java如何实现SM2加密解密
时间: 2024-01-19 10:02:32 浏览: 58
Java可以使用Bouncy Castle库来实现SM2加密解密。下面是一个示例代码:
```java
import org.bouncycastle.crypto.AsymmetricCipherKeyPair;
import org.bouncycastle.crypto.CipherParameters;
import org.bouncycastle.crypto.engines.SM2Engine;
import org.bouncycastle.crypto.generators.ECKeyPairGenerator;
import org.bouncycastle.crypto.params.ECKeyGenerationParameters;
import org.bouncycastle.crypto.params.ECPrivateKeyParameters;
import org.bouncycastle.crypto.params.ECPublicKeyParameters;
import org.bouncycastle.crypto.params.ParametersWithRandom;
import org.bouncycastle.crypto.util.PrivateKeyFactory;
import org.bouncycastle.crypto.util.PublicKeyFactory;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.bouncycastle.util.encoders.Hex;
import java.security.Security;
import java.security.SecureRandom;
public class SM2Example {
public static void main(String[] args) throws Exception {
Security.addProvider(new BouncyCastleProvider());
// 生成密钥对
ECKeyPairGenerator keyPairGenerator = new ECKeyPairGenerator();
ECKeyGenerationParameters keyGenerationParameters = new ECKeyGenerationParameters(SM2Util.DOMAIN_PARAMS, new SecureRandom());
keyPairGenerator.init(keyGenerationParameters);
AsymmetricCipherKeyPair keyPair = keyPairGenerator.generateKeyPair();
// 获取公钥和私钥
ECPublicKeyParameters publicKey = (ECPublicKeyParameters) keyPair.getPublic();
ECPrivateKeyParameters privateKey = (ECPrivateKeyParameters) keyPair.getPrivate();
// 加密
String plaintext = "Hello, SM2!";
byte[] plaintextBytes = plaintext.getBytes("UTF-8");
CipherParameters encryptParams = new ParametersWithRandom(publicKey, new SecureRandom());
SM2Engine encryptEngine = new SM2Engine();
encryptEngine.init(true, encryptParams);
byte[] ciphertextBytes = encryptEngine.processBlock(plaintextBytes, 0, plaintextBytes.length);
// 解密
CipherParameters decryptParams = new ParametersWithRandom(privateKey, new SecureRandom());
SM2Engine decryptEngine = new SM2Engine();
decryptEngine.init(false, decryptParams);
byte[] decryptedBytes = decryptEngine.processBlock(ciphertextBytes, 0, ciphertextBytes.length);
String decryptedText = new String(decryptedBytes, "UTF-8");
System.out.println("Plaintext: " + plaintext);
System.out.println("Ciphertext: " + Hex.toHexString(ciphertextBytes));
System.out.println("Decrypted text: " + decryptedText);
}
}
```
这段代码使用Bouncy Castle库生成SM2密钥对,然后使用公钥加密明文,私钥解密密文。注意,需要先导入Bouncy Castle库,并且确保密钥对的生成参数`SM2Util.DOMAIN_PARAMS`正确设置。
相关推荐
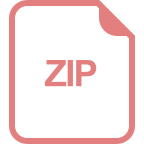
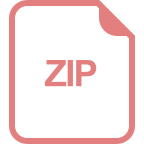
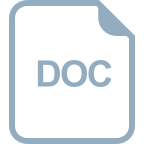
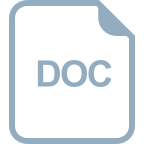
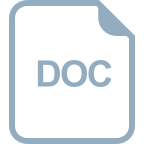
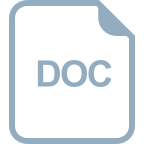
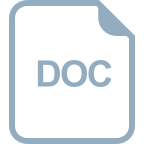









