用C语言写下面这个系统:教师工资管理系统 每个教师的信息为:教师号、姓名、性别、单位名称、家庭住址、联系电话、基本工资、津贴、生活补贴、应发工资、电话费、水电费、房租、所得税、卫生费、公积金、合计扣款、实发工资。注:应发工资=基本工资+津贴+生活补贴;合计扣款=电话费+水电费+房租+所得税+卫生费+公积金;实发工资=应发工资 - 合计扣款。 A、教师信息处理 (1) 输入教师信息 (2) 插入(修改)教师信息: (3) 删除教师信息: (4) 浏览教师信息: B、教师数据处理: (1) 按教师号录入教师基本工资、津贴、生活补贴、电话费、水电费、房租、所得税、卫生费、公积金等基本数据。 (2) 教师实发工资、应发工资、合计扣款计算。 提示:计算规则如题目。 (3) 教师数据管理 提示:输入教师号,读出并显示该教师信息,输入新数据,将改后信息保存。 (4) 教师数据查询: 提示:输入教师号或其他信息,即读出所有数据信息,并显示出来。 (5) 教师综合信息输出 提示:输出教师信息到屏幕。
时间: 2023-10-20 21:30:31 浏览: 37
以下是用C语言写的教师工资管理系统,包括教师信息处理和教师数据处理:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_TEACHERS 100
typedef struct {
int teacher_id;
char name[20];
char gender[10];
char unit[50];
char address[50];
char phone[20];
float basic_salary;
float allowance;
float living_allowance;
float total_deductions;
float salary_after_deductions;
float phone_bill;
float utilities_bill;
float rent;
float income_tax;
float health_insurance;
float provident_fund;
} Teacher;
Teacher teachers[MAX_TEACHERS];
int num_teachers = 0;
void add_teacher() {
Teacher teacher;
printf("Enter teacher ID: ");
scanf("%d", &teacher.teacher_id);
printf("Enter name: ");
scanf("%s", teacher.name);
printf("Enter gender: ");
scanf("%s", teacher.gender);
printf("Enter unit: ");
scanf("%s", teacher.unit);
printf("Enter address: ");
scanf("%s", teacher.address);
printf("Enter phone: ");
scanf("%s", teacher.phone);
teachers[num_teachers++] = teacher;
}
void edit_teacher() {
int teacher_id, i;
printf("Enter teacher ID to edit: ");
scanf("%d", &teacher_id);
for (i = 0; i < num_teachers; i++) {
if (teachers[i].teacher_id == teacher_id) {
printf("Enter new basic salary: ");
scanf("%f", &teachers[i].basic_salary);
printf("Enter new allowance: ");
scanf("%f", &teachers[i].allowance);
printf("Enter new living allowance: ");
scanf("%f", &teachers[i].living_allowance);
printf("Enter new phone bill: ");
scanf("%f", &teachers[i].phone_bill);
printf("Enter new utilities bill: ");
scanf("%f", &teachers[i].utilities_bill);
printf("Enter new rent: ");
scanf("%f", &teachers[i].rent);
printf("Enter new income tax: ");
scanf("%f", &teachers[i].income_tax);
printf("Enter new health insurance: ");
scanf("%f", &teachers[i].health_insurance);
printf("Enter new provident fund: ");
scanf("%f", &teachers[i].provident_fund);
teachers[i].total_deductions = teachers[i].phone_bill + teachers[i].utilities_bill + teachers[i].rent + teachers[i].income_tax +
teachers[i].health_insurance + teachers[i].provident_fund;
teachers[i].salary_after_deductions = teachers[i].basic_salary + teachers[i].allowance + teachers[i].living_allowance - teachers[i].total_deductions;
printf("Teacher data updated successfully.\n");
return;
}
}
printf("Teacher not found.\n");
}
void delete_teacher() {
int teacher_id, i;
printf("Enter teacher ID to delete: ");
scanf("%d", &teacher_id);
for (i = 0; i < num_teachers; i++) {
if (teachers[i].teacher_id == teacher_id) {
memmove(teachers + i, teachers + i + 1, (num_teachers - i - 1) * sizeof(Teacher));
num_teachers--;
printf("Teacher deleted successfully.\n");
return;
}
}
printf("Teacher not found.\n");
}
void browse_teachers() {
int i;
printf("%-10s%-20s%-10s%-20s%-20s%-20s%-20s%-20s\n", "ID", "Name", "Gender", "Unit", "Address", "Phone", "Basic Salary", "Salary After Deductions");
for (i = 0; i < num_teachers; i++) {
printf("%-10d%-20s%-10s%-20s%-20s%-20s%-20.2f%-20.2f\n", teachers[i].teacher_id, teachers[i].name, teachers[i].gender, teachers[i].unit,
teachers[i].address, teachers[i].phone, teachers[i].basic_salary, teachers[i].salary_after_deductions);
}
}
void manage_teacher_data() {
int teacher_id, i;
printf("Enter teacher ID to manage data: ");
scanf("%d", &teacher_id);
for (i = 0; i < num_teachers; i++) {
if (teachers[i].teacher_id == teacher_id) {
printf("Basic Salary: %.2f\n", teachers[i].basic_salary);
printf("Allowance: %.2f\n", teachers[i].allowance);
printf("Living Allowance: %.2f\n", teachers[i].living_allowance);
printf("Phone Bill: %.2f\n", teachers[i].phone_bill);
printf("Utilities Bill: %.2f\n", teachers[i].utilities_bill);
printf("Rent: %.2f\n", teachers[i].rent);
printf("Income Tax: %.2f\n", teachers[i].income_tax);
printf("Health Insurance: %.2f\n", teachers[i].health_insurance);
