printinfo函数
时间: 2023-08-09 18:10:25 浏览: 654
printinfo函数是一个可变参数函数,它可以接受不同数量的参数。如果在函数调用时没有指定参数,它就是一个空元组。如果传递了多个参数,它们将被打印出来。[1]另外,如果在函数调用时使用了两个星号**,参数将以字典的形式导入。[2]这意味着可以通过键值对的方式传递参数。例如,printinfo(1, a=2, b=3)将打印出1和一个包含键值对a=2和b=3的字典。[2]此外,还可以定义一个名为printInfo的函数,它可以完成打印信息的功能。[3]
相关问题
C语言struct函数声明
在 C 语言中,可以使用结构体来定义一个自定义的数据类型,结构体中可以包含成员变量和成员函数。如果需要在结构体中声明函数,可以使用以下的语法格式:
```
struct student {
char name[20];
int age;
float score;
void (*printInfo)(struct student);
};
void print(struct student stu) {
printf("Name: %s\n", stu.name);
printf("Age: %d\n", stu.age);
printf("Score: %.2f\n", stu.score);
}
int main() {
struct student stu1 = {"Tom", 18, 90.5, print};
stu1.printInfo(stu1);
return 0;
}
```
上面的代码定义了一个名为 student 的结构体,其中包含一个成员函数 printInfo,该函数的类型为 void (*)(struct student),即一个返回值为 void,参数为 struct student 类型的函数指针。在 main 函数中,我们定义了一个名为 stu1 的 student 类型变量,并为其赋值,其中 printInfo 成员变量被赋值为 print 函数的地址。在 main 函数中,我们调用了 stu1.printInfo 函数,即调用了 print 函数,输出了 stu1 中的成员变量值。
需要注意的是,如果在结构体中声明的函数需要访问结构体成员变量,需要将结构体指针作为参数传递给函数,并在函数内部使用箭头操作符(->)来访问结构体成员变量。
定义一个基类Person,数据成员包含姓名,性别,年龄。成员构造函数用于初始化数据成员、成员函数Print()用于输出信息。从Person类公有派生出Student类,增加整型数据成员number用于保存学号,定义构造函数初始化数据成员、成员函数PrintInfo()用于输出学生信息。在主函数中完成测试。
好的,我了解您的需求,根据您的需求,我给您提供如下的代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Person {
public:
Person(string name, string gender, int age) : m_name(name), m_gender(gender), m_age(age) {}
void Print() const { cout << "姓名:" << m_name << ",性别:" << m_gender << ",年龄:" << m_age << endl; }
protected:
string m_name;
string m_gender;
int m_age;
};
class Student : public Person {
public:
Student(string name, string gender, int age, int number) : Person(name, gender, age), m_number(number) {}
void PrintInfo() const { cout << "学号:" << m_number << ","; Print(); }
private:
int m_number;
};
int main() {
Student s("张三", "男", 20, 1001);
s.PrintInfo();
return 0;
}
```
在上面的代码中,我们定义了一个名为Person的基类,该类包含姓名、性别和年龄三个数据成员,还包含一个构造函数用于初始化数据成员,以及一个Print函数用于输出信息。在构造函数中,我们初始化了姓名、性别和年龄的值。
接下来,我们从Person类公有派生出Student类,增加了一个整型数据成员number用于保存学号,并且定义了一个构造函数用于初始化数据成员,还定义了一个PrintInfo函数用于在控制台输出学生信息,其中调用了基类的Print函数进行信息的输出。
在主函数中,我们创建了一个Student对象,并调用其PrintInfo函数进行学生信息的输出。
希望这个代码能够帮助到您,如果您还有其他问题,请随时问我。
相关推荐
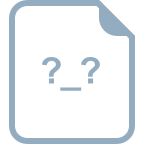
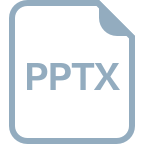
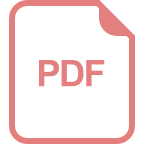













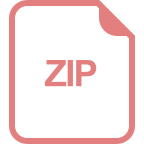