首页
linux的select函数
linux的select函数
时间: 2023-05-01 10:06:41
浏览: 100
linux系统下select函数详解
5星 · 资源好评率100%
立即下载
select函数详解
Linux的select函数是用于多路复用I/O的系统调用函数。它可以对多个文件描述符进行监听,并在其中任意一个文件描述符变为“就绪状态”时返回该文件描述符,以便进行读写等操作。因此,select函数是一种高效的实现异步I/O的方法。
阅读全文
相关推荐
Linux中select函数使用详解.zip_Linux中select函数使用详解
在Linux系统中,select函数是用于处理多个文件描述符(FD)的I/O事件的一种方式,尤其在多路复用I/O编程中扮演着重要角色。它允许程序同时监控多个文件描述符,等待它们准备就绪后进行读写操作,而无需轮询检查每...
linux使用select实现精确定时器详解
首先看看select函数原型如下: 代码如下:int select(int nfds, fd_set *readfds, fd_set *writefds, fd_set *exceptfds, struct timeval *timeout);参数说明:slect的第一个参数nfds为fdset集合中最大描述符值加1,...
linux select函数
在 Linux 中,select 函数是一种 I/O 多路复用机制,用于同时监控多个文件描述符的读写状态。该函数的原型如下: #include <sys/select.h> int select(int nfds, fd_set *readfds, fd_set *writefds, fd_set *...
linuxselect函数
select() 是一个在 Linux 系统中提供的函数,用于检查多个文件描述符(sockets、标准输入输出、文件等)是否处于可读、可写或者异常等状态。 函数原型如下: c #include <sys/select.h> int select(int nfds...
linux select函数怎么用
在 Linux 中,select() 函数的原型是: #include <sys/select.h> int select(int nfds, fd_set *readfds, fd_set *writefds, fd_set *exceptfds, struct timeval *timeout); 其中,参数说明如下: - ...
linux 用户层的select函数
select函数是Linux用户层常用的函数之一,它可用于监视一组文件描述符,等待其中任意一个或多个文件描述符变为就绪状态(可读、可写或异常),从而进行相应的操作。 select函数的原型如下: c #include <sys/...
linux Cselect函数多路复用
select函数是Linux C编程中的一种多路复用机制,用于监视并等待多个文件描述符的属性发生变化。它可以同时监视多个文件描述符的可读、可写和异常状态。select函数的原型如下: c #include <sys/select.h> int ...
c++的select函数
在Linux下,select函数最多可以同时监听1024个文件描述符,而且每次调用select函数时,都需要重新设置待检测的文件描述符集合,因为select函数会修改文件描述符集合。另外,select函数的效率也比较低,因为它需要...
select函数的用法
在Linux环境下,select函数的用法如下: int select(int maxfd, fd_set *readset, fd_set *writeset, fd_set *exceptset, const struct timeval *timeout); 其中,maxfd是需要监视的最大文件描述符值加1;readset、...
linux select timeout
在 Linux 中,select 函数可以用于监视多个文件描述符的状态变化,从而实现 IO 多路复用。如果你想在 select 函数中设置一个超时时间,可以在 timeout 参数中指定一个非零值。timeout 参数的类型是 struct timeval,...
linux poll函数
Linux poll函数是一种用于I/O多路复用的系统调用,它可以同时监视多个文件描述符,等待其中任意一个文件描述符上的事件...与select函数相比,poll函数支持的文件描述符数量更多,效率更高,但是使用起来稍微复杂一些。
linux select poll epoll
select, poll, 和 epoll 是 Linux 中用于多路复用 I/O 操作的机制。 select 是最古老且最简单的一种机制。它采用轮询的方式来监视一组文件描述符的状态,当其中任何一个文件描述符就绪时,select 函数将返回,并且...
linux驱动select末班
select函数是Linux内核提供的一种多路复用I/O的机制,可以同时监视多个文件描述符的状态,当其中任意一个文件描述符发生变化时,select函数就会返回。在驱动中使用select函数可以实现异步通信,提高系统的并发性能。...
select Linux
在Linux下,select函数是一种I/O复用技术,可以同时监视多个文件描述符,判断是否有符合条件的事件发生。通过使用select函数,我们可以查看是否有可读、可写或错误的事件发生。该函数的原型如下: #include ...
linux select使用实例
下面是一个简单的 Linux select 使用实例,用于监听标准输入和标准输出是否有数据可读写。 c #include #include #include <sys/select.h> #include int main() { fd_set rfds, wfds; // 用于存储文件描述...
str 和select函数引用
而select函数通常出现在网络编程或I/O多路复用上下文中,如在Unix/Linux系统编程的套接字编程中。select()函数允许程序监视多个文件描述符(如套接字),当某个描述符变得可读或可写时,该函数会通知进程。它是...
python中的select函数
select函数是Python中用于监听多个文件描述符(包括socket连接)的一种I/O复用机制。...需要注意的是,select函数在Windows系统上有一些限制,建议在Unix/Linux系统上使用更为强大的epoll或kqueue等机制来代替。
linux网络编程select
Linux网络编程中的select函数用于在一组文件描述符上进行多路复用,以便能够同时监视多个文件描述符是否有数据可读、可写或异常等事件发生。 select函数的原型如下: c #include <sys/select.h> int select(int...
linux中select
select函数的参数包括要监视的文件描述符集合、超时时间和监视事件类型等。当有文件描述符发生变化时,select函数会返回相应的文件描述符集合,程序可以根据返回的结果进行相应的处理。select函数常用于网络编程中,...
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
海量
VIP免费资源
千本
正版电子书
商城
会员专享价
千门
课程&专栏
全年可省5,000元
立即开通
全年可省5,000元
立即开通
最新推荐
linux使用select实现精确定时器详解
在Linux系统编程中,`select`函数是一种常用的同步I/O多路复用机制,它可以监控多个文件描述符,等待它们中的任意一个准备就绪。在本文中,我们将深入探讨如何利用`select`函数来实现精确定时器,尤其是微妙级别的...
linux内核select/poll,epoll实现与区别
Linux内核中的`select`、`poll`和`epoll`是用于实现多路复用I/O的关键函数,它们允许程序在一个线程中同时处理多个文件描述符的读写操作,提高了系统的并发处理能力。这些函数在不同场景下各有优劣,理解它们的实现...
linux下select和poll的用法
select 和 poll 函数都是 Linux 中常用的系统调用,它们用于查询设备是否可读写或是否处于某种状态。在设备驱动程序中,select 和 poll 函数可以用于查询设备的状态,从而实现设备的异步输入/输出操作。
linux下的高并发处理select 和epoll
在Linux系统中,处理高并发I/O事件时,select和epoll是两种常见的技术。本文将详细介绍这两种技术,以及它们在处理大量并发连接时的特点和优势。 首先,我们来看看`select`函数。`select`是一种古老的I/O多路复用...
藏区特产销售平台--论文.zip
藏区特产销售平台--论文.zip
Angular程序高效加载与展示海量Excel数据技巧
资源摘要信息: "本文将讨论如何在Angular项目中加载和显示Excel海量数据,具体包括使用xlsx.js库读取Excel文件以及采用批量展示方法来处理大量数据。为了更好地理解本文内容,建议参阅关联介绍文章,以获取更多背景信息和详细步骤。" 知识点: 1. Angular框架: Angular是一个由谷歌开发和维护的开源前端框架,它使用TypeScript语言编写,适用于构建动态Web应用。在处理复杂单页面应用(SPA)时,Angular通过其依赖注入、组件和服务的概念提供了一种模块化的方式来组织代码。 2. Excel文件处理: 在Web应用中处理Excel文件通常需要借助第三方库来实现,比如本文提到的xlsx.js库。xlsx.js是一个纯JavaScript编写的库,能够读取和写入Excel文件(包括.xlsx和.xls格式),非常适合在前端应用中处理Excel数据。 3. xlsx.core.min.js: 这是xlsx.js库的一个缩小版本,主要用于生产环境。它包含了读取Excel文件核心功能,适合在对性能和文件大小有要求的项目中使用。通过使用这个库,开发者可以在客户端对Excel文件进行解析并以数据格式暴露给Angular应用。 4. 海量数据展示: 当处理成千上万条数据记录时,传统的方式可能会导致性能问题,比如页面卡顿或加载缓慢。因此,需要采用特定的技术来优化数据展示,例如虚拟滚动(virtual scrolling),分页(pagination)或懒加载(lazy loading)等。 5. 批量展示方法: 为了高效显示海量数据,本文提到的批量展示方法可能涉及将数据分组或分批次加载到视图中。这样可以减少一次性渲染的数据量,从而提升应用的响应速度和用户体验。在Angular中,可以利用指令(directives)和管道(pipes)来实现数据的分批处理和显示。 6. 关联介绍文章: 提供的文章链接为读者提供了更深入的理解和实操步骤。这可能是关于如何配置xlsx.js在Angular项目中使用、如何读取Excel文件中的数据、如何优化和展示这些数据的详细指南。读者应根据该文章所提供的知识和示例代码,来实现上述功能。 7. 文件名称列表: "excel"这一词汇表明,压缩包可能包含一些与Excel文件处理相关的文件或示例代码。这可能包括与xlsx.js集成的Angular组件代码、服务代码或者用于展示数据的模板代码。在实际开发过程中,开发者需要将这些文件或代码片段正确地集成到自己的Angular项目中。 总结而言,本文将指导开发者如何在Angular项目中集成xlsx.js来处理Excel文件的读取,以及如何优化显示大量数据的技术。通过阅读关联介绍文章和实际操作示例代码,开发者可以掌握从后端加载数据、通过xlsx.js解析数据以及在前端高效展示数据的技术要点。这对于开发涉及复杂数据交互的Web应用尤为重要,特别是在需要处理大量数据时。
管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
【SecureCRT高亮技巧】:20年经验技术大佬的个性化设置指南
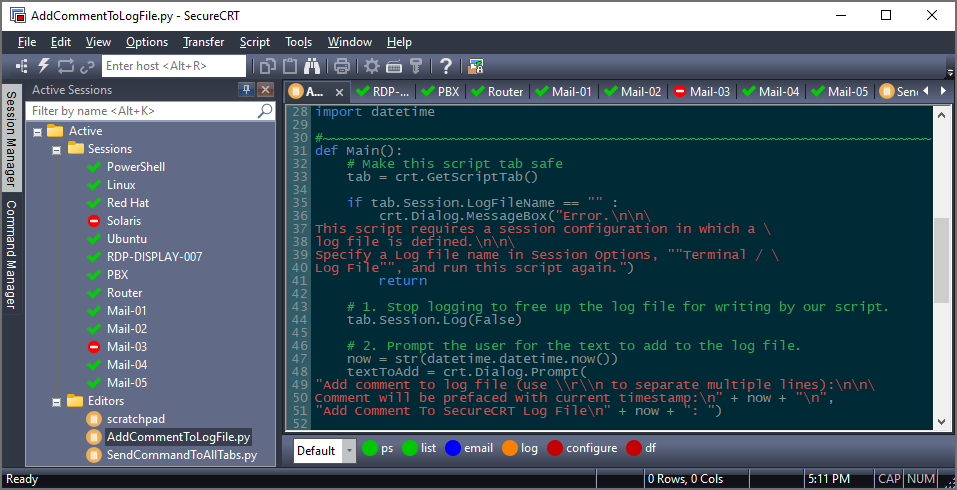 参考资源链接:[SecureCRT设置代码关键字高亮教程](https://wenku.csdn.net/doc/6412b5eabe7fbd1778d44db0?spm=1055.2635.3001.10343) # 1. SecureCRT简介与高亮功能概述 SecureCRT是一款广泛应用于IT行业的远程终端仿真程序,支持
如何设计一个基于FPGA的多功能数字钟,实现24小时计时、手动校时和定时闹钟功能?
设计一个基于FPGA的多功能数字钟涉及数字电路设计、时序控制和模块化编程。首先,你需要理解计时器、定时器和计数器的概念以及如何在FPGA平台上实现它们。《大连理工数字钟设计:模24计时器与闹钟功能》这份资料详细介绍了实验报告的撰写过程,包括设计思路和实现方法,对于理解如何构建数字钟的各个部分将有很大帮助。 参考资源链接:[大连理工数字钟设计:模24计时器与闹钟功能](https://wenku.csdn.net/doc/5y7s3r19rz?spm=1055.2569.3001.10343) 在硬件设计方面,你需要准备FPGA开发板、时钟信号源、数码管显示器、手动校时按钮以及定时闹钟按钮等
Argos客户端开发流程及Vue配置指南
资源摘要信息:"argos-client:客户端" 1. Vue项目基础操作 在"argos-client:客户端"项目中,首先需要进行项目设置,通过运行"yarn install"命令来安装项目所需的依赖。"yarn"是一个流行的JavaScript包管理工具,它能够管理项目的依赖关系,并将它们存储在"package.json"文件中。 2. 开发环境下的编译和热重装 在开发阶段,为了实时查看代码更改后的效果,可以使用"yarn serve"命令来编译项目并开启热重装功能。热重装(HMR, Hot Module Replacement)是指在应用运行时,替换、添加或删除模块,而无需完全重新加载页面。 3. 生产环境的编译和最小化 项目开发完成后,需要将项目代码编译并打包成可在生产环境中部署的版本。运行"yarn build"命令可以将源代码编译为最小化的静态文件,这些文件通常包含在"dist/"目录下,可以部署到服务器上。 4. 单元测试和端到端测试 为了确保项目的质量和可靠性,单元测试和端到端测试是必不可少的。"yarn test:unit"用于运行单元测试,这是测试单个组件或函数的测试方法。"yarn test:e2e"用于运行端到端测试,这是模拟用户操作流程,确保应用程序的各个部分能够协同工作。 5. 代码规范与自动化修复 "yarn lint"命令用于代码的检查和风格修复。它通过运行ESLint等代码风格检查工具,帮助开发者遵守预定义的编码规范,从而保持代码风格的一致性。此外,它也能自动修复一些可修复的问题。 6. 自定义配置与Vue框架 由于"argos-client:客户端"项目中提到的Vue标签,可以推断该项目使用了Vue.js框架。Vue是一个用于构建用户界面的渐进式JavaScript框架,它允许开发者通过组件化的方式构建复杂的单页应用程序。在项目的自定义配置中,可能需要根据项目需求进行路由配置、状态管理(如Vuex)、以及与后端API的集成等。 7. 压缩包子文件的使用场景 "argos-client-master"作为压缩包子文件的名称,表明该项目可能还涉及打包发布或模块化开发。在项目开发中,压缩包子文件通常用于快速分发和部署代码,或者是在模块化开发中作为依赖进行引用。使用压缩包子文件可以确保项目的依赖关系清晰,并且方便其他开发者快速安装和使用。 通过上述内容的阐述,我们可以了解到在进行"argos-client:客户端"项目的开发时,需要熟悉的一系列操作,包括项目设置、编译和热重装、生产环境编译、单元测试和端到端测试、代码风格检查和修复,以及与Vue框架相关的各种配置。同时,了解压缩包子文件在项目中的作用,能够帮助开发者高效地管理和部署代码。