【网络安全】:Java字符串处理在安全中的应用案例分析
发布时间: 2024-08-29 13:36:51 阅读量: 40 订阅数: 28 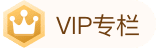
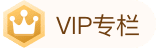
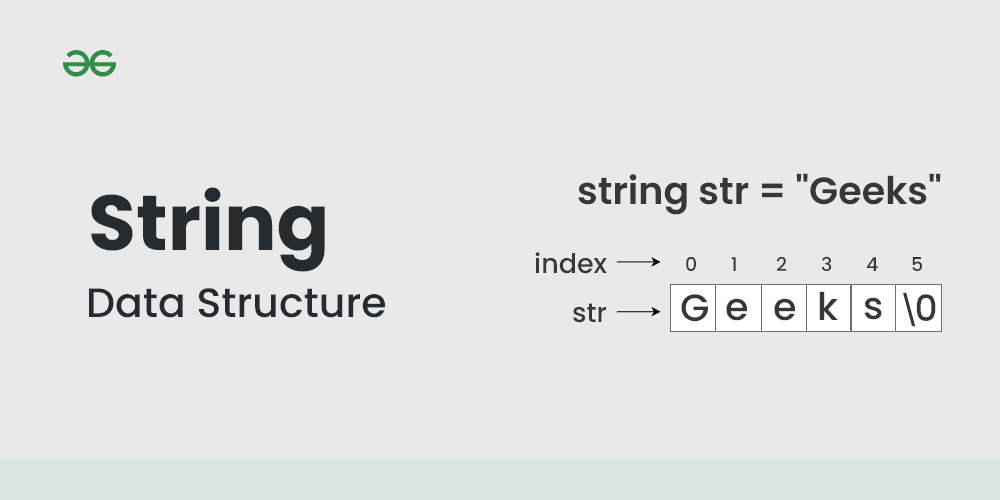
# 1. Java字符串处理概述
## 简介
在Java编程语言中,字符串(String)是处理文本的核心数据类型。随着应用的复杂性增加,字符串处理的能力和效率直接影响软件的性能与安全性。在实际开发过程中,熟练掌握字符串操作是每一位Java开发者的基本功。
## 字符串的基础知识
Java中的字符串是不可变的,意味着一旦创建便无法更改。对于字符串的操作,Java提供了丰富的方法,包括但不限于字符串的创建、拼接、比较、截取、替换和转换等。这些方法可以在`String`类中找到,或者使用相关的工具类如`StringBuilder`和`StringBuffer`来优化操作性能。
## 字符串处理的重要性
在安全性方面,字符串处理不当可能导致应用程序易受攻击,例如,未经充分验证和清洗的用户输入可能会成为注入攻击的媒介。因此,在进行字符串操作时,开发者不仅需要关注代码的执行效率,还应确保程序的安全性。这一章节将为后续深入探讨字符串处理在网络安全中的应用奠定基础。
了解了字符串在Java中的基本概念和重要性,接下来章节中我们将探索它在网络安全中的应用和最佳实践。
# 2. 字符串处理在网络安全中的理论基础
### 2.1 字符串处理的安全性原理
#### 2.1.1 字符串加密与解密原理
在网络安全的领域中,字符串的加密与解密是保护敏感信息不被未授权访问的重要手段。加密的过程涉及将明文转换为密文,而解密则是将密文还原回可读的明文。这一过程主要依赖于加密算法和密钥。
加密算法可以分为对称加密和非对称加密两大类。对称加密使用相同的密钥进行加密和解密,例如AES(高级加密标准)和DES(数据加密标准)。非对称加密则使用一对密钥——公钥和私钥,公钥可以公开,私钥需保密,如RSA算法。在实际应用中,对称加密由于速度快,常用于数据的加密传输;而非对称加密由于密钥管理复杂,通常用于加密对称密钥或数字签名。
安全性原理还涉及密钥管理,因为无论加密算法多么强大,一旦密钥泄露,则安全保护立即失效。因此,密钥的生成、存储、分发和更新都必须严格控制,确保安全。
```java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.security.SecureRandom;
public class EncryptionExample {
public static void main(String[] args) throws Exception {
// 生成密钥
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
keyGenerator.init(128, new SecureRandom());
SecretKey secretKey = keyGenerator.generateKey();
// 加密
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
byte[] encrypted = cipher.doFinal("Hello World!".getBytes());
System.out.println("Encrypted text: " + bytesToHex(encrypted));
// 解密
cipher.init(Cipher.DECRYPT_MODE, secretKey);
byte[] decrypted = cipher.doFinal(encrypted);
System.out.println("Decrypted text: " + new String(decrypted));
}
// 辅助方法,将字节数组转换为十六进制字符串
private static String bytesToHex(byte[] bytes) {
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
}
}
```
在上述Java代码示例中,使用了AES加密算法对字符串"Hello World!"进行加密和解密。在加密过程中,我们使用`KeyGenerator`生成了AES密钥,并用`Cipher`类进行加密和解密操作。输出结果表明,原始字符串被成功地转换为密文,并能够还原。
#### 2.1.2 字符串校验与完整性保证
字符串校验是网络安全中的另一个重要环节。校验的目的在于确保数据在传输或存储过程中未被篡改。消息摘要算法(如MD5、SHA-1和SHA-256)常用于这一目的,它们通过哈希函数生成原始数据的固定长度的哈希值(摘要),用于校验数据的完整性。
由于哈希函数的特性是单向的且对于输入的微小变化会产生截然不同的输出,因此它们被广泛用于验证数据的完整性。即使数据被截获,没有密钥的情况下几乎不可能从哈希值反推出原始数据。然而需要注意的是,由于密码学中的碰撞攻击,MD5和SHA-1等算法已不再被认为是安全的,更推荐使用SHA-256或更高版本。
```java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class HashingExample {
public static void main(String[] args) throws NoSuchAlgorithmException {
String data = "Hello World!";
System.out.println("Original data: " + data);
// 创建SHA-256摘要
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] encodedhash = digest.digest(data.getBytes());
System.out.println("SHA-256 hash: " + bytesToHex(encodedhash));
// 验证数据完整性
// 假设这是从不可信源接收到的数据的哈希
byte[] receivedHash = hexStringToByteArray("接收的哈希值");
digest.update(data.getBytes());
byte[] calculatedHash = digest.digest();
if (MessageDigest.isEqual(receivedHash, calculatedHash)) {
System.out.println("数据完整性验证成功");
} else {
System.out.println("数据完整性验证失败");
}
}
// 将十六进制字符串转换为字节数组
private static byte[] hexStringToByteArray(String s) {
int len = s.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(s.charAt(i), 16) << 4)
+ Character.digit(s.charAt(i+1), 16));
}
return data;
}
}
```
上述代码通过Java的`MessageDigest`类来生成数据的SHA-256哈希值。在实际应用中,发送方会计算数据的哈希值并发送给接收方,接收方收到数据后会重新计算哈希值并与发送方传来的哈希值进行比较,从而验证数据是否在传输过程中被篡改。这里展示了数据校验的完整流程。
### 2.2 安全编码实践
#### 2.2.1 输入验证与清洗
输入验证是网络安全的基石,它确保用户输入的数据是符合预期格式的,并且不包含潜在的攻击代码。对于Web应用而言,输入验证不仅应该在服务器端进行,也应当在客户端进行,以减少服务器的负载并提高响应速度。
清洗是输入验证的一种形式,其目标是移除或替换用户输入中的潜在危险字符。例如,在一个表单中,如果期望的输入是电子邮件地址,那么任何不包含"@"符号的输入都应当被拒绝。同样,任何HTML或JavaScript代码片段都应从输入中清除,以防止XSS攻击。
在Java中,可以使用正则表达式进行输入的验证和清洗:
```java
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class InputValidationExample {
public static boolean isValidEmail(String email) {
String emailRegex = "^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$";
Pattern pattern = ***pile(emailRegex);
Matcher matcher = pattern.matcher(email);
return matcher.matches();
}
public static void main(String[] args) {
String inputEmail = "***";
boolean isEmailValid = isValidEmail(inputEmail);
System.out.println("Is email valid? " + isEmailValid);
}
}
```
此例中使用了正则表达式来验证电子邮件地址是否格式正确。通过定义的`isValidEmail`函数,输入的字符串被检查是否匹配电子邮件格式的正则表达式。
#### 2.2.2 防止注入攻击的编码策略
注入攻击,如SQL注入、命令注入等,是网络安全中非常常见的攻击方式。它们通过在输入中嵌入恶意代码片段来破坏程序的逻辑。防止注入攻击的关键在于严格的输入验证、参数化查询和使用安全API。
参数化查询是一种防止SQL注入的常用策略。它通过使用预定义的SQL命令模板和参数来执行SQL查询,而不允许用户输入直接拼接到SQL语句中。Java中可以使用JDBC提供的预处理语句(PreparedStatement)来实现参数化查询:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class PreparedStatementExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "myusername";
String password = "mypassword";
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DriverManager.getConnection(url, user, password);
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, "admin");
pstmt.setString(2, "adminpass");
boolean resultsFound = pstmt.execute();
if (resultsFound) {
// 处理查询结果
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接和语句对象
try {
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在这个示例中,使用`PreparedStatement`来执行带有两个参数的SQL查询,它能够有效地防止SQL注入攻击。即使用户输入了恶意SQL代码,也只会被当作普通字符串处理,不会影响SQL命令的执行。
#### 2.2.3 安全的字符串比较方法
在比较字符串时,特别是在处理密码或敏感信息时,重要的是使用安全的方法来比较两个字符串是否相等,
0
0
相关推荐
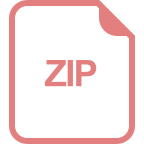
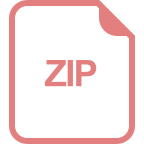
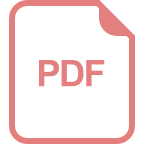





