用java编写一个碟包,需要能保存CD和DVD碟片,记录碟片的发行信息,有存入、取出、外借、归还、点数、遍历等功能的程序
时间: 2024-05-21 10:13:14 浏览: 81
很高兴回答你的问题。您可以使用Java编写一个碟包程序,其中包括保存CD和DVD碟片、记录碟片的发行信息、存入、取出、外借、归还、点数、遍历等功能。您可以使用Java的面向对象编程来实现这些功能,例如创建一个Disc类来表示CD和DVD碟片,使用HashMap来记录碟片的发行信息,以及使用ArrayList来存储和管理碟片。如果您需要更具体的代码实现,请告诉我,我会尽力帮助您。
相关问题
JAVA代码题 创建一个碟包,需要能保存CD和DVD碟片,记录碟片的发行信息,有存入、取出、外借、归还、点数、遍历等功能。
以下是一个简单的Java代码实现,包含了所述的基本功能:
// 碟片类
class Disc {
private String title; // 碟片名称
private String artist; // 艺术家/演员
private String genre; // 风格/流派
private int year; // 发行年份
private boolean isLent; // 是否借出
private String borrower; // 借出人
public Disc(String title, String artist, String genre, int year) {
this.title = title;
this.artist = artist;
this.genre = genre;
this.year = year;
this.isLent = false;
this.borrower = null;
}
public String getTitle() {
return title;
}
public String getArtist() {
return artist;
}
public String getGenre() {
return genre;
}
public int getYear() {
return year;
}
public boolean isLent() {
return isLent;
}
public void lend(String borrower) {
if (isLent) {
System.out.println("This disc is already lent out!");
} else {
isLent = true;
this.borrower = borrower;
System.out.println("Successfully lent out to " + borrower);
}
}
public void returnDisc() {
if (isLent) {
isLent = false;
borrower = null;
System.out.println("Successfully returned!");
} else {
System.out.println("This disc is not currently lent out.");
}
}
public void printDetails() {
System.out.println("Title: " + title);
System.out.println("Artist: " + artist);
System.out.println("Genre: " + genre);
System.out.println("Year: " + year);
if (isLent) {
System.out.println("Currently lent out to: " + borrower);
} else {
System.out.println("Currently available.");
}
}
}
// 碟包类
class DiscPackage {
private ArrayList<Disc> discs;
public DiscPackage() {
discs = new ArrayList<Disc>();
}
public void addDisc(Disc disc) {
discs.add(disc);
System.out.println("Successfully added disc: " + disc.getTitle());
}
public void removeDisc(Disc disc) {
if (discs.remove(disc)) {
System.out.println("Successfully removed disc: " + disc.getTitle());
} else {
System.out.println("Disc not found in package.");
}
}
public Disc findDisc(String title) {
for (Disc disc : discs) {
if (disc.getTitle().equals(title)) {
return disc;
}
}
return null;
}
public void lendDisc(String title, String borrower) {
Disc disc = findDisc(title);
if (disc != null) {
disc.lend(borrower);
} else {
System.out.println("Disc not found in package.");
}
}
public void returnDisc(String title) {
Disc disc = findDisc(title);
if (disc != null) {
disc.returnDisc();
} else {
System.out.println("Disc not found in package.");
}
}
public void printDetails() {
if (discs.size() == 0) {
System.out.println("The disc package is empty.");
} else {
System.out.println("Disc package contents:");
for (Disc disc : discs) {
disc.printDetails();
System.out.println();
}
}
}
}
// 测试代码
public class Main {
public static void main(String[] args) {
Disc cd = new Disc("The Dark Side of the Moon", "Pink Floyd", "Progressive rock", 1973);
Disc dvd = new Disc("The Lord of the Rings: The Fellowship of the Ring", "Peter Jackson", "Fantasy", 2001);
DiscPackage package1 = new DiscPackage();
package1.addDisc(cd);
package1.addDisc(dvd);
package1.printDetails();
package1.lendDisc("The Dark Side of the Moon", "John Smith");
package1.lendDisc("The Dark Side of the Moon", "Jane Doe");
package1.printDetails();
package1.returnDisc("The Dark Side of the Moon");
package1.returnDisc("The Dark Side of the Moon");
package1.printDetails();
}
}
Java代码题 创建一个碟包,需要能保存CD和DVD碟片,记录碟片的发行信息,有存入、取出、外借、归还、点数、遍历等功能。
public class DiscPackage {
private List<Disc> discs;
public DiscPackage() {
discs = new ArrayList<>();
}
public void addDisc(Disc disc) {
discs.add(disc);
}
public Disc getDisc(String title) {
for (Disc disc : discs) {
if (disc.getTitle().equals(title)) {
return disc;
}
}
return null;
}
public void removeDisc(String title) {
for (int i = 0; i < discs.size(); i++) {
if (discs.get(i).getTitle().equals(title)) {
discs.remove(i);
break;
}
}
}
public void lendDisc(String title) {
Disc disc = getDisc(title);
if (disc != null && !disc.isLent()) {
disc.setLent(true);
}
}
public void returnDisc(String title) {
Disc disc = getDisc(title);
if (disc != null && disc.isLent()) {
disc.setLent(false);
}
}
public int getTotalPoints() {
int totalPoints = 0;
for (Disc disc : discs) {
totalPoints += disc.getPoints();
}
return totalPoints;
}
public void printDiscs() {
for (Disc disc : discs) {
System.out.println(disc);
}
}
}
public abstract class Disc {
private String title;
private String artist;
private int releaseYear;
private boolean lent;
private int points;
public Disc(String title, String artist, int releaseYear, int points) {
this.title = title;
this.artist = artist;
this.releaseYear = releaseYear;
this.points = points;
this.lent = false;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getArtist() {
return artist;
}
public void setArtist(String artist) {
this.artist = artist;
}
public int getReleaseYear() {
return releaseYear;
}
public void setReleaseYear(int releaseYear) {
this.releaseYear = releaseYear;
}
public boolean isLent() {
return lent;
}
public void setLent(boolean lent) {
this.lent = lent;
}
public int getPoints() {
return points;
}
public void setPoints(int points) {
this.points = points;
}
@Override
public String toString() {
return "Title: " + title + ", Artist: " + artist + ", Release Year: " + releaseYear + ", Lent: " + lent + ", Points: " + points;
}
}
public class CD extends Disc {
private int numTracks;
public CD(String title, String artist, int releaseYear, int points, int numTracks) {
super(title, artist, releaseYear, points);
this.numTracks = numTracks;
}
public int getNumTracks() {
return numTracks;
}
public void setNumTracks(int numTracks) {
this.numTracks = numTracks;
}
@Override
public String toString() {
return "CD - " + super.toString() + ", Number of Tracks: " + numTracks;
}
}
public class DVD extends Disc {
private int duration;
public DVD(String title, String artist, int releaseYear, int points, int duration) {
super(title, artist, releaseYear, points);
this.duration = duration;
}
public int getDuration() {
return duration;
}
public void setDuration(int duration) {
this.duration = duration;
}
@Override
public String toString() {
return "DVD - " + super.toString() + ", Duration: " + duration;
}
}
相关推荐
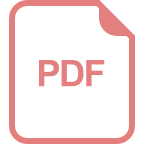
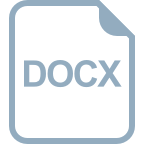
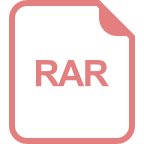
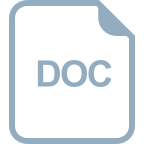
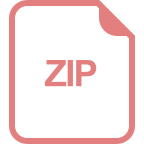