【自定义字符串格式化】:Java实现技巧与实践
发布时间: 2024-08-29 13:18:07 阅读量: 44 订阅数: 23 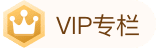
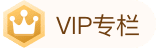
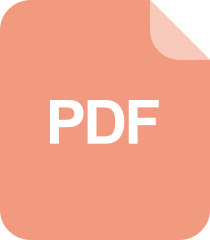
java字符串与格式化输出的深入分析
# 1. 自定义字符串格式化的概念和需求
## 1.1 格式化概述
在软件开发中,字符串格式化是将数据转换成特定格式的文本表示的过程。它对于生成用户友好的输出、记录日志、创建定制的报表等任务至关重要。随着业务逻辑的复杂化,标准库提供的格式化方法有时无法满足特定的需求,因此自定义字符串格式化应运而生。
## 1.2 格式化需求的多样性
自定义格式化的常见需求包括但不限于:
- 处理复杂的日期时间格式。
- 为货币和数字提供特定的显示规则。
- 格式化自定义对象,展示其属性。
- 支持多语言环境下的格式化。
## 1.3 自定义格式化的优点
自定义格式化能够提供更精确的控制,并且可以创建可复用的格式化模式。这样的模式可以被应用在不同的场景中,提高开发效率并保持代码的一致性。它也是应对国际化需求和优化性能的关键。
在下一章,我们将深入探讨Java中的字符串格式化技术,包括标准库提供的方法以及如何实现自定义格式化。
# 2. Java中的字符串格式化技术
## 2.1 Java标准库中的字符串格式化方法
### 2.1.1 使用`String.format`方法
`String.format`方法是Java中实现字符串格式化的传统方式,它提供了一种灵活且强大的方式来构造格式化的字符串。`String.format`方法采用类似于C语言中的`printf`函数的语法,使用格式说明符来指定各种数据类型的格式。
```java
String name = "Alice";
int age = 30;
String formattedString = String.format("Name: %s, Age: %d", name, age);
System.out.println(formattedString);
```
在上面的示例中,`%s`和`%d`分别代表字符串和十进制整数的格式说明符。`String.format`方法的灵活性体现在它能够使用各种不同的格式说明符来满足多样的格式化需求。例如,可以使用`%.2f`来格式化浮点数,保留两位小数。
此外,`String.format`还支持更复杂的格式化场景,如左对齐、右对齐、宽度和精度的指定等:
```java
String formattedString = String.format("|%-10s|%10s|", name, age);
```
在这里,`%-10s`和`%10s`分别表示左对齐和右对齐的字符串,宽度为10个字符。
### 2.1.2 使用`MessageFormat`类
`MessageFormat`类是Java标准库中另一个强大的字符串格式化工具,特别适合于本地化和国际化场景。它允许开发者指定消息模板,并动态插入变量,以支持多语言环境下的格式化需求。
```java
String pattern = "Hello, {0}! You have {1} new messages.";
Object[] params = {"Alice", 5};
String formattedString = MessageFormat.format(pattern, params);
System.out.println(formattedString);
```
`MessageFormat`使用占位符`{0}`, `{1}`等来指定参数的位置,并通过`format`方法替换这些占位符。它的优点在于可以通过更改模式字符串来快速适应不同的语言环境,而不需要改变代码逻辑。
### 性能对比与分析
在进行字符串格式化时,性能是一个重要的考虑因素。`String.format`和`MessageFormat`在性能上存在差异,具体取决于使用场景和格式化操作的复杂性。
在简单的格式化任务中,`String.format`通常比`MessageFormat`更快,因为它的执行路径更直接,没有额外的解析步骤。但当格式化操作涉及大量数据或复杂的模式匹配时,`MessageFormat`的可读性和易用性使其成为更好的选择。
### 性能优化的策略和实践
在处理大量数据时,频繁地调用`String.format`或`MessageFormat`可能会导致性能瓶颈。为了优化性能,可以采用以下策略:
- 缓存频繁使用的格式化模板。
- 避免在循环或性能敏感的代码块中使用字符串格式化。
- 使用更轻量级的格式化方法,比如`StringBuilder`。
```java
StringBuilder sb = new StringBuilder();
sb.append("Name: ").append(name).append(", Age: ").append(age);
String formattedString = sb.toString();
System.out.println(formattedString);
```
在上述代码中,`StringBuilder`是一种更高效的字符串构建方式,尤其适合在循环或频繁调用的场景中使用。
## 2.2 自定义格式化方法的实现
### 2.2.1 实现基本的自定义格式化
在某些场景下,Java标准库提供的格式化功能可能无法满足特定需求。此时,开发者需要实现自定义的格式化方法。这通常涉及到解析字符串模板,并替换其中的变量。
自定义格式化的关键是定义清晰的解析逻辑和替换机制。例如,可以创建一个`SimpleFormatter`类,它使用简单的模板语言来格式化字符串:
```java
public class SimpleFormatter {
public String format(String template, Object... args) {
return parseTemplate(template, args);
}
private String parseTemplate(String template, Object... args) {
// 实现解析和替换逻辑
}
}
```
### 2.2.2 处理复杂格式和国际化的自定义格式化
当格式化需求变得更加复杂,例如需要处理时间、货币、日期等格式时,自定义格式化方法需要扩展以支持这些格式。这可能涉及到集成第三方库,或者使用Java的国际化工具类,如`java.text.NumberFormat`和`java.text.DateFormat`。
对于国际化的支持,自定义格式化方法应该考虑区域设置。例如:
```java
import java.text.SimpleDateFormat;
import java.util.Locale;
public class CustomDateFormatter {
public String formatDate(String pattern, Date date, Locale locale) {
SimpleDateFormat sdf = new SimpleDateFormat(pattern, locale);
return sdf.format(date);
}
}
```
## 2.3 字符串格式化的性能考量
### 2.3.1 性能对比与分析
性能分析是确保软件运行效率的关键环节。开发者需要对不同的格式化方法进行基准测试,以了解它们在不同场景下的表现。基准测试可以揭示诸如内存使用、CPU消耗和执行时间等重要性能指标。
### 2.3.2 性能优化的策略和实践
优化策略的实施应基于性能测试结果,通常涉及以下方面:
- 优化算法,减少不必要的计算。
- 减少对象的创建,特别是在循环或高频调用中。
- 使用高效的缓存机制。
以下是一个使用`StringBuilder`实现的自定义格式化方法的示例:
```java
public class FastFormatter {
public String format(String template, Object... args) {
StringBuilder sb = new StringBuilder(template);
int argIndex = 0;
while (true) {
int startIndex = sb.indexOf("{}", argIndex);
if (startIndex == -1) {
break;
}
if (argIndex >= args.length) {
throw new IllegalArgumentException("Too few arguments for the template");
}
sb.replace(startIndex, startIndex + 2, String.valueOf(args[argIndex++]));
}
return sb.toString();
}
}
```
在这个示例中,`FastFormatter`通过在模板中查找`{}`占位符并替换为参数值,减少了字符串的创建和复制次数,从而提高了性能。
通过本章节的介绍,我们了解了Java中字符串格式化的传统方法和现代实践,探索了性能优化的策略和自定义格式化方法的实现。对于追求性能极致和高度定制化的开发者而言,这些知识是不可或缺的资产。
# 3. 自定义字符串格式化的实践应用
在第三章中,我们将探讨自定义字符串格式化在实际编程场景中的具体应用。格式化字符串可以应用于多种类型的数据,例如日期时间、数字、货币以及自定义对象。在这一章节中,我们将通过实际的代码示例和详细的步骤,展示如何在Java中实现和使用这些高级格式化技术。
## 3.1 格式化日期和时间
格式化日期和时间是软件开发中常见的需求,无论是为了日志记录、用户界面显示还是数据交换,将日期和时间以可读的格式呈现出来都是极其重要的。Java提供了强大的日期和时间API,让我们可以轻松实现复杂的格式化需求。
### 3.1.1 格式化日期时间到特定格式
在Java 8之后,`java.time`包中的`DateTimeFormatter`类成为推荐的方式来格式化日期和时间。下面是一个将日期时间格式化为"年-月-日 时:分:秒"的示例:
```java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeFormattingExample {
public static void main(String[] args) {
LocalDateTime now = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = now.format(formatter);
System.out.println(formattedDateTime);
}
}
```
代码逻辑解释:
- 首先,我们从`LocalDateTime`类获取当前的日期和时间。
- 接着,我们创建了一个`DateTimeFormatter`实例,并通过`ofPattern`方法指定了一个格式化模式。
- 最后,我们使用`format`方法将`LocalDateTime`对象按照指定的格式进行格式化,并输出格式化后的字符串。
参数说明:
- `yyyy`表示四位数的年份。
- `MM`表示月份。
- `dd`表示日。
- `HH`表示小时。
- `mm`表示分钟。
- `ss`表示秒。
### 3.1.2 实现不同时区的时间格式化
在全球化应用中,处理不同时区的时间是必不可少的。`java.time`包中的`ZonedDateTime`类和`ZoneId`类可以帮助我们处理时区相关的时间格式化:
```java
import java.time.ZonedDateTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
public class TimezoneFormattingExample {
public static void main(String[] args) {
ZonedDateTime东京时间 = ZonedDateTime.now(ZoneId.of("Asia/Tokyo"));
DateTimeForm
```
0
0
相关推荐
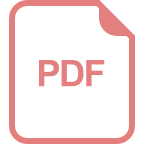
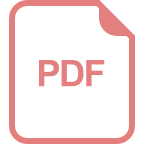
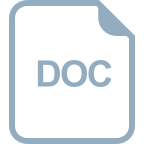
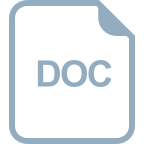
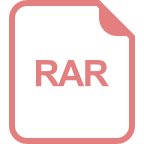
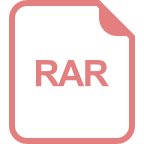
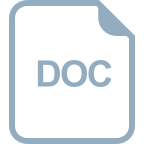