Java生成表格放入邮件
时间: 2023-10-08 22:07:45 浏览: 30
要将Java生成的表格放入邮件,你需要使用JavaMail库。以下是一个示例程序,演示如何创建一个带表格的邮件:
```
import java.util.Properties;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeTable;
import javax.activation.DataHandler;
import javax.activation.DataSource;
import javax.activation.FileDataSource;
public class EmailExample {
public static void main(String[] args) {
String to = "recipient@example.com"; // 收件人邮箱
String from = "sender@example.com"; // 发件人邮箱
String host = "smtp.example.com"; // 邮件服务器
String subject = "Table Example"; // 邮件主题
// 创建邮件会话
Properties properties = System.getProperties();
properties.setProperty("mail.smtp.host", host);
Session session = Session.getDefaultInstance(properties);
try {
// 创建邮件
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(from));
message.addRecipient(Message.RecipientType.TO, new InternetAddress(to));
message.setSubject(subject);
// 创建表格
MimeTable table = new MimeTable(4, 2);
table.addRow(true, "Name", "Age");
table.addRow(false, "Alice", "20");
table.addRow(true, "Bob", "25");
table.addRow(false, "Charlie", "30");
// 将表格添加到邮件正文中
MimeMultipart multipart = new MimeMultipart();
MimeBodyPart bodyPart = new MimeBodyPart();
bodyPart.setContent(table, "text/html");
multipart.addBodyPart(bodyPart);
message.setContent(multipart);
// 发送邮件
Transport.send(message);
System.out.println("Email sent successfully.");
} catch (MessagingException ex) {
ex.printStackTrace();
}
}
}
```
在上面的示例中,我们创建了一个4行2列的表格,并将其添加到邮件正文中。你可以根据自己的需求更改表格的行数、列数、内容和样式。最后,我们使用Transport类的send()方法发送邮件。注意,你需要替换示例中的收件人邮箱、发件人邮箱和邮件服务器信息。
相关推荐
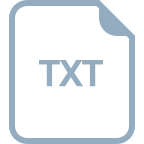
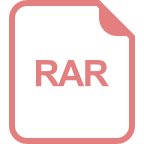
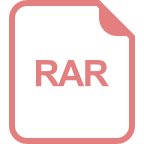














