帮我实现yolo格式标签文件转化为XML标签文件
时间: 2024-04-07 11:33:34 浏览: 50
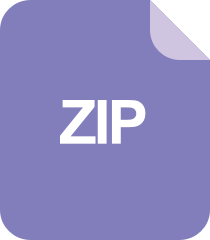
MNIST手写字体的yolo和voc格式数据集标签
当将YOLO格式的标签文件转换为XML格式的标签文件时,您可以按照以下步骤进行操作:
1. 解析YOLO格式的标签文件,获取每个边界框的类别、边界框左上角坐标和宽高。
2. 创建一个XML文件,并设置根节点。
3. 针对每个边界框,创建一个XML子节点,并添加类别、边界框坐标和宽高的子节点。
4. 将XML内容保存到文件中。
以下是一个使用Python实现的示例代码:
```python
import xml.etree.ElementTree as ET
def convert_yolo_to_xml(yolo_file, output_file):
with open(yolo_file, 'r') as f:
lines = f.readlines()
root = ET.Element("annotations")
for line in lines:
line = line.strip().split()
class_id = int(line[0])
x_center = float(line[1])
y_center = float(line[2])
width = float(line[3])
height = float(line[4])
xmin = int((x_center - width / 2) * image_width)
ymin = int((y_center - height / 2) * image_height)
xmax = int((x_center + width / 2) * image_width)
ymax = int((y_center + height / 2) * image_height)
box = ET.SubElement(root, "object")
name = ET.SubElement(box, "name")
name.text = str(class_id)
bndbox = ET.SubElement(box, "bndbox")
xmin_node = ET.SubElement(bndbox, "xmin")
xmin_node.text = str(xmin)
ymin_node = ET.SubElement(bndbox, "ymin")
ymin_node.text = str(ymin)
xmax_node = ET.SubElement(bndbox, "xmax")
xmax_node.text = str(xmax)
ymax_node = ET.SubElement(bndbox, "ymax")
ymax_node.text = str(ymax)
tree = ET.ElementTree(root)
tree.write(output_file)
# 使用示例
yolo_file = 'path/to/yolo.txt'
output_file = 'path/to/xml.xml'
image_width = 640
image_height = 480
convert_yolo_to_xml(yolo_file, output_file)
```
请将示例代码中的`yolo_file`替换为YOLO格式的标签文件路径,`output_file`替换为您希望保存XML标签文件的路径,`image_width`和`image_height`替换为图像的宽度和高度。运行代码后,将生成一个包含边界框信息的XML标签文件。
阅读全文
相关推荐
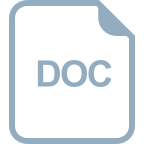
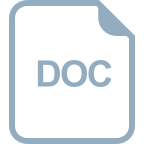
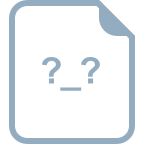
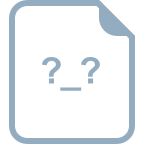
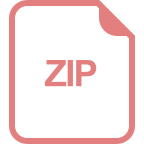







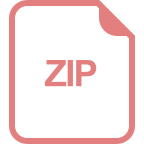