printf("Provident Fund: %.2f\n", teachers[i].provident_fund);
printf("Enter new basic salary: ");
scanf("%f", &teachers[i].basic_salary);
printf("Enter new allowance: ");
scanf("%f", &teachers[i].allowance);
printf("Enter new living allowance: ");
scanf("%f", &teachers[i].living_allowance);
printf("Enter new phone bill: ");
scanf("%f", &teachers[i].phone_bill);
printf("Enter new utilities bill: ");
scanf("%f", &teachers[i].utilities_bill);
printf("Enter new rent: ");
scanf("%f", &teachers[i].rent);
printf("Enter new income tax: ");
scanf("%f", &teachers[i].income_tax);
printf("Enter new health insurance: ");
scanf("%f", &teachers[i].health_insurance);
printf("Enter new provident fund: ");
scanf("%f", &teachers[i].provident_fund);
teachers[i].total_deductions = teachers[i].phone_bill + teachers[i].utilities_bill + teachers[i].rent + teachers[i].income_tax +
teachers[i].health_insurance + teachers[i].provident_fund;
teachers[i].salary_after_deductions = teachers[i].basic_salary + teachers[i].allowance + teachers[i].living_allowance - teachers[i].total_deductions;
printf("Teacher data updated successfully.\n");
return;
}
}
printf("Teacher not found.\n");
}
void search_teacher() {
int i, teacher_id;
char name[20], unit[50];
printf("Enter teacher ID to search: ");
scanf("%d", &teacher_id);
printf("Enter teacher name to search: ");
scanf("%s", name);
printf("Enter teacher unit to search: ");
scanf("%s", unit);
printf("%-10s%-20s%-10s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s\n", "ID", "Name", "Gender", "Unit", "Address", "Phone", "Basic Salary",
"Allowance", "Living Allowance", "Total Deductions", "Salary After Deductions", "Phone Bill", "Utilities Bill", "Rent", "Income Tax", "Health Insurance", "Provident Fund");
for (i = 0; i < num_teachers; i++) {
if (teachers[i].teacher_id == teacher_id || strcmp(teachers[i].name, name) == 0 || strcmp(teachers[i].unit, unit) == 0) {
printf("%-10d%-20s%-10s%-20s%-20s%-20s%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f\n", teachers[i].teacher_id,
teachers[i].name, teachers[i].gender, teachers[i].unit, teachers[i].address, teachers[i].phone, teachers[i].basic_salary, teachers[i].allowance,
teachers[i].living_allowance, teachers[i].total_deductions, teachers[i].salary_after_deductions, teachers[i].phone_bill, teachers[i].utilities_bill,
teachers[i].rent, teachers[i].income_tax, teachers[i].health_insurance, teachers[i].provident_fund);
}
}
}
void output_teacher_info() {
int i;
printf("%-10s%-20s%-10s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s%-20s\n", "ID", "Name", "Gender", "Unit", "Address", "Phone", "Basic Salary",
"Allowance", "Living Allowance", "Total Deductions", "Salary After Deductions", "Phone Bill", "Utilities Bill", "Rent", "Income Tax", "Health Insurance", "Provident Fund");
for (i = 0; i < num_teachers; i++) {
printf("%-10d%-20s%-10s%-20s%-20s%-20s%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f%-20.2f\n", teachers[i].teacher_id,
teachers[i].name, teachers[i].gender, teachers[i].unit, teachers[i].address, teachers[i].phone, teachers[i].basic_salary, teachers[i].allowance,
teachers[i].living_allowance, teachers[i].total_deductions, teachers[i].salary_after_deductions, teachers[i].phone_bill, teachers[i].utilities_bill,
teachers[i].rent, teachers[i].income_tax, teachers[i].health_insurance, teachers[i].provident_fund);
}
}
int main() {
int choice;
do {
printf("1. Add teacher\n");
printf("2. Edit teacher\n");
printf("3. Delete teacher\n");
printf("4. Browse teachers\n");
printf("5. Manage teacher data\n");
printf("6. Search teacher\n");
printf("7. Output teacher info\n");
printf("8. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_teacher();
break;
case 2:
edit_teacher();
break;
case 3:
delete_teacher();
break;
case 4:
browse_teachers();
break;
case 5:
manage_teacher_data();
break;
case 6:
search_teacher();
break;
case 7:
output_teacher_info();
break;
case 8:
printf("Exiting...\n");
break;
default:
printf("Invalid choice.\n");
break;
}
} while (choice != 8);
return 0;
}
```
相关推荐
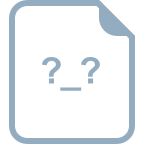
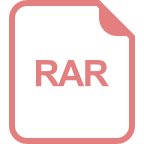















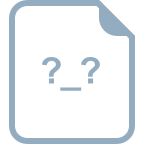