你可以写一段两阶段多路归并排序的算法吗
时间: 2024-01-23 18:02:26 浏览: 199
当然可以,以下是一段基于两阶段多路归并排序的伪代码:
```
// 定义两个数组作为输入数据
int[] array1 = { 1, 3, 5, 7 };
int[] array2 = { 2, 4, 6, 8 };
// 定义一个空数组作为输出数据
int[] result = new int[array1.length + array2.length];
// 第一阶段:将输入数据分成若干组进行排序
int groupSize = 2; // 每组包含的元素个数
for (int i = 0; i < result.length; i += groupSize) {
// 对每组进行排序
int[] group = new int[groupSize];
int index1 = i;
int index2 = i + groupSize / 2;
for (int j = 0; j < groupSize; j++) {
if (index1 >= i + groupSize / 2) {
// 已经处理完第一个输入数组
group[j] = array2[index2++];
} else if (index2 >= i + groupSize) {
// 已经处理完第二个输入数组
group[j] = array1[index1++];
} else if (array1[index1] < array2[index2]) {
// 第一个输入数组的当前元素小于第二个输入数组的当前元素
group[j] = array1[index1++];
} else {
// 第二个输入数组的当前元素小于第一个输入数组的当前元素
group[j] = array2[index2++];
}
}
// 将排序后的结果存入输出数组
System.arraycopy(group, 0, result, i, groupSize);
}
// 第二阶段:将排好序的若干组进行归并排序
int groupSize = 2; // 每组包含的元素个数
while (groupSize < result.length) {
for (int i = 0; i < result.length; i += groupSize * 2) {
// 对每两组进行归并排序
int[] group1 = new int[groupSize];
int[] group2 = new int[groupSize];
System.arraycopy(result, i, group1, 0, groupSize);
System.arraycopy(result, i + groupSize, group2, 0, groupSize);
int index1 = 0;
int index2 = 0;
for (int j = i; j < i + groupSize * 2; j++) {
if (index1 >= groupSize) {
// 已经处理完第一个数组
result[j] = group2[index2++];
} else if (index2 >= groupSize) {
// 已经处理完第二个数组
result[j] = group1[index1++];
} else if (group1[index1] < group2[index2]) {
// 第一个数组的当前元素小于第二个数组的当前元素
result[j] = group1[index1++];
} else {
// 第二个数组的当前元素小于第一个数组的当前元素
result[j] = group2[index2++];
}
}
}
// 继续对更大的组进行归并排序
groupSize *= 2;
}
```
注意,以上代码仅为伪代码,需要根据具体的编程语言进行实现。此外,该算法的时间复杂度为 O(n log n),其中 n 表示输入数据的大小。
阅读全文
相关推荐
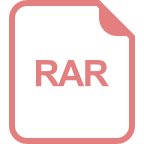
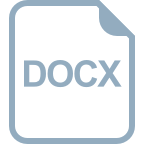
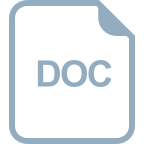
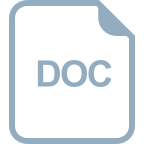
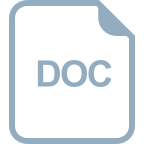


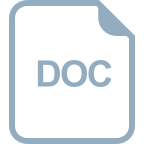
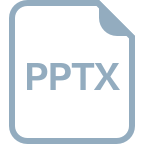
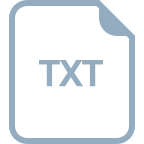
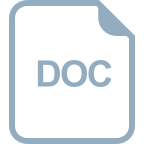
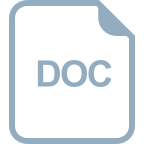
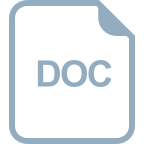
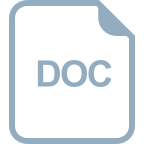
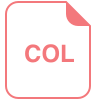
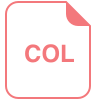