SystemProperties获取int类型的值
时间: 2024-03-07 16:46:30 浏览: 22
在安卓中,可以使用SystemProperties类获取系统属性的值,包括int类型的值。可以使用以下代码获取int类型的属性值:
```java
int value = SystemProperties.getInt("my_prop", 0); // 获取名为my_prop的属性值,如果不存在则返回0
```
其中,参数1为要获取的属性名称,参数2为如果属性不存在时的默认值。需要注意的是,SystemProperties是一个隐藏的API,需要在代码中使用反射来调用。具体的实现方法如下:
```java
try {
Class<?> clazz = Class.forName("android.os.SystemProperties");
Method method = clazz.getDeclaredMethod("getInt", String.class, int.class);
int value = (int) method.invoke(null, "my_prop", 0);
} catch (Exception e) {
e.printStackTrace();
}
```
需要注意的是,使用反射调用隐藏API可能会产生一些不可预见的问题,因此在使用时需要谨慎。
相关问题
kotlin创建springboot 配置properties类,包含int类型
可以按照以下步骤创建一个包含int类型的properties类:
1. 在src/main/resources目录下创建一个名为application.properties的文件。
2. 在该文件中添加以下内容:
```
myapp.port=8080
```
其中myapp.port表示属性名,8080表示属性值,可以根据需要修改。
3. 创建一个名为MyAppProperties的类,用于读取application.properties中的属性值。该类代码如下:
```
@ConfigurationProperties(prefix = "myapp")
@Component
public class MyAppProperties {
private int port;
public int getPort() {
return port;
}
public void setPort(int port) {
this.port = port;
}
}
```
该类使用@ConfigurationProperties注解指定属性前缀为myapp,然后定义了一个名为port的属性,类型为int。getPort和setPort方法用于访问该属性。
4. 在入口类中注入MyAppProperties类,以获取属性值。代码如下:
```
@SpringBootApplication
public class MyApp {
@Autowired
private MyAppProperties myAppProperties;
public static void main(String[] args) {
SpringApplication.run(MyApp.class, args);
}
@Bean
public CommandLineRunner commandLineRunner(ApplicationContext ctx) {
return args -> {
int port = myAppProperties.getPort();
System.out.println("Port: " + port);
};
}
}
```
该类使用@Autowired注解注入MyAppProperties类,然后在commandLineRunner方法中获取port属性的值,并输出到控制台。
5. 运行应用程序,可以看到输出结果为:Port: 8080。
以上就是创建一个包含int类型的properties类的步骤,通过这种方式可以方便地管理应用程序的配置信息。
C#判断所有值类型PropertyType
您可以使用反射来获取一个类型的所有属性,并且判断每个属性的类型是否为值类型。以下是一个示例代码:
```csharp
using System;
using System.Reflection;
class MyClass
{
public int MyInt { get; set; }
public float MyFloat { get; set; }
public string MyString { get; set; }
}
class Program
{
static void Main(string[] args)
{
Type type = typeof(MyClass);
PropertyInfo[] properties = type.GetProperties();
foreach (PropertyInfo property in properties)
{
Type propertyType = property.PropertyType;
if (propertyType.IsValueType)
{
Console.WriteLine(property.Name + " is a value type.");
}
else
{
Console.WriteLine(property.Name + " is not a value type.");
}
}
}
}
```
输出结果:
```
MyInt is a value type.
MyFloat is a value type.
MyString is not a value type.
```
相关推荐
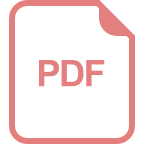
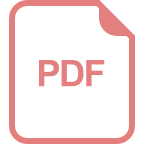
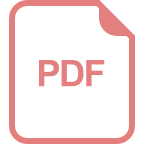







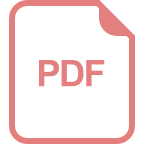
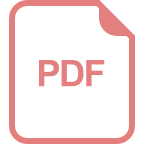
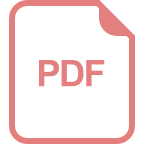
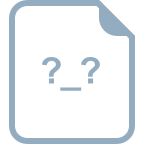