#include <stdio.h> #include<stdlib.h> void input(int *&a,int & n); void output(int *a,int n); void swap(int &a, int &b); int Partition(int a[],int low,int high); void QuickSort(int a[],int s,int t); int n; int main () { int i; int *a = NULL; input (a,n); QuickSort(a, 0, n-1) ; free(a); return 0; } void input(int *&a,int & n) { int i; scanf("%d",&n); if((a=(int *) malloc ((n)*sizeof(int)))==NULL) { printf("不能成功分配内存单元\n"); exit(0); } for(i=0;i<n;i++) { scanf("%d",&a[i]); } } void output(int *a,int n) { int i; for(i=0;i<n;i++) { printf("%d ",a[i]); } printf("\n"); } void swap(int &a, int &b) { int t; t=a;a=b;b=t; } /**********定义Partition() 和 QuickSort()函数**********/ /********** Begin **********/ int Partition(int a[], int low, int high) { int pivot = a[low]; while (low < high) { while (low < high && a[high] >= pivot) { high--; } a[low] = a[high]; while (low < high && a[low] <= pivot) { low++; } a[high] = a[low]; } a[low] = pivot; return low; } void QuickSort(int a[], int s, int t) { if (s < t) { int pos = Partition(a, s, t); QuickSort(a, s, pos - 1); QuickSort(a, pos + 1, t); } } /********** End **********/输出每次划分后数组的值
时间: 2023-08-28 19:16:31 浏览: 55
很好,下面是代码实现:
```
void PrintPartition(int a[], int low, int high) {
for (int i = low; i <= high; i++) {
printf("%d ", a[i]);
}
printf("\n");
}
void QuickSort(int a[], int s, int t) {
if (s < t) {
int pos = Partition(a, s, t);
PrintPartition(a, s, t); // 打印每次划分后的数组值
QuickSort(a, s, pos - 1);
QuickSort(a, pos + 1, t);
}
}
```
在`QuickSort`函数中加入了`PrintPartition`函数,在每次划分后打印数组的值。
相关问题
#include <stdio.h> #include<stdlib.h> #include<time.h> //下面两个函数与第一关第二关的功能相同 void input(int *&a,
#include <stdio.h> 是C语言中的头文件之一,它包含了一些输入输出相关的函数。例如,printf() 和 scanf() 这两个常用函数就包含在这个头文件中。
#include<stdlib.h> 是C语言中的头文件之一,它包含了一些与内存分配、进程控制以及其他实用函数相关的内容。例如,malloc() 和 free() 就是两个常用的内存分配函数。
#include<time.h> 是C语言中的头文件之一,它包含了一些关于时间和日期操作的函数。例如,time() 函数就可以获取当前的系统时间。
函数 input(int *&a, ...) 的具体实现我无法确定,因为缺少完整的代码。但是,从函数名 input() 可以猜测这是一个用于输入数据的函数。
至于第一关和第二关,我并不清楚具体指的是哪些内容,请提供更详细的信息。我会尽力回答您的问题。
在c语言中使用#include <stdio.h> #include <stdlib.h> #include <string.h> #include<wchar.h> #include<locale.h> #include <wctype.h>等库函数实现英文标点转换成中文标点
在C语言中,可以使用以下库函数来实现英文标点转换成中文标点:
1. `setlocale()`函数:用于设置程序的本地化环境,包括字符编码和区域设置。在这个问题中,我们需要将本地化环境设置为中文,以便正确地处理中文字符。
2. `wctomb()`函数:用于将宽字符转换为多字节字符。我们可以使用这个函数将宽字符的中文标点转换为对应的多字节字符。
下面是一个示例代码,演示如何使用上述库函数实现英文标点转换成中文标点:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <wchar.h>
#include <locale.h>
#include <wctype.h>
void convertPunctuation(const wchar_t* input, wchar_t* output) {
setlocale(LC_ALL, ""); // 设置本地化环境为中文
while (*input != L'\0') {
if (iswpunct(*input)) { // 判断是否为英文标点
char mbChar[MB_CUR_MAX];
wctomb(mbChar, *input); // 将宽字符转换为多字节字符
strcat(output, mbChar); // 将多字节字符拼接到输出字符串中
} else {
wcscat(output, input); // 将非英文标点的宽字符直接拼接到输出字符串中
}
input++;
}
}
int main() {
const wchar_t* input = L"Hello, world! This is a test.";
wchar_t output[100] = L"";
convertPunctuation(input, output);
wprintf(L"转换后的字符串:%ls\n", output);
return 0;
}
```
在上面的示例代码中,我们定义了一个`convertPunctuation()`函数,它接受一个宽字符数组作为输入,并将转换后的字符串存储在另一个宽字符数组中。在函数内部,我们使用`iswpunct()`函数来判断是否为英文标点,如果是,则使用`wctomb()`函数将宽字符转换为多字节字符,并将其拼接到输出字符串中;如果不是英文标点,则直接将宽字符拼接到输出字符串中。
在`main()`函数中,我们定义了一个示例输入字符串`input`,并将其传递给`convertPunctuation()`函数进行转换。最后,我们使用`wprintf()`函数打印转换后的字符串。
请注意,以上代码仅适用于将英文标点转换为中文标点。如果需要处理其他字符或更复杂的转换规则,可能需要使用更高级的技术或库函数。
相关推荐
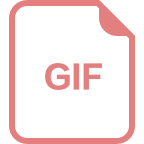
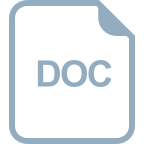
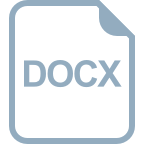














