package step2; import java.util.ArrayList; import java.util.Iterator; public class WalkCollection { public static void main(String[] args) { WalkCollection_src walkCollection_src = new WalkCollection_src(); ArrayList arrayListExample = walkCollection_src.getArrayListExample(); // ---------------------Begin------------------------ //使用迭代器iterator遍历arrayListExample集合 //使用foreach遍历arrayListExample集合 // ---------------------End------------------------ } }
时间: 2023-08-15 12:13:34 浏览: 178
下面是遍历arrayListExample集合的代码:
```java
//使用迭代器iterator遍历arrayListExample集合
Iterator iterator = arrayListExample.iterator();
while(iterator.hasNext()){
Object obj = iterator.next();
System.out.println(obj);
}
//使用foreach遍历arrayListExample集合
for(Object obj : arrayListExample){
System.out.println(obj);
}
```
以上两种方式都可以遍历ArrayList集合,不同之处在于使用迭代器可以在遍历时进行删除等操作,而使用foreach则不能进行修改操作。
相关问题
声明一个 Student 类,属性包括姓名、学号、总成绩;生成 10 个 Student 类对象,并放在一个一维数组中,编写方法按总成绩进行排序,将排序后的对象 分别保持在Vector 、ArrayList、 HashTable类型的对象中,并遍历显示其中 元素的信息。 程序设计参考框架如下: import java,util.Vector; import java.util.ArrayList; import java.util.Hashtable; import java.util.Enumeration; import java.util.Iterator; public class Ex71 { public static void main (String[] args){
public class Student implements Comparable<Student>{
private String name;
private int id;
private int totalScore;
public Student(String name, int id, int totalScore){
this.name = name;
this.id = id;
this.totalScore = totalScore;
}
public String getName(){
return name;
}
public int getId(){
return id;
}
public int getTotalScore(){
return totalScore;
}
public void setName(String name){
this.name = name;
}
public void setId(int id){
this.id = id;
}
public void setTotalScore(int totalScore){
this.totalScore = totalScore;
}
public int compareTo(Student s){
return this.totalScore - s.totalScore;
}
}
import java.util.Vector;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.Enumeration;
import java.util.Iterator;
public class Ex71 {
public static void main (String[] args){
Student[] students = new Student[10];
students[0] = new Student("Tom", 1001, 80);
students[1] = new Student("Jerry", 1002, 90);
students[2] = new Student("Alice", 1003, 85);
students[3] = new Student("Bob", 1004, 70);
students[4] = new Student("Peter", 1005, 95);
students[5] = new Student("Mary", 1006, 65);
students[6] = new Student("John", 1007, 75);
students[7] = new Student("Lucy", 1008, 88);
students[8] = new Student("David", 1009, 92);
students[9] = new Student("Lily", 1010, 78);
// sort by total score
Arrays.sort(students);
// put sorted objects into Vector
Vector<Student> vector = new Vector<Student>();
for(Student s : students){
vector.add(s);
}
// put sorted objects into ArrayList
ArrayList<Student> arrayList = new ArrayList<Student>();
for(Student s : students){
arrayList.add(s);
}
// put sorted objects into Hashtable
Hashtable<Integer, Student> hashtable = new Hashtable<Integer, Student>();
int i = 1;
for(Student s : students){
hashtable.put(i++, s);
}
// display elements in Vector
System.out.println("Elements in Vector:");
Enumeration<Student> enumeration = vector.elements();
while(enumeration.hasMoreElements()){
Student s = enumeration.nextElement();
System.out.println("Name: " + s.getName() + ", ID: " + s.getId() + ", Total Score: " + s.getTotalScore());
}
// display elements in ArrayList
System.out.println("Elements in ArrayList:");
Iterator<Student> iterator = arrayList.iterator();
while(iterator.hasNext()){
Student s = iterator.next();
System.out.println("Name: " + s.getName() + ", ID: " + s.getId() + ", Total Score: " + s.getTotalScore());
}
// display elements in Hashtable
System.out.println("Elements in Hashtable:");
Enumeration<Integer> keys = hashtable.keys();
while(keys.hasMoreElements()){
Integer key = keys.nextElement();
Student s = hashtable.get(key);
System.out.println("Name: " + s.getName() + ", ID: " + s.getId() + ", Total Score: " + s.getTotalScore());
}
}
}
1.完善下列代码用两种方法遍历list集合 import java.util.ArrayList; import java.util.Iterator; import java.util.List; public class AccessingList { public static void main(String[] args) { List<String> list = new ArrayList<String> (); list.add("A"); list.add("B"); list.add("C"); // 第一种遍历方法:forEach循环遍历 // 第二种遍历方法:迭代器遍历 } }
第一种遍历方法:forEach循环遍历
```
for (String s : list) {
System.out.println(s);
}
```
第二种遍历方法:迭代器遍历
```
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String s = iterator.next();
System.out.println(s);
}
```
阅读全文
相关推荐
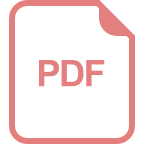
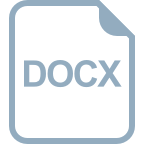
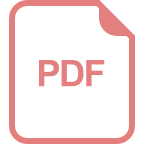













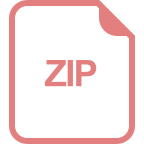