list集合根据对象属性去重的多种方法实现
时间: 2023-11-16 14:07:07 浏览: 49
有多种方法可以实现List集合根据对象属性去重,以下是其中几种常用的方法:
1. 重写对象的equals和hashCode方法
重写对象的equals和hashCode方法,根据属性判断两个对象是否相等,然后将对象放入Set集合中去重。示例代码如下:
```java
public class Person {
private String name;
private int age;
// 省略构造方法和getter/setter方法
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return age == person.age &&
Objects.equals(name, person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
List<Person> personList = new ArrayList<>();
personList.add(new Person("张三", 20));
personList.add(new Person("李四", 22));
personList.add(new Person("张三", 20));
Set<Person> personSet = new HashSet<>(personList);
System.out.println(personSet);
```
输出结果为:`[Person{name='张三', age=20}, Person{name='李四', age=22}]`
2. 使用Java 8的stream和distinct方法
使用Java 8的stream和distinct方法,根据属性去重。示例代码如下:
```java
List<Person> personList = new ArrayList<>();
personList.add(new Person("张三", 20));
personList.add(new Person("李四", 22));
personList.add(new Person("张三", 20));
List<Person> distinctPersonList = personList.stream().distinct().collect(Collectors.toList());
System.out.println(distinctPersonList);
```
输出结果为:`[Person{name='张三', age=20}, Person{name='李四', age=22}]`
3. 使用Java 8的stream和toMap方法
使用Java 8的stream和toMap方法,根据属性去重。示例代码如下:
```java
List<Person> personList = new ArrayList<>();
personList.add(new Person("张三", 20));
personList.add(new Person("李四", 22));
personList.add(new Person("张三", 20));
List<Person> distinctPersonList = personList.stream().collect(Collectors.toMap(
person -> person.getName() + "-" + person.getAge(),
person -> person,
(oldValue, newValue) -> oldValue)).values().stream().collect(Collectors.toList());
System.out.println(distinctPersonList);
```
输出结果为:`[Person{name='张三', age=20}, Person{name='李四', age=22}]`
以上是几种常用的List集合根据对象属性去重的方法,可以根据实际场景选择合适的方法。
相关推荐
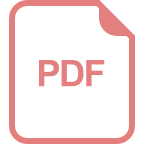
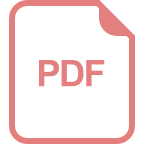














