java sm2加密解密
时间: 2023-08-31 16:09:33 浏览: 152
Java 中可以使用 Bouncy Castle 库来实现 SM2 的加密和解密。以下是一个简单的示例代码:
```java
import org.bouncycastle.asn1.x9.X9ECParameters;
import org.bouncycastle.crypto.CipherParameters;
import org.bouncycastle.crypto.engines.SM2Engine;
import org.bouncycastle.crypto.params.ECPrivateKeyParameters;
import org.bouncycastle.crypto.params.ECPublicKeyParameters;
import org.bouncycastle.crypto.params.ParametersWithRandom;
import org.bouncycastle.crypto.util.PrivateKeyFactory;
import org.bouncycastle.crypto.util.PublicKeyFactory;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.bouncycastle.util.encoders.Hex;
import java.security.Security;
public class SM2Demo {
public static void main(String[] args) throws Exception {
// 添加 Bouncy Castle 作为安全提供程序
Security.addProvider(new BouncyCastleProvider());
// 生成随机密钥对
X9ECParameters ecParams = X9ECParameters.getInstance("sm2p256v1");
ECKeyPairGenerator keyPairGenerator = new ECKeyPairGenerator();
keyPairGenerator.init(new ECKeyGenerationParameters(ecParams, new SecureRandom()));
AsymmetricCipherKeyPair keyPair = keyPairGenerator.generateKeyPair();
// 转换为 Bouncy Castle 的密钥格式
ECPrivateKeyParameters privateKey = (ECPrivateKeyParameters) PrivateKeyFactory.createKey(keyPair.getPrivate().getEncoded());
ECPublicKeyParameters publicKey = (ECPublicKeyParameters) PublicKeyFactory.createKey(keyPair.getPublic().getEncoded());
// 明文数据
byte[] plaintext = "Hello, world!".getBytes(StandardCharsets.UTF_8);
// 加密
CipherParameters encryptParams = new ParametersWithRandom(publicKey, new SecureRandom());
SM2Engine encryptEngine = new SM2Engine();
encryptEngine.init(true, encryptParams);
byte[] ciphertext = encryptEngine.processBlock(plaintext, 0, plaintext.length);
// 输出密文
System.out.println("Ciphertext: " + Hex.toHexString(ciphertext));
// 解密
CipherParameters decryptParams = privateKey;
SM2Engine decryptEngine = new SM2Engine();
decryptEngine.init(false, decryptParams);
byte[] plaintext2 = decryptEngine.processBlock(ciphertext, 0, ciphertext.length);
// 输出明文
System.out.println("Plaintext: " + new String(plaintext2, StandardCharsets.UTF_8));
}
}
```
需要注意的是,Bouncy Castle 库可能需要单独引入,具体可以参考相关文档。此外,SM2 的密钥长度和加密算法都与传统的 RSA、AES 等算法有所不同,需要根据具体需求进行调整。
阅读全文
相关推荐
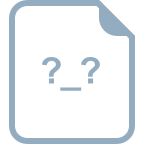

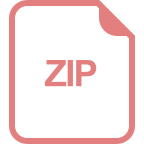
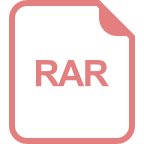
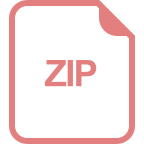









