1. 声明并定义一个学生信息类StInfo,该类有四个数据成员和两个成员函数,其访问性都为公有。要求包括: (1)学号:int SID,姓名:char *Name,班级:char *Class,手机号:char *Phone; (2)给数据成员赋值的函数:void SetInfo(int sid,char *name,char* cla,char* phone),打印学生信息的函数:void PrintInfo( )。
时间: 2023-05-28 17:07:05 浏览: 370
class StInfo{
public:
int SID;
char *Name;
char *Class;
char *Phone;
void SetInfo(int sid, char *name, char *cla, char *phone){
SID = sid;
Name = name;
Class = cla;
Phone = phone;
}
void PrintInfo(){
cout<<"学号:"<<SID<<endl;
cout<<"姓名:"<<Name<<endl;
cout<<"班级:"<<Class<<endl;
cout<<"手机号:"<<Phone<<endl;
}
};
相关问题
在右侧编辑器中的Begin-End之间补充代码,声明并定义一个学生信息类 StInfo,该类有四个成员变量和两个函数,其访问性都为公有,具体要求如下
```cpp
class StInfo {
public:
// 成员变量
std::string name; // 姓名
int age; // 年龄
std::string gender; // 性别
float score; // 成绩
// 成员函数
void printInfo() {
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
std::cout << "Gender: " << gender << std::endl;
std::cout << "Score: " << score << std::endl;
}
void setInfo(const std::string& name_, int age_, const std::string& gender_, float score_) {
name = name_;
age = age_;
gender = gender_;
score = score_;
}
};
```
以上是一个简单的 StInfo 类,包含了姓名、年龄、性别、成绩等基本信息,以及获取和设置这些信息的成员函数。其中 `printInfo` 函数用于输出学生信息,而 `setInfo` 函数用于设置学生信息。
c++声明并定义一个学生信息类StInfo
在C++中,我们可以声明并定义一个名为`StInfo`的学生信息类,用于存储学生的姓名、年龄和学号等属性。下面是一个基本的示例:
```cpp
// 定义命名空间,通常放在项目的开头,这里为了简化例子省略了
// StInfo 是类名,首字母大写表示这是一个公共类
class StInfo {
private:
// 数据成员,代表学生属性
std::string name; // 学生姓名
int age; // 年龄
std::string id; // 学号
public:
// 构造函数,用于初始化类的实例
StInfo(const std::string& studentName, int studentAge, const std::string& studentID) :
name(studentName), age(studentAge), id(studentID) {}
// 获取属性的成员函数
std::string getName() const { return name; }
int getAge() const { return age; }
std::string getId() const { return id; }
// 可能需要添加设置属性值的赋值运算符和其它成员函数
void setName(std::string new_name) { name = new_name; }
void setAge(int new_age) { age = new_age; }
void setId(const std::string& new_id) { id = new_id; }
};
```
在这个类里,我们有私有数据成员来保存学生的个人信息,并提供公有的构造函数、getter和setter方法以便外部访问和修改这些信息。
阅读全文
相关推荐
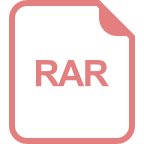
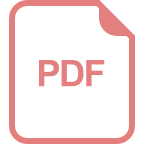








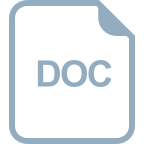
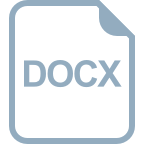
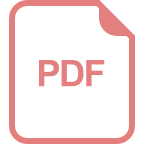
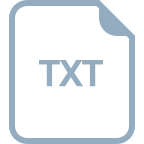
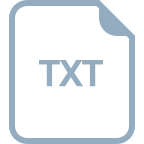
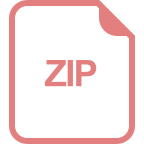