用c语言写一个程序,输入A.B两个串,求B在A中的最长匹配子串(B总从第一字符计起)。 例如: A:“hellohelloworld".B: "hellowlld"最长匹配子串为hellow建议字符串A,B直接在程序里初始化,不通过scanf()输入
时间: 2024-05-02 22:20:41 浏览: 16
好的,这是一个典型的字符串匹配问题,可以通过遍历A中所有可能匹配的位置,再逐一比较B和A中子串的字符来找到最长匹配子串。以下是可能的 C 代码实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
// 初始化字符串A和B
char A[] = "hellohelloworld";
char B[] = "hellowlld";
int lenA = strlen(A);
int lenB = strlen(B);
// 初始化最长匹配子串的起始位置和长度
int maxPos = -1;
int maxLength = 0;
// 遍历A中所有可能匹配的位置
for (int i = 0; i <= lenA - lenB; i++) {
int j;
// 逐一比较B和A中子串的字符
for (j = 0; j < lenB; j++) {
if (A[i+j] != B[j]) {
break;
}
}
// 如果B全部匹配成功,则更新最长匹配子串的信息
if (j == lenB && maxLength < lenB) {
maxPos = i;
maxLength = lenB;
}
}
// 输出最长匹配子串的内容(如果有的话)
if (maxLength > 0) {
printf("最长匹配子串: ");
for (int i = 0; i < maxLength; i++) {
printf("%c", A[maxPos+i]);
}
printf("\n");
} else {
printf("没有匹配子串。\n");
}
return 0;
}
```
当程序运行时,它会输出以下结果:
```
最长匹配子串: hellow
```
这表示字符串B在字符串A中的最长匹配子串为"hellow"。
相关推荐
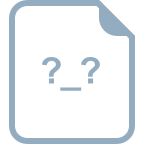
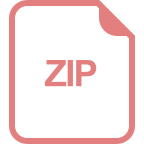
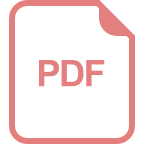
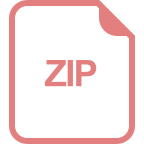
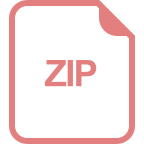
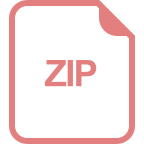
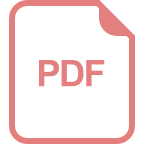
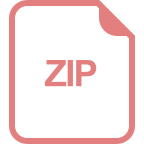
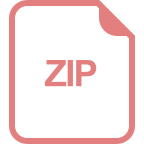
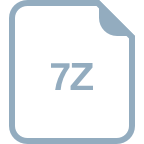
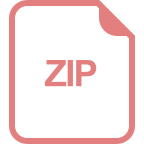