netty中Fegin调用 InheritableThreadLocal丢失缓存
时间: 2023-12-06 18:32:52 浏览: 35
在Netty中使用Feign调用时,可能会遇到InheritableThreadLocal丢失缓存的问题。这是因为Netty使用了线程池来处理请求,而线程池会重用线程。InheritableThreadLocal是线程继承的一种机制,可以在子线程中访问父线程中设置的值,但是在线程池中,父线程的值并不会自动传递给子线程。
为了解决这个问题,可以在调用Feign之前,手动将InheritableThreadLocal的值设置到子线程中。可以使用Runnable或者Callable的方式,在提交任务到线程池之前,将InheritableThreadLocal的值传递给子线程。例如:
```java
Runnable task = () -> {
InheritableThreadLocalValue value = InheritableThreadLocal.get();
// 在子线程中使用value进行操作
};
// 将InheritableThreadLocal的值传递给子线程
InheritableThreadLocalValue parentValue = InheritableThreadLocal.get();
executorService.submit(() -> {
InheritableThreadLocal.set(parentValue);
task.run();
});
```
这样就能确保子线程中能够访问到正确的InheritableThreadLocal的值。
相关问题
java使用netty模拟rpc调用
使用Netty模拟RPC调用需要先了解RPC的基本概念和原理,以及Netty框架的使用方法。
RPC(Remote Procedure Call)远程过程调用是一种通过网络从远程计算机程序上请求服务,而不需要了解底层网络技术的协议。它允许程序调用另一个地址空间(通常是共享网络的另一台机器上)的过程或函数,而不用显式地编写远程调用的代码。RPC通常基于客户端/服务器模型,客户端向服务器发送RPC请求,服务器响应请求并返回结果。
Netty是一个高性能的、异步的、事件驱动的网络编程框架,它可以轻松地实现RPC调用。
下面是一个简单的Java代码示例,演示如何使用Netty模拟RPC调用:
1. 首先需要定义一个接口,这个接口定义了要远程调用的方法:
```java
public interface HelloService {
String sayHello(String name);
}
```
2. 接下来创建一个实现类,实现HelloService接口:
```java
public class HelloServiceImpl implements HelloService {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
```
3. 创建一个服务端程序,启动Netty服务端,并将HelloServiceImpl注册到服务端:
```java
public class Server {
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectDecoder(ClassResolvers.cacheDisabled(null)));
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ServerHandler());
}
});
ChannelFuture f = b.bind(8888).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
private static class ServerHandler extends SimpleChannelInboundHandler<Object> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, Object msg) throws Exception {
if (msg instanceof RpcRequest) {
RpcRequest request = (RpcRequest) msg;
String className = request.getClassName();
String methodName = request.getMethodName();
Class<?>[] parameterTypes = request.getParameterTypes();
Object[] parameters = request.getParameters();
// 根据类名获取实现类
Class<?> clazz = Class.forName(className);
Object service = clazz.newInstance();
// 根据方法名和参数类型获取方法
Method method = clazz.getMethod(methodName, parameterTypes);
// 执行方法
Object result = method.invoke(service, parameters);
// 返回结果
ctx.writeAndFlush(result);
}
}
}
}
```
4. 创建一个客户端程序,通过Netty客户端向服务端发送RPC请求:
```java
public class Client {
public static void main(String[] args) throws Exception {
EventLoopGroup group = new NioEventLoopGroup();
try {
Bootstrap b = new Bootstrap();
b.group(group)
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new ObjectEncoder());
pipeline.addLast(new ObjectDecoder(ClassResolvers.cacheDisabled(null)));
pipeline.addLast(new ClientHandler());
}
});
ChannelFuture f = b.connect("localhost", 8888).sync();
// 发送RPC请求
RpcRequest request = new RpcRequest();
request.setClassName("com.example.HelloServiceImpl");
request.setMethodName("sayHello");
request.setParameterTypes(new Class<?>[] { String.class });
request.setParameters(new Object[] { "world" });
f.channel().writeAndFlush(request);
// 等待响应
f.channel().closeFuture().sync();
} finally {
group.shutdownGracefully();
}
}
private static class ClientHandler extends SimpleChannelInboundHandler<Object> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, Object msg) throws Exception {
// 处理响应结果
System.out.println(msg);
ctx.channel().close();
}
}
}
```
这样,我们就通过Netty模拟了一次RPC调用。当客户端向服务端发送RPC请求时,服务端会根据请求参数调用相应的方法并返回结果,客户端收到响应结果后输出到控制台。
netty框架怎么调用webSocket服务方法
使用Netty框架调用WebSocket服务方法的步骤如下:
1. 导入相关依赖
在Maven的pom.xml文件中加入以下依赖:
```
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.42.Final</version>
</dependency>
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-transport-native-epoll</artifactId>
<version>4.1.42.Final</version>
<classifier>linux-x86_64</classifier>
</dependency>
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-transport-native-kqueue</artifactId>
<version>4.1.42.Final</version>
<classifier>osx-x86_64</classifier>
</dependency>
```
2. 编写WebSocket服务类
创建一个类来实现WebSocket服务,使用Netty的WebSocketServerHandler类来处理WebSocket消息。在这个类中,我们需要实现以下方法:
```
public class WebSocketServer extends SimpleChannelInboundHandler<WebSocketFrame> {
// 连接建立时触发
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
// TODO
}
// 连接关闭时触发
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
// TODO
}
// 处理WebSocket帧消息
@Override
protected void channelRead0(ChannelHandlerContext ctx, WebSocketFrame msg) throws Exception {
// TODO
}
// 异常处理
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
// TODO
}
}
```
3. 启动WebSocket服务
在main方法中启动WebSocket服务:
```
public static void main(String[] args) throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new ChunkedWriteHandler());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new WebSocketServerProtocolHandler("/websocket"));
pipeline.addLast(new WebSocketServer());
}
});
ChannelFuture f = bootstrap.bind(8080).sync();
f.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
```
4. 调用WebSocket服务方法
使用WebSocket客户端来调用WebSocket服务方法:
```
WebSocketClient client = new WebSocketClient();
client.connect(new URI("ws://localhost:8080/websocket"), new WebSocketHandler() {
@Override
public void onOpen(ServerHandshake handshake) {
// 连接建立时触发
}
@Override
public void onMessage(String message) {
// 收到消息时触发
}
@Override
public void onClose(int code, String reason, boolean remote) {
// 连接关闭时触发
}
@Override
public void onError(Exception ex) {
// 异常处理
}
});
client.send("hello world"); // 调用WebSocket服务方法
```
相关推荐
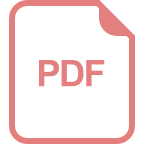












