请帮我写一个脚本:将yolo格式的txt文件转成xml格式,其中类别为0:ztc 1:wjj 2:snc 3:td 4:ttc
时间: 2024-02-27 19:58:11 浏览: 121
好的,以下是一个将YOLO格式的标签文件转换为XML格式的脚本,其中类别为0:ztc 1:wjj 2:snc 3:td 4:ttc。请将脚本保存为.py文件,然后在命令行中运行。
```python
import os
import xml.etree.ElementTree as ET
# Define the class names
CLASS_NAMES = ["ztc", "wjj", "snc", "td", "ttc"]
def convert_yolo_to_xml(yolo_file, output_dir):
# Open the YOLO format file and read each label line
with open(yolo_file) as f:
labels = f.readlines()
# Create the root XML element
root = ET.Element("annotation")
# Create the child elements and add them to the root element
filename = os.path.splitext(os.path.basename(yolo_file))[0] + ".jpg"
ET.SubElement(root, "filename").text = filename
size = ET.SubElement(root, "size")
ET.SubElement(size, "width").text = "416"
ET.SubElement(size, "height").text = "416"
ET.SubElement(size, "depth").text = "3"
# Loop over each label and add it to the XML file
for label in labels:
label_parts = label.strip().split()
label_name = CLASS_NAMES[int(label_parts[0])]
x_center = float(label_parts[1])
y_center = float(label_parts[2])
width = float(label_parts[3])
height = float(label_parts[4])
left = int((x_center - width / 2) * 416)
top = int((y_center - height / 2) * 416)
right = int((x_center + width / 2) * 416)
bottom = int((y_center + height / 2) * 416)
object_element = ET.SubElement(root, "object")
ET.SubElement(object_element, "name").text = label_name
bbox = ET.SubElement(object_element, "bndbox")
ET.SubElement(bbox, "xmin").text = str(left)
ET.SubElement(bbox, "ymin").text = str(top)
ET.SubElement(bbox, "xmax").text = str(right)
ET.SubElement(bbox, "ymax").text = str(bottom)
# Write the XML file to disk
xml_file = os.path.join(output_dir, os.path.splitext(os.path.basename(yolo_file))[0] + ".xml")
tree = ET.ElementTree(root)
tree.write(xml_file)
```
使用时,只需要将YOLO格式的标签文件路径和输出目录路径作为参数传递给convert_yolo_to_xml函数即可。例如:
```python
convert_yolo_to_xml("path/to/label.txt", "path/to/output/dir")
```
注意,此脚本假设YOLO格式的标签文件中的坐标是相对于图像宽度和高度的百分比,因此需要将它们转换为像素坐标。此外,如果YOLO格式的标签文件中的类别编号与CLASS_NAMES列表中定义的顺序不同,则需要相应地修改代码。
阅读全文
相关推荐
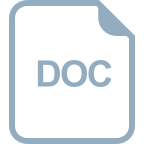
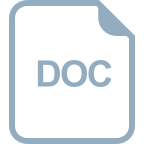
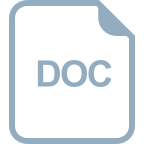
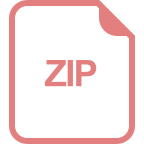
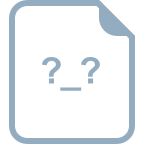
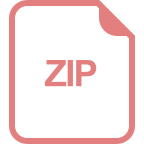
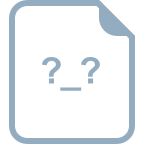
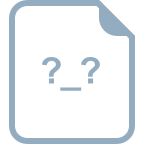
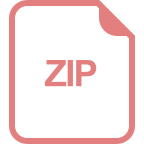
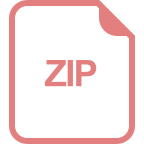
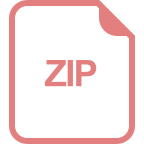
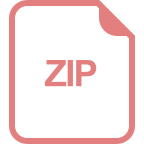
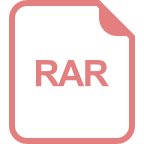
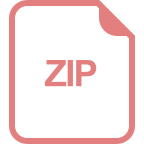