linux select使用实例
时间: 2023-08-19 22:05:08 浏览: 111
下面是一个简单的 Linux `select` 使用实例,用于监听标准输入和标准输出是否有数据可读写。
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <unistd.h>
int main() {
fd_set rfds, wfds; // 用于存储文件描述符集合的 fd_set 结构体
int maxfd = STDIN_FILENO + 1; // 待监视的最大文件描述符值加一
struct timeval timeout = {5, 0}; // 超时时间为 5 秒
while (1) {
FD_ZERO(&rfds); // 将读文件描述符集合清零
FD_ZERO(&wfds); // 将写文件描述符集合清零
FD_SET(STDIN_FILENO, &rfds); // 将标准输入加入到读文件描述符集合
FD_SET(STDOUT_FILENO, &wfds); // 将标准输出加入到写文件描述符集合
// 调用 select 函数进行 I/O 多路复用
int ret = select(maxfd, &rfds, &wfds, NULL, &timeout);
if (ret < 0) {
perror("select error");
exit(1);
} else if (ret == 0) {
printf("timeout\n");
} else {
// 判断标准输入是否可读
if (FD_ISSET(STDIN_FILENO, &rfds)) {
char buf[1024];
int n = read(STDIN_FILENO, buf, sizeof(buf));
if (n < 0) {
perror("read error");
exit(1);
} else if (n == 0) {
printf("EOF\n");
break;
} else {
printf("read: %s", buf);
}
}
// 判断标准输出是否可写
if (FD_ISSET(STDOUT_FILENO, &wfds)) {
char buf[1024] = "hello world\n";
int n = write(STDOUT_FILENO, buf, sizeof(buf));
if (n < 0) {
perror("write error");
exit(1);
} else {
printf("write: %s", buf);
}
}
}
}
return 0;
}
```
该程序通过 `FD_SET` 函数将标准输入和标准输出加入到读和写文件描述符集合中,然后调用 `select` 函数进行 I/O 多路复用。当标准输入可读或标准输出可写时,`select` 函数会返回,程序通过 `FD_ISSET` 函数判断哪个文件描述符可读或可写,并进行相应的读写操作。
阅读全文
相关推荐
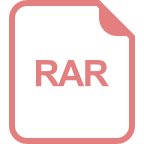
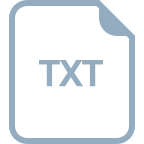
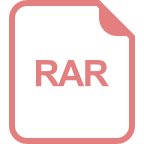
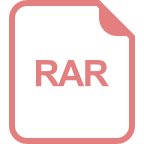
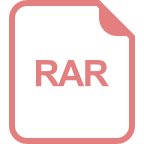
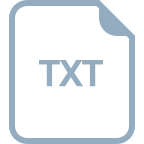
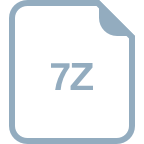
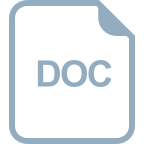
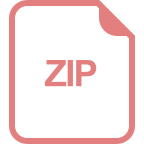
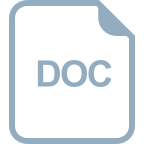
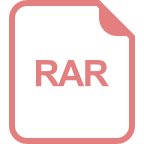
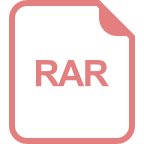
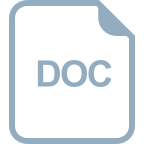


