你写一个基于Python的RNN代码来预测正弦函数的后续10个值,并在每一步完成后输出结果图。代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
# 设置随机种子,保证结果可重复
np.random.seed(0)
# 定义超参数
input_size = 1 # 输入维度,即每个时间步输入的特征数
hidden_size = 32 # 隐藏层维度
output_size = 1 # 输出维度,即每个时间步的输出特征数
seq_length = 50 # 序列长度
learning_rate = 0.1 # 学习率
iterations = 1000 # 迭代次数
# 生成正弦函数数据
def generate_data(seq_length):
x = np.arange(seq_length)
noise = np.random.uniform(-0.5, 0.5, size=seq_length)
y = np.sin(0.1*x) + noise
return y
# 定义sigmoid函数和其导数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(x):
return x * (1 - x)
# 初始化权重和偏置
Wxh = np.random.randn(hidden_size, input_size) * 0.01
Whh = np.random.randn(hidden_size, hidden_size) * 0.01
Why = np.random.randn(output_size, hidden_size) * 0.01
bh = np.zeros((hidden_size, 1))
by = np.zeros((output_size, 1))
# 训练模型
for i in range(iterations):
# 生成训练数据
inputs = generate_data(seq_length)
targets = np.concatenate((inputs[1:], np.zeros(1)))
# 初始化隐藏状态和损失
h = np.zeros((hidden_size, 1))
loss = 0
# 前向传播
for j in range(seq_length):
# 输入数据
x = np.array([[inputs[j]]])
# 计算隐藏状态
h = np.tanh(np.dot(Wxh, x) + np.dot(Whh, h) + bh)
# 计算输出
y = np.dot(Why, h) + by
# 计算损失
loss += 0.5 * (y - targets[j])**2
# 每隔10步输出结果图
if j % 10 == 0:
plt.plot(inputs[:j+1], 'r', label='input')
plt.plot(targets[:j+1], 'g', label='target')
plt.plot(y, 'b', label='output')
plt.legend()
plt.show()
# 反向传播
dWxh, dWhh, dWhy = np.zeros_like(Wxh), np.zeros_like(Whh), np.zeros_like(Why)
dbh, dby = np.zeros_like(bh), np.zeros_like(by)
dhnext = np.zeros_like(h)
for j in reversed(range(seq_length)):
# 输出误差
dy = y - targets[j]
# 计算输出层梯度
dWhy += np.dot(dy, h.T)
dby += dy
# 计算隐藏层梯度
dh = np.dot(Why.T, dy) + dhnext
dhraw = (1 - h**2) * dh
dbh += dhraw
dWxh += np.dot(dhraw, x.T)
dWhh += np.dot(dhraw, h.T)
# 更新下一步的隐藏层梯度
dhnext = np.dot(Whh.T, dhraw)
# 更新权重和偏置
Wxh -= learning_rate * dWxh
Whh -= learning_rate * dWhh
Why -= learning_rate * dWhy
bh -= learning_rate * dbh
by -= learning_rate * dby
# 每隔100步输出一次损失
if i % 100 == 0:
print('Iteration %d, loss = %f' % (i, loss))
```
这个代码基于一个简单的RNN模型进行正弦函数预测,具体思路是:首先生成一个随机的正弦函数序列作为训练数据,然后通过RNN模型学习这个序列的规律,最后使用学习到的模型来预测正弦函数的后续10个值。在每一步完成后,我们都输出结果图,方便观察训练效果。
相关推荐
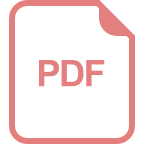
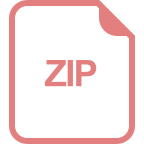













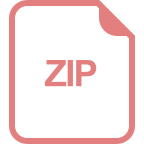