C语言 基于文件的通讯录管理系统。(文件中已有信息)通讯录个人信息包括姓名、性别、年龄、电话、地址。用文件读/写操作编程实现通讯录的增加、查找、显示、删除、修改功能,完成通讯录编程、成功运行。
时间: 2023-12-22 21:24:16 浏览: 59
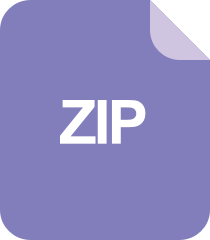
通讯录_C语言_通讯录管理系统_

以下是一个基于文件的通讯录管理系统的示例代码:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LENGTH 50
#define MAX_ADDRESS_LENGTH 100
#define MAX_PHONE_LENGTH 20
typedef struct {
char name[MAX_NAME_LENGTH];
char sex;
int age;
char phone[MAX_PHONE_LENGTH];
char address[MAX_ADDRESS_LENGTH];
} Contact;
void add_contact(FILE* file) {
Contact contact;
printf("Enter name: ");
scanf("%s", contact.name);
printf("Enter sex (M/F): ");
scanf(" %c", &contact.sex);
printf("Enter age: ");
scanf("%d", &contact.age);
printf("Enter phone number: ");
scanf("%s", contact.phone);
printf("Enter address: ");
scanf("%s", contact.address);
fwrite(&contact, sizeof(Contact), 1, file);
printf("Contact added.\n");
}
void find_contact(FILE* file) {
char name[MAX_NAME_LENGTH];
Contact contact;
printf("Enter name to search: ");
scanf("%s", name);
rewind(file);
while (fread(&contact, sizeof(Contact), 1, file) == 1) {
if (strcmp(contact.name, name) == 0) {
printf("Name: %s\nSex: %c\nAge: %d\nPhone: %s\nAddress: %s\n", contact.name, contact.sex, contact.age, contact.phone, contact.address);
return;
}
}
printf("Contact not found.\n");
}
void display_contacts(FILE* file) {
Contact contact;
rewind(file);
while (fread(&contact, sizeof(Contact), 1, file) == 1) {
printf("Name: %s\nSex: %c\nAge: %d\nPhone: %s\nAddress: %s\n", contact.name, contact.sex, contact.age, contact.phone, contact.address);
}
}
void delete_contact(FILE* file) {
char name[MAX_NAME_LENGTH];
Contact contact;
FILE* temp_file = fopen("temp.bin", "wb");
printf("Enter name to delete: ");
scanf("%s", name);
rewind(file);
while (fread(&contact, sizeof(Contact), 1, file) == 1) {
if (strcmp(contact.name, name) != 0) {
fwrite(&contact, sizeof(Contact), 1, temp_file);
}
}
fclose(file);
fclose(temp_file);
remove("contacts.bin");
rename("temp.bin", "contacts.bin");
file = fopen("contacts.bin", "ab");
printf("Contact deleted.\n");
}
void modify_contact(FILE* file) {
char name[MAX_NAME_LENGTH];
Contact contact;
FILE* temp_file = fopen("temp.bin", "wb");
printf("Enter name to modify: ");
scanf("%s", name);
rewind(file);
while (fread(&contact, sizeof(Contact), 1, file) == 1) {
if (strcmp(contact.name, name) == 0) {
printf("Enter new name (leave blank to keep the same): ");
scanf("%s", contact.name);
printf("Enter new sex (leave blank to keep the same): ");
scanf(" %c", &contact.sex);
printf("Enter new age (leave blank to keep the same): ");
scanf("%d", &contact.age);
printf("Enter new phone number (leave blank to keep the same): ");
scanf("%s", contact.phone);
printf("Enter new address (leave blank to keep the same): ");
scanf("%s", contact.address);
}
fwrite(&contact, sizeof(Contact), 1, temp_file);
}
fclose(file);
fclose(temp_file);
remove("contacts.bin");
rename("temp.bin", "contacts.bin");
file = fopen("contacts.bin", "ab");
printf("Contact modified.\n");
}
int main() {
FILE* file = fopen("contacts.bin", "rb+");
if (file == NULL) {
file = fopen("contacts.bin", "wb+");
}
int choice;
do {
printf("1. Add contact\n2. Find contact\n3. Display contacts\n4. Delete contact\n5. Modify contact\n6. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
add_contact(file);
break;
case 2:
find_contact(file);
break;
case 3:
display_contacts(file);
break;
case 4:
delete_contact(file);
break;
case 5:
modify_contact(file);
break;
case 6:
break;
default:
printf("Invalid choice.\n");
}
} while (choice != 6);
fclose(file);
return 0;
}
该程序使用了一个Contact结构体来存储每个通讯录条目的信息,并使用了文件读写操作来实现增加、查找、显示、删除和修改通讯录条目的功能。在程序运行时,它会提示用户输入一个选项来执行相应的操作,直到用户选择退出为止。
阅读全文
相关推荐
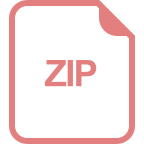
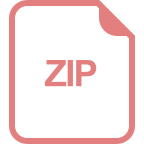

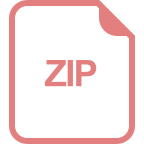
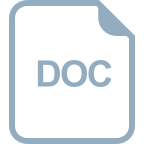
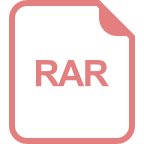
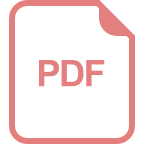
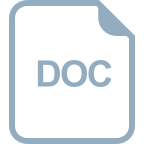
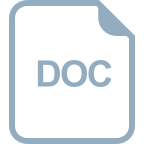
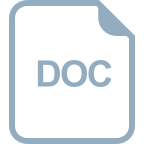
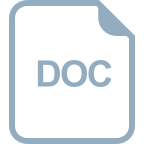
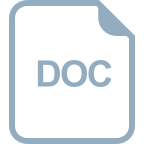
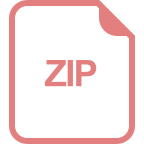
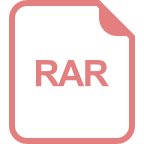
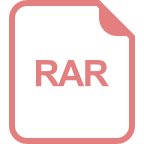
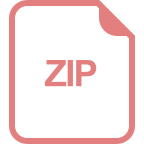