使用python,将文件夹中所有的labelme标注生成的json文件转换为可以用于labelimg标注的yolo格式的xml文件,且文件名与原文件名一致,且json文件中存在shape_type为circle的情况,生成的yaml中的标签需为circle的外切矩形,最后将生成的xml文件放在新文件夹里
时间: 2024-02-15 18:05:14 浏览: 87
好的,您可以使用以下代码实现:
```python
import os
import json
import xml.etree.ElementTree as ET
# 定义标签和颜色
LABELS = {'circle': 0}
COLORS = {'circle': '0,255,0'}
# 定义json文件路径和xml文件路径
json_dir = '/path/to/json/dir'
xml_dir = '/path/to/xml/dir'
# 遍历json文件夹中的所有文件
for json_file in os.listdir(json_dir):
# 如果不是json文件,则跳过
if not json_file.endswith('.json'):
continue
# 读取json文件内容
with open(os.path.join(json_dir, json_file), 'r') as f:
data = json.load(f)
# 获取文件名(去掉扩展名)
filename = os.path.splitext(json_file)[0]
# 创建xml文件
root = ET.Element('annotation')
# 添加filename元素
ET.SubElement(root, 'filename').text = filename + '.jpg'
# 添加size元素
size = ET.SubElement(root, 'size')
ET.SubElement(size, 'width').text = str(data['imageWidth'])
ET.SubElement(size, 'height').text = str(data['imageHeight'])
ET.SubElement(size, 'depth').text = '3'
# 遍历shapes
for shape in data['shapes']:
label = shape['label']
if label not in LABELS:
continue
# 添加object元素
obj = ET.SubElement(root, 'object')
ET.SubElement(obj, 'name').text = label
ET.SubElement(obj, 'pose').text = 'Unspecified'
ET.SubElement(obj, 'truncated').text = '0'
ET.SubElement(obj, 'difficult').text = '0'
# 添加bndbox元素
bndbox = ET.SubElement(obj, 'bndbox')
if shape['shape_type'] == 'circle':
# 如果是圆形,获取外切矩形
x, y = shape['points'][0]
r = shape['points'][1][0] - x
ET.SubElement(bndbox, 'xmin').text = str(round(x - r))
ET.SubElement(bndbox, 'ymin').text = str(round(y - r))
ET.SubElement(bndbox, 'xmax').text = str(round(x + r))
ET.SubElement(bndbox, 'ymax').text = str(round(y + r))
else:
# 否则,获取矩形
x1, y1 = shape['points'][0]
x2, y2 = shape['points'][1]
ET.SubElement(bndbox, 'xmin').text = str(round(min(x1, x2)))
ET.SubElement(bndbox, 'ymin').text = str(round(min(y1, y2)))
ET.SubElement(bndbox, 'xmax').text = str(round(max(x1, x2)))
ET.SubElement(bndbox, 'ymax').text = str(round(max(y1, y2)))
# 添加difficult元素
ET.SubElement(obj, 'difficult').text = '0'
# 添加bndbox颜色
ET.SubElement(obj, 'color').text = COLORS[label]
# 保存xml文件
xml_file = os.path.join(xml_dir, filename + '.xml')
tree = ET.ElementTree(root)
tree.write(xml_file)
```
这段代码会将指定文件夹中的所有json文件转换为xml文件,并将xml文件保存到指定文件夹中。处理圆形标注时,会将圆形转换为外切矩形。同时,会为每个标注添加颜色信息,以便在标注时更加直观。
阅读全文
相关推荐
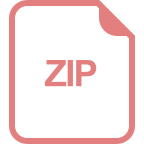
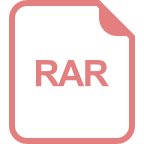
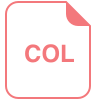
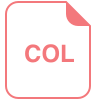
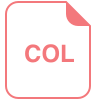
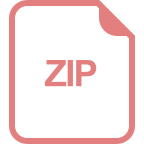
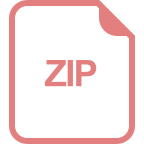
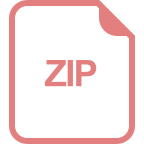
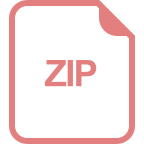
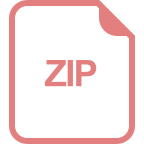
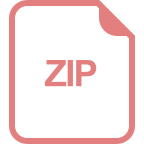
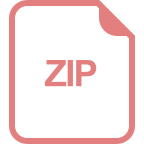
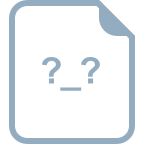
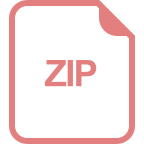
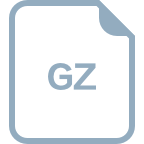
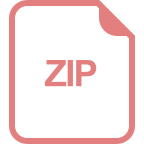