java从FTP(FileZilla Server)上传文件
时间: 2024-01-30 07:44:50 浏览: 86
以下是使用Java实现从FTP服务器(例如FileZilla Server)上传文件的代码示例:
```java
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
public class FTPUploader {
public static void main(String[] args) {
String server = "ftp.example.com";
int port = 21;
String user = "username";
String pass = "password";
String localFilePath = "C:/Users/Username/Documents/file.txt";
String remoteFilePath = "/public_html/file.txt";
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(user, pass);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
FileInputStream inputStream = new FileInputStream(localFilePath);
ftpClient.storeFile(remoteFilePath, inputStream);
inputStream.close();
ftpClient.logout();
} catch (IOException ex) {
System.out.println("Error: " + ex.getMessage());
ex.printStackTrace();
} finally {
try {
if (ftpClient.isConnected()) {
ftpClient.disconnect();
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
}
```
在上述代码中,需要修改以下变量:
- `server`:FTP服务器地址。
- `port`:FTP服务器端口号,默认为21。
- `user`:登录FTP服务器的用户名。
- `pass`:登录FTP服务器的密码。
- `localFilePath`:要上传的本地文件的路径。
- `remoteFilePath`:要上传到FTP服务器的远程文件路径。
注意,以上代码使用了Apache Commons Net库,需要先将其添加到项目依赖中。
阅读全文
相关推荐

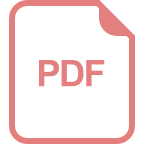
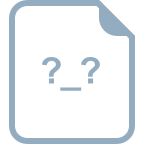
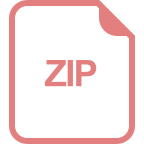
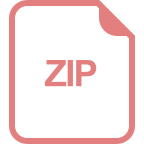
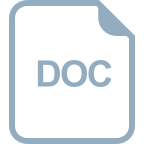
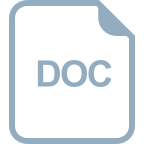
