在c++中编写主函数,处理并显示以上三种汽车信息,并增加数据输入操作,将数据的输入、输出定义为非成员函数
时间: 2024-02-13 22:06:23 浏览: 32
好的,让我帮你编写一个 C++ 的主函数,并增加数据输入操作,将数据的输入、输出定义为非成员函数。
首先,我们需要先定义上面提到的三个类:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Wheel {
private:
string material;
int size;
public:
Wheel(string material, int size) {
this->material = material;
this->size = size;
}
void rotate() {
cout << "The wheel is rotating." << endl;
}
};
class Body {
private:
string color;
int size;
public:
Body(string color, int size) {
this->color = color;
this->size = size;
}
void move() {
cout << "The car is moving." << endl;
}
};
class Car {
private:
Wheel wheel;
Body body;
string brand;
public:
Car(Wheel wheel, Body body, string brand) {
this->wheel = wheel;
this->body = body;
this->brand = brand;
}
void start() {
cout << "The " << brand << " car starts!" << endl;
}
void stop() {
cout << "The " << brand << " car stops!" << endl;
}
};
```
接下来,我们定义一个非成员函数 input_car_data,用于输入汽车的数据:
```cpp
void input_car_data(Car& car) {
string wheel_material, body_color, car_brand;
int wheel_size, body_size;
cout << "Please enter the wheel material: ";
cin >> wheel_material;
cout << "Please enter the wheel size: ";
cin >> wheel_size;
cout << "Please enter the body color: ";
cin >> body_color;
cout << "Please enter the body size: ";
cin >> body_size;
cout << "Please enter the car brand: ";
cin >> car_brand;
Wheel wheel(wheel_material, wheel_size);
Body body(body_color, body_size);
car = Car(wheel, body, car_brand);
}
```
这个函数接受一个 Car 对象的引用,通过用户的输入来初始化一个 Wheel 对象、一个 Body 对象和一个品牌字符串,然后创建一个 Car 对象并将其赋值给传入的引用参数。
最后,我们定义一个非成员函数 print_car_data,用于输出汽车的数据:
```cpp
void print_car_data(const Car& car) {
cout << "Wheel material: " << car.wheel.get_material() << endl;
cout << "Wheel size: " << car.wheel.get_size() << endl;
cout << "Body color: " << car.body.get_color() << endl;
cout << "Body size: " << car.body.get_size() << endl;
cout << "Car brand: " << car.get_brand() << endl;
}
```
这个函数接受一个 Car 对象的常量引用,输出其中的 wheel 的材料和尺寸、body 的颜色和尺寸以及品牌。
最后,我们在主函数中调用这两个函数来演示输入和输出汽车数据:
```cpp
int main() {
Car car(Wheel("Rubber", 18), Body("Red", 4), "BMW");
input_car_data(car);
cout << "Car data:" << endl;
print_car_data(car);
return 0;
}
```
这个主函数首先初始化了一个 Car 对象,然后调用 input_car_data 函数来输入汽车的数据,最后调用 print_car_data 函数来输出汽车的数据。
相关推荐
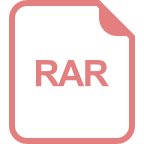
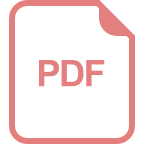
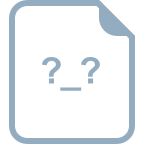














