输入一个字符串,统计每个字符出现的次数,输出最大次数。
时间: 2023-05-31 09:03:03 浏览: 118
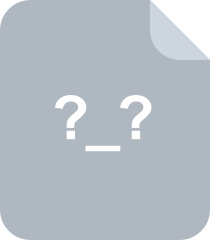
统计一个字符串中每个字符出现的次数
可以使用字典来统计每个字符出现的次数,然后找出出现次数最大的值即可。
示例代码如下:
```python
s = input("请输入字符串:")
# 使用字典统计每个字符出现的次数
count = {}
for c in s:
count[c] = count.get(c, 0) + 1
# 找出出现次数最大的值
max_count = max(count.values())
# 输出结果
print("每个字符出现的次数:", count)
print("最大次数为:", max_count)
```
运行示例:
```
请输入字符串:hello world
每个字符出现的次数: {'h': 1, 'e': 1, 'l': 3, 'o': 2, ' ': 1, 'w': 1, 'r': 1, 'd': 1}
最大次数为: 3
```
阅读全文
相关推荐















