c语言中的sin函数用法
时间: 2024-10-27 11:09:50 浏览: 17
在C语言中,`sin()` 函数是一个数学库函数,它属于`math.h`头文件的一部分。这个函数用于计算给定角度(通常以弧度为单位)的正弦值。其基本语法如下:
```c
#include <math.h> // 引入math库
double sin(double angle);
```
`sin(angle)` 中,`angle` 参数是要转换的角度,返回的是一个 `double` 类型的结果,表示该角度对应的正弦数值,范围从 -1 到 1。
例如,如果你想要计算45度角的正弦值,可以这样做:
```c
#include <stdio.h>
#include <math.h>
int main() {
double angle_radians = M_PI / 4; // 将角度转换为弧度 (45度)
double result = sin(angle_radians);
printf("The sine of %f degrees is %f\n", angle_radians * 180/M_PI, result);
return 0;
}
```
相关问题
c语言sin函数和cos函数怎么用
在C语言中,`sin()` 和 `cos()` 函数是数学库中的标准函数,属于 `math.h` 库的一部分,用于计算正弦和余弦值。这两个函数需要一个弧度值作为输入参数。
以下是它们的基本使用方法:
```c
#include <stdio.h>
#include <math.h> // 引入 math.h 包含 sin() 和 cos() 函数
int main() {
double angle_in_degrees; // 角度
double angle_in_radians; // 弧度值
double sin_value, cos_value;
// 将角度转换为弧度(π rad ≈ 180°)
angle_in_degrees = 45; // 例如求45度角的正弦值
angle_in_radians = M_PI * (angle_in_degrees / 180.0);
// 计算正弦和余弦值
sin_value = sin(angle_in_radians);
cos_value = cos(angle_in_radians);
printf("sin(45°) = %.6lf\n", sin_value);
printf("cos(45°) = %.6lf\n", cos_value);
return 0;
}
```
在这个例子中,我们首先将给定的角度转换为弧度,然后调用 `sin()` 和 `cos()` 来计算对应的值,并打印结果。
C语言 sin函数的多种写法
1. 使用math.h库函数:使用标准库math.h中的sin函数,语法为sin(x),其中x为弧度值。
2. 泰勒级数展开:sin函数可以通过泰勒级数展开进行计算,即sin(x) = x - x^3/3! + x^5/5! - x^7/7! + ...,其中!表示阶乘,可以使用循环结构进行计算。
3. 牛顿迭代法:使用牛顿迭代法可以较快地求解sin函数,具体方法为:设f(x) = sin(x) - a,其中a为要求的sin函数值,令f(x) = 0,由牛顿迭代公式x = x0 - f(x0)/f'(x0),可以得到sin函数值。
4. 三角恒等式:使用三角恒等式sin(x) = cos(pi/2 - x)进行计算。
5. 查表法:将sin函数在一定范围内的取值预先计算出来并存储在表格中,需要求解时直接查表即可。
阅读全文
相关推荐
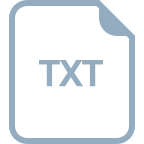
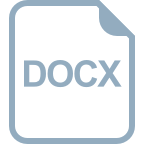
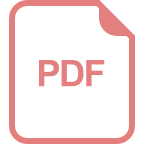
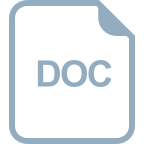
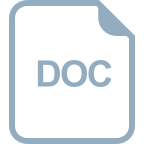
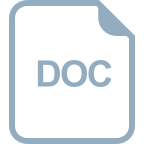
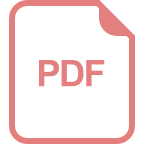
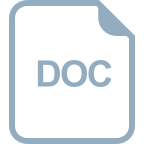
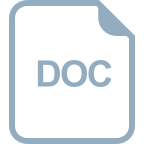
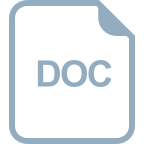






