【字符串池机制】:Java深入解析及高效应用
发布时间: 2024-08-29 13:14:52 阅读量: 15 订阅数: 28 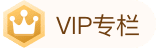
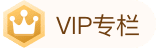

# 1. 字符串池机制概述
在现代编程实践中,字符串是使用最频繁的数据类型之一。对于Java和许多其他语言来说,字符串池(也称为字符串常量池)是一个用于优化内存使用和提高性能的特殊内存区域。字符串池的核心思想是在内存中维护一个字符串缓存,这样重复创建相同内容的字符串实例时,可以重用缓存中的对象,而不是每次都分配新的内存空间。这不仅可以减少内存消耗,还可以加速字符串比较和检索操作。
字符串池机制的实现通常是通过维护一个哈希表来实现的。当字符串对象被创建时,系统会先检查哈希表中是否已经存在内容相同的对象。如果存在,就返回该对象的引用,否则就创建一个新的字符串对象,添加到哈希表中,并返回其引用。
尽管字符串池带来了许多好处,但同时它也引入了一些复杂性,特别是在多线程环境下。理解字符串池的内部工作机制及其对性能的潜在影响对于每个IT专业人士来说都是至关重要的。在后续章节中,我们将深入探讨字符串池的内部实现、性能影响、实际应用场景、深入分析以及在Java虚拟机中的具体实现细节。
# 2. 字符串池的内部实现
## 2.1 字符串字面量池的结构和工作原理
### 2.1.1 字符串常量池的概念与作用
字符串常量池是Java内存管理的一部分,负责存储程序中定义的字符串字面量。这些字符串字面量一旦创建,就会被放入常量池中,以便后续使用时可以快速检索和复用,从而提高内存使用效率和程序性能。
字符串常量池位于JVM的运行时常量池中,运行时常量池是方法区的一部分。在Java 7及之后的版本中,字符串常量池被移至堆内存中,从而优化了字符串的存储和处理速度。
### 2.1.2 字符串常量池在内存中的存储方式
字符串常量池在内存中的存储通常使用哈希表来实现,以实现快速的检索。每个字符串在常量池中都对应一个唯一的哈希码,这个哈希码是通过字符串的内容计算得出的。
在Java中,`String.intern()` 方法可以用来获取字符串在常量池中的引用。如果常量池中已经存在该字符串的引用,就直接返回;如果不存在,就创建新的引用,并将其添加到常量池中。
### 2.1.3 字符串字面量的存储和检索过程
当Java程序中出现字符串字面量时,如 `"Hello World"`,JVM首先会检查字符串常量池中是否已经存在相同内容的字符串。如果存在,就直接返回已有的引用;如果不存在,就在常量池中创建一个新的字符串对象,并将它的引用返回给程序。
这个过程涉及到一个关键的概念——字符串驻留。字符串驻留是指字符串字面量和字符串常量池中的引用关联起来,使得在JVM中对于相同的字符串字面量,只有一个对象实例存在。
```java
String s1 = "Hello World";
String s2 = "Hello World";
System.out.println(s1 == s2); // 输出 true
```
在上面的例子中,`s1` 和 `s2` 指向的是同一个字符串对象,因为字符串常量池中只有一个 `"Hello World"`。
## 2.2 intern方法与字符串池的关系
### 2.2.1 intern方法的工作机制
`intern()` 方法是Java中一个重要的字符串操作方法,它用于确保字符串对象在字符串常量池中只有一个副本。当调用一个字符串对象的 `intern()` 方法时,如果字符串常量池中已经存在一个相同的字符串,则返回常量池中的引用;否则,将这个字符串对象添加到常量池中,并返回它的引用。
### 2.2.2 字符串对象的intern方法示例分析
```java
String s1 = "Hello";
String s2 = new String("Hello").intern();
String s3 = "Hello";
System.out.println(s1 == s2); // 输出 false,s1在常量池,s2在堆中
System.out.println(s2 == s3); // 输出 true,s2和s3都指向常量池中的同一个字符串
```
在上述代码中,`s1` 是直接在常量池中创建的字符串,而 `s2` 是先创建了一个新的堆字符串,然后通过 `intern()` 方法将其添加到常量池中。`s3` 直接引用常量池中的字符串。
### 2.2.3 字符串池中重复字符串的处理
重复字符串的处理是字符串常量池的重要功能之一。当程序尝试创建一个已存在于常量池中的字符串时,JVM会直接返回常量池中已经存在的字符串对象,而不会创建新的对象。这样做可以避免内存中存储多个相同内容的字符串对象,从而节约内存资源。
```java
String s1 = "Java";
String s2 = "Java";
System.out.println(s1 == s2); // 输出 true,s1和s2指向同一个对象
```
在这个例子中,无论创建多少次 `"Java"` 字符串,由于字符串常量池的存在,它们都指向同一个内存地址。
## 2.3 字符串池的性能影响和优化策略
### 2.3.1 字符串池对内存管理的影响
字符串池的使用对Java程序的内存管理有重要的影响。它可以减少字符串对象的重复创建,减少内存占用,提高内存的使用效率。特别是在大型应用中,合理的使用字符串池可以显著减少内存碎片,提高垃圾回收的效率。
### 2.3.2 字符串池优化内存使用的场景
字符串池优化内存使用的场景包括但不限于:
- 大量重复字符串的场景,如日志输出、配置文件中的字符串等。
- 字符串拼接操作频繁的场景,使用字符串池可以避免创建大量中间字符串对象。
- 在Web应用中,通常会有大量的请求和响应,这些都涉及到字符串的操作,字符串池可以显著提高处理速度。
### 2.3.3 避免字符串池性能问题的方法
为了避免字符串池引起的性能问题,开发者可以采取以下一些方法:
- 合理使用 `intern()` 方法来确保字符串实例的唯一性。
- 对于大量的字符串操作,考虑使用 `StringBuilder` 或 `StringBuffer`,避免使用 `+` 操作符,这样可以减少不必要的字符串对象创建。
- 在JVM启动时通过设置 `-XX:+UseStringDeduplication` 参数启用JVM的字符串去重功能,进一步优化内存使用。
```java
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("a");
}
String result = sb.toString();
String internedResult = result.intern();
System.out.println(result == internedResult); // 输出 true
```
在这个例子中,通过 `StringBuilder` 构建了一个长字符串,然后使用 `intern()` 方法将其添加到字符串常量池中。由于字符串已经存在于常量池中,所以 `intern()` 方法返回的是相同的引用。
# 3. 字符串池的实际应用场景
## 3.1 字符串池与Java集合框架
### 3.1.1 集合框架中字符串池的使用
在Java集合框架中,字符串池的概念可以提供显著的性能优化。当使用集合框架存储字符串时,如`ArrayList`, `HashSet`, 或者`HashMap`等,这些集合经常被用来存储大量的数据。如果这些数据中包含了
0
0
相关推荐
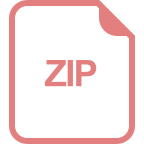
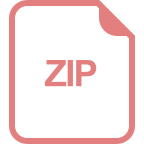
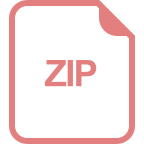





