将txt转换为xml标签python代码
时间: 2023-08-06 21:03:22 浏览: 84
以下是将txt转换为xml标签的Python代码:
```python
import xml.etree.ElementTree as ET
# 读取txt文件
with open('example.txt', 'r') as f:
data = f.readlines()
# 创建根节点
root = ET.Element('root')
# 循环读取每一行数据,创建子节点,并添加到根节点中
for line in data:
child = ET.SubElement(root, 'item')
child.text = line.strip()
# 将根节点转换为xml字符串,并保存到文件中
tree = ET.ElementTree(root)
tree.write('example.xml')
```
上述代码中,我们使用了Python内置的xml.etree.ElementTree模块来进行XML的操作。首先,我们读取了txt文件的内容,然后创建了根节点,循环读取每一行数据并创建子节点,最后将根节点转换为xml字符串并保存到文件中。
相关问题
转换两种txt标签为xml的代码
以下是将两种不同的txt标签转换为XML的Python代码示例:
假设我们有两个txt文件: `file1.txt` 和 `file2.txt` ,它们的内容分别如下:
`file1.txt`:
```
NAME: John
AGE: 30
CITY: New York
```
`file2.txt`:
```
<book>
<title>Harry Potter</title>
<author>J.K. Rowling</author>
<year>1997</year>
</book>
```
我们将使用Python中的ElementTree模块来将这些txt标签转换为XML。下面是示例代码:
```python
import xml.etree.ElementTree as ET
# 将file1.txt中的标签转换为XML
with open('file1.txt', 'r') as f:
xml_data = '<root>\n'
for line in f:
tag, data = line.strip().split(':')
xml_data += f'<{tag.lower()}>{data}</{tag.lower()}>\n'
xml_data += '</root>'
# 将XML写入文件
with open('file1.xml', 'w') as xml_file:
xml_file.write(xml_data)
# 将file2.txt中的标签转换为XML
with open('file2.txt', 'r') as f:
xml_data = f.read()
# 将字符串转换为Element对象
root = ET.fromstring(xml_data)
# 将Element对象写入文件
ET.ElementTree(root).write('file2.xml')
```
运行以上代码后,会生成两个新的XML文件:`file1.xml` 和 `file2.xml`,它们的内容分别如下:
`file1.xml`:
```xml
<root>
<name>John</name>
<age>30</age>
<city>New York</city>
</root>
```
`file2.xml`:
```xml
<book>
<title>Harry Potter</title>
<author>J.K. Rowling</author>
<year>1997</year>
</book>
```
注意,第二个文件已经是XML格式了,因此我们只需将其字符串转换为Element对象,然后使用ElementTree模块将其写入文件即可。
读取coco标签并转化为VOC标签python代码
以下是一个将COCO标签转化为VOC标签的Python代码例:
```python
import json
from xml.etree.ElementTree import Element, SubElement, tostring
from xml.dom.minidom import parseString
def coco2voc(coco_path, voc_path):
with open(coco_path, 'r') as f:
coco_data = json.load(f)
voc_root = Element('annotation')
# 添加文件名
filename = SubElement(voc_root, 'filename')
filename.text = coco_data['image']['file_name']
# 添加图像尺寸
size = SubElement(voc_root, 'size')
width = SubElement(size, 'width')
width.text = str(coco_data['image']['width'])
height = SubElement(size, 'height')
height.text = str(coco_data['image']['height'])
depth = SubElement(size, 'depth')
depth.text = '3' # 假设是RGB图像
# 添加目标边界框
for ann in coco_data['annotations']:
obj = SubElement(voc_root, 'object')
name = SubElement(obj, 'name')
name.text = coco_data['categories'][ann['category_id'] - 1]['name']
bbox = ann['bbox']
xmin = SubElement(obj, 'xmin')
xmin.text = str(bbox[0])
ymin = SubElement(obj, 'ymin')
ymin.text = str(bbox[1])
xmax = SubElement(obj, 'xmax')
xmax.text = str(bbox[0] + bbox[2])
ymax = SubElement(obj, 'ymax')
ymax.text = str(bbox[1] + bbox[3])
# 将XML转换为字符串并保存到文件
xml_str = parseString(tostring(voc_root)).toprettyxml(indent=' ')
with open(voc_path, 'w') as f:
f.write(xml_str)
# 示例用法
coco_path = 'path/to/coco.json'
voc_path = 'path/to/voc.xml'
coco2voc(coco_path, voc_path)
```
请将`coco_path`修改为你的COCO标签文件的路径,将`voc_path`修改为你想要保存VOC标签的路径。运行代码后,将生成一个符合VOC标签格式的XML文件。
相关推荐
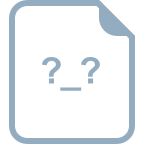
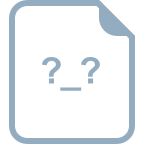
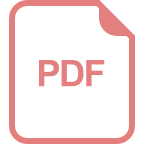












