python的super函数
时间: 2023-06-26 10:06:38 浏览: 45
在Python中,`super()`函数是用来调用父类的方法。它可以用来在子类中调用父类的方法,避免在子类中重复编写相同的代码。
`super()`函数的一般用法是在子类的方法中调用父类的同名方法。例如:
```python
class Parent:
def hello(self):
print("Hello, Parent!")
class Child(Parent):
def hello(self):
super().hello() # 调用父类的hello方法
print("Hello, Child!")
c = Child()
c.hello()
```
输出结果为:
```
Hello, Parent!
Hello, Child!
```
在这个例子中,`Child`类继承了`Parent`类,并且重写了`hello()`方法。在`Child`类的`hello()`方法中,我们使用`super()`函数来调用父类的`hello()`方法,并在其后面添加自己的代码。
需要注意的是,`super()`函数的参数可以是当前子类的类名或者实例对象。如果省略参数,则默认为当前子类和当前实例对象。另外,`super()`函数只能用于新式类,不能用于旧式类。
相关问题
pythonsuper函数
super()函数是Python中的一个内置函数,它用于调用父类的方法。在面向对象编程中,如果子类和父类具有同名的方法,可以使用super()函数来调用父类的方法,以实现方法的继承和扩展。super()函数可以根据参数的不同,有不同的用法:
1. super():在子类中调用父类的方法时,可以使用super()函数来代替父类名字,这样可以避免直接使用父类名字造成的代码耦合性和维护困难。super()函数会自动找到子类的父类,并调用父类的方法。
2. super(type, obj):当父类不是直接的基类时,可以使用super(type, obj)来调用父类的方法。其中type是子类的类型,obj是子类的实例对象。
需要注意的是,super()函数必须在子类的方法中调用,并且在方法内部的第一行调用。
python super函数
在Python中,`super()`函数用于调用父类的方法。它可以在子类中调用父类的构造函数或其他方法。
使用`super()`函数的一般语法是:
```python
super().method_name(args)
```
其中,`method_name`是要调用的父类方法的名称,`args`是传递给父类方法的参数。
一般情况下,`super()`函数在子类的构造函数中使用,以确保子类能够继承父类的属性和方法。通过调用父类的构造函数,子类可以使用父类的属性和方法,并且可以添加或修改自己的属性和方法。
以下是一个示例,展示了如何在子类中使用`super()`函数调用父类的构造函数:
```python
class ParentClass:
def __init__(self, name):
self.name = name
class ChildClass(ParentClass):
def __init__(self, name, age):
super().__init__(name)
self.age = age
child = ChildClass("John", 10)
print(child.name) # 输出: John
print(child.age) # 输出: 10
```
在上面的例子中,`ChildClass`继承了`ParentClass`。通过使用`super().__init__(name)`,子类`ChildClass`调用了父类`ParentClass`的构造函数,并传递了一个参数`name`。这样,子类就可以使用父类的属性`name`,并且还可以添加自己的属性`age`。
希望这能帮到你!如果还有其他问题,请随时提问。
相关推荐
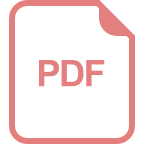
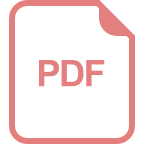
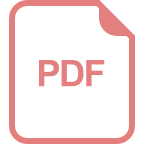












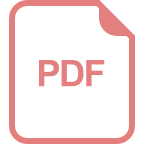