【正则表达式优化】:Java高级应用与性能提升技巧
发布时间: 2024-08-29 13:00:41 阅读量: 32 订阅数: 28 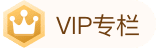
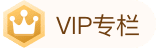
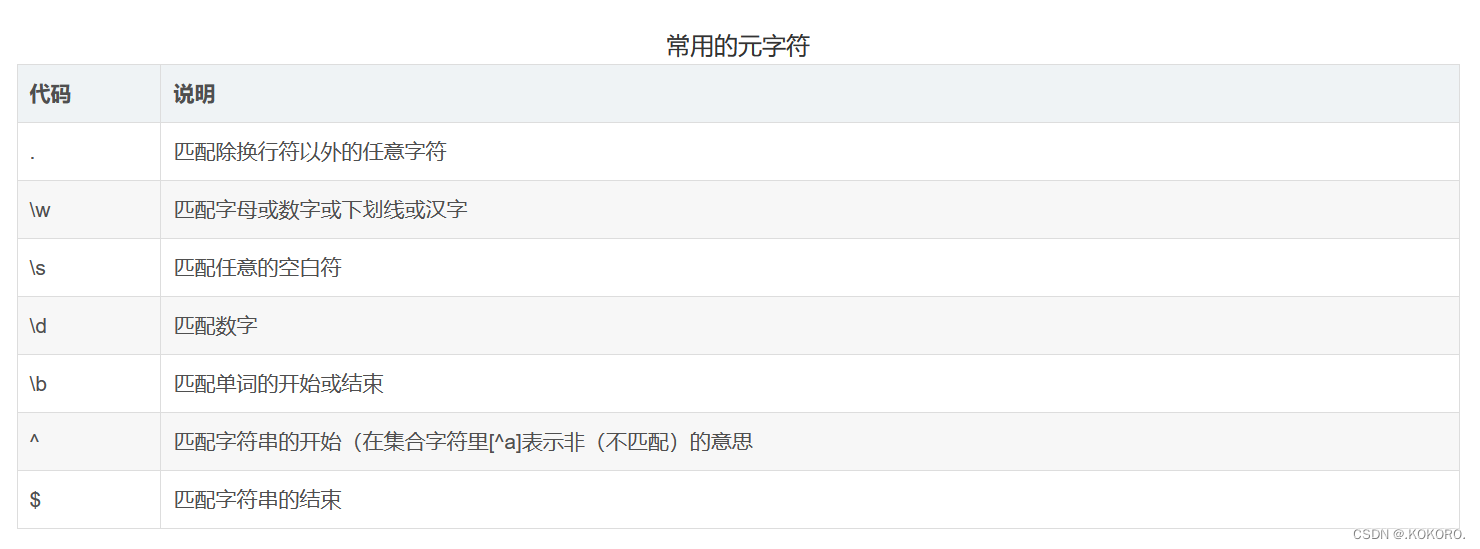
# 1. 正则表达式基础及在Java中的应用
在现代软件开发中,正则表达式是用于匹配字符串中字符组合的一种强大工具。正则表达式不仅能有效识别文本模式,还能在数据处理、文本分析和验证等多种场景中发挥作用。本章将首先介绍正则表达式的概念、结构及其在Java语言中的基本应用方法。通过实例演示,帮助读者建立起使用正则表达式处理字符串数据的初步能力。
正则表达式通常由普通字符和元字符组成。普通字符代表它们自身,如字母或数字,而元字符则拥有特殊含义,例如星号(*)表示前面的字符可以出现零次或多次。掌握这些基本元素,是编写正则表达式的前提。
Java通过`java.util.regex`包中的`Pattern`和`Matcher`类支持正则表达式。开发者通过这两个类,可以创建模式对象并使用它来查找和替换字符串中的子串。以下是一个简单的例子:
```java
import java.util.regex.Pattern;
import java.util.regex.Matcher;
public class RegexExample {
public static void main(String[] args) {
String text = "Hello World!";
Pattern pattern = ***pile("Hello");
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Found 'Hello': " + matcher.group());
}
}
}
```
上述代码将打印出 "Found 'Hello': Hello",表明找到了"Hello"这一模式。这一章的内容将为读者理解后续章节打下坚实的基础。
# 2. 正则表达式的性能优化技巧
## 2.1 理解正则表达式的原理
### 2.1.1 正则表达式的构成与匹配流程
正则表达式(Regular Expressions),简称regex,是一种用于文本匹配的强大力量。它由一系列字符和符号组成,用来描述或者限定一组字符串。正则表达式的构成包括普通字符(比如字母和数字)和特殊字符(如"*", "+", "?", "^", "$" 等)。
匹配流程一般遵循从左到右的顺序,正则表达式引擎将每一个字符或者字符集与目标字符串逐个进行比较,一旦发现不匹配就回溯到前一个可能的匹配位置,并尝试其他的匹配路径。这个过程称为回溯。回溯是许多正则表达式实现的核心机制,但也是造成性能问题的主要原因。
### 2.1.2 回溯机制及其对性能的影响
回溯机制允许正则表达式在部分匹配失败后,退回前一个步骤,尝试其他可能的匹配。这个机制在处理含有量词和选择结构的正则表达式时显得尤为重要。
然而,回溯也会导致大量的计算和时间开销。特别是在处理包含复杂嵌套、贪婪量词等情况下,性能问题尤为突出。例如,正则表达式 `a*a` 需要进行多次回溯才能完成匹配,特别是在目标字符串以多个 'a' 结尾时。
```regex
a*a
```
如果目标字符串是 "aaaaaaaaaa",正则表达式引擎需要尝试匹配不同的 'a' 长度组合,直到找到所有可能的匹配为止,这个过程效率极低。
## 2.2 优化正则表达式的设计
### 2.2.1 避免嵌套量词和捕获组
嵌套量词,比如 `(a*)*` 中的 `a*` 可以匹配任意多的 'a' 字符,外层的 `*` 使得它成为一个嵌套结构。这种嵌套结构可能触发指数级的回溯,应当避免使用。
捕获组 `( )` 用于捕获匹配的文本,以便后续使用。然而,每创建一个捕获组都可能增加额外的处理开销。在不需要捕获匹配的文本时,应尽量避免使用捕获组。
### 2.2.2 精确控制匹配范围与边界
通过精确地控制匹配的开始和结束边界,可以避免不必要的匹配尝试。例如,使用 `\b` 可以明确表示单词的边界,这样可以避免在非单词字符上进行匹配尝试。
```regex
\bword\b
```
这个正则表达式将确保只匹配独立的单词 'word',而不会错误地匹配到 'swordfish' 中的 'word'。
### 2.2.3 使用懒惰量词和否定前瞻
懒惰量词(也称作非贪婪量词),比如 `*?`,`+?` 和 `??`,匹配尽可能少的字符。在可以的情况下使用懒惰量词,可以减少匹配尝试的次数,提高性能。
否定前瞻(如 `(?!)` 和 `(?<!)`)提供了一种检查一个字符串是否出现在另一个字符串之前或之后的方法,而不消耗任何字符。这可以在很多情况下减少不必要的回溯。
## 2.3 Java代码中的正则表达式优化
### 2.3.1 使用StringBuilder优化字符串拼接
在Java中,频繁使用 `+` 操作符拼接字符串会产生大量的临时对象,增加垃圾回收的压力。使用 `StringBuilder` 或 `StringBuffer` 可以显著提升性能。
```java
StringBuilder sb = new StringBuilder();
for (String s : strings) {
sb.append(s);
}
String result = sb.toString();
```
在这个例子中,`StringBuilder` 被用来收集所有的字符串,最终一次性生成结果字符串。
### 2.3.2 利用Pattern和Matcher类的高级特性
Java的 `Pattern` 和 `Matcher` 类提供了许多高级特性,可以用来优化正则表达式的执行。例如,`Matcher.find()` 方法会从当前位置开始查找匹配,可以用来逐步解析一个大文本,而不是在一次操作中完成所有匹配。
```java
Pattern pattern = ***pile("your_regex");
Matcher matcher = pattern.matcher(yourText);
while (matcher.find()) {
// 处理匹配
}
```
使用这种方式可以有效地控制内存和处理时间,对于非常大的文本尤其有用。
在下一章节中,我们将探讨正则表达式在Java中的实战应用,并展示如何在不同的应用场景中使用上述优化技巧。
# 3. Java正则表达式的实战应用
正则表达式是处理文本和数据的强大工具,它允许程序员以简洁的语法来描述复杂的文本模式。在Java中,正则表达式应用广泛,从简单的数据验证到复杂的文本搜索和分析。本章将探讨Java正则表达式在不同场景中的实战应用,并展示如何在实际项目中有效地利用它们。
## 3.1 数据验证与清洗
### 3.1.1 校验电子邮件和电话号码格式
在处理用户输入时,验证数据的有效性是重要的一步。电子邮件地址和电话号码的格式验证是常见的需求。正则表达式可以用来校验这些信息是否符合期望的格式。
例如,一个简单的电子邮件格式正则表达式如下:
```java
String emailRegex = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,6}$";
```
电话号码验证可以通过不同的正则表达式来匹配不同国家的号码格式。下面是一个示例,适用于美国和加拿大的电话号码:
```java
String phoneRegex = "^(\\+1\\s?)?((\\([2-9]{1}\\d{2}\\)|[2-9]{1}\\d{2})-)?[2-9]{1}\\d{2}-\\d{4}$";
```
在使用时,可以这样进行验证:
```java
public boolean validateEmail(String email) {
Pattern pattern = ***pile(emailRegex);
Matcher matcher = pattern.matcher(email);
return matcher.matches();
}
public boolean validatePhone(String phone) {
Pattern pattern = ***pile(phoneRegex);
Matcher matcher = pattern.matcher(phone);
return matcher.matches();
}
```
### 3.1.2 清洗日志文件中的数据
日志文件是诊断系统问题和性能监控的重要数据源。在分析日志之前,经常需要先对其进行清洗,以去除无用信息,提取关键数据。正则表达式可以高效地完成这一任务。
例如,假设日志文件中记录了每次请求的路径,可以使用如下正则表达式来提取它们:
```java
String logEntry = "2023-03-15 12:10:32 INFO /api/users/list";
String pathRegex = "\"(.*?)\"";
Pattern pattern = ***pile(pathRegex);
Matcher matcher = pattern.matcher(logEntry);
if (matcher.fin
```
0
0
相关推荐
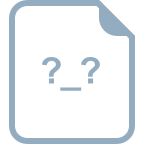
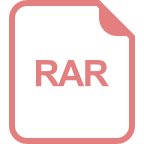
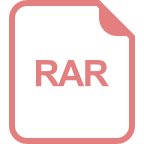





