想用Python写一个脚本,根据需求ID,自动标记对应的单元格 单元格有单条需求ID时,一切正常,但是通过一条ID,匹配单元格内有多条需求ID时,无法匹配到
时间: 2023-02-07 16:58:14 浏览: 48
在这种情况下,你可以使用 Python 的正则表达式模块 re 来匹配多个需求 ID。
例如,假设你有一个单元格,内容如下:
cell = "需求ID: 123, 456, 789"
你可以使用如下代码来匹配所有需求 ID:
import re
# 匹配所有数字,并将它们保存到一个列表中
ids = re.findall(r'\d+', cell)
# 输出 ['123', '456', '789']
print(ids)
然后,你就可以遍历列表中的每个 ID,并根据需要对单元格进行标记。
如果你不熟悉正则表达式,你可以先学习一下,这会对你的脚本编写有很大帮助。
相关问题
写一个python脚本,将含有单元格填充颜色等格式的excel表格,合成一个表
好的,以下是一个Python脚本示例,可以将多个Excel表格合并为一个表格,并保留单元格填充颜色等格式:
```python
import openpyxl
from openpyxl.utils import get_column_letter
# 读取所有要合并的表格,将它们存储在一个列表中
workbooks = ['workbook1.xlsx', 'workbook2.xlsx', 'workbook3.xlsx']
sheets = []
for workbook in workbooks:
wb = openpyxl.load_workbook(workbook)
for sheet_name in wb.sheetnames:
sheet = wb[sheet_name]
sheets.append(sheet)
# 创建一个新的工作簿,并复制第一个表格到其中
merged_workbook = openpyxl.Workbook()
merged_sheet = merged_workbook.active
merged_sheet.title = sheets[0].title
for row in sheets[0].rows:
for cell in row:
merged_sheet[cell.coordinate].value = cell.value
merged_sheet[cell.coordinate].fill = cell.fill
merged_sheet[cell.coordinate].font = cell.font
# 将其他表格的内容复制到新工作簿中
for sheet in sheets[1:]:
new_sheet = merged_workbook.create_sheet(title=sheet.title)
for row in sheet.rows:
for cell in row:
new_sheet[cell.coordinate].value = cell.value
new_sheet[cell.coordinate].fill = cell.fill
new_sheet[cell.coordinate].font = cell.font
# 保存新工作簿
merged_workbook.save('merged_workbook.xlsx')
```
这个脚本做的事情是:
1. 读取所有要合并的Excel表格,并将它们存储在一个列表中。
2. 创建一个新的工作簿,并将第一个表格复制到其中。
3. 遍历其他表格的每个单元格,并将它们复制到新工作簿中。
4. 保存新工作簿。
在复制单元格时,我们还使用了 `cell.fill` 和 `cell.font` 属性来保留单元格填充颜色和字体等格式。
python面向对象写一个合并相同单元格,并输出新excel的脚本
好的,这是一个简单的示例代码,用于合并指定单元格并输出到新的 Excel 文件中:
```python
import openpyxl
class ExcelMerger:
def __init__(self, file_path):
self.file_path = file_path
self.workbook = openpyxl.load_workbook(file_path)
self.sheet = self.workbook.active
def merge_cells(self, start_row, start_col, end_row, end_col):
self.sheet.merge_cells(start_row=start_row, start_column=start_col, end_row=end_row, end_column=end_col)
def save_excel(self, new_file_path):
self.workbook.save(new_file_path)
if __name__ == '__main__':
merger = ExcelMerger('example.xlsx')
merger.merge_cells(1, 1, 3, 3)
merger.save_excel('output.xlsx')
```
在这个示例代码中,我们定义了一个名为 `ExcelMerger` 的类,包含了三个方法:
- `__init__(self, file_path)`:初始化方法,接收 Excel 文件的路径,并使用 `openpyxl` 库加载 Excel 文件。
- `merge_cells(self, start_row, start_col, end_row, end_col)`:用于合并指定范围内的单元格。
- `save_excel(self, new_file_path)`:用于保存合并后的 Excel 文件到新的文件中。
在 `__init__` 方法中,我们使用 `openpyxl` 库加载了指定的 Excel 文件,并将其保存到 `workbook` 和 `sheet` 变量中。在 `merge_cells` 方法中,我们使用 `sheet.merge_cells` 方法合并指定范围内的单元格。最后,在 `save_excel` 方法中,我们使用 `workbook.save` 方法将合并后的 Excel 文件保存到指定的路径中。
在 `if __name__ == '__main__':` 中,我们使用 `ExcelMerger` 类的实例对象 `merger` 来合并指定范围内的单元格,并将合并后的 Excel 文件保存到新的文件中。
你可以根据自己的需求修改这个示例代码,实现更加复杂的合并操作。
相关推荐
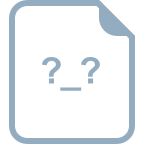
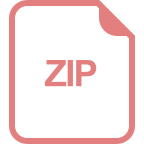
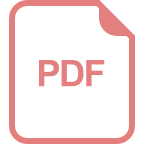












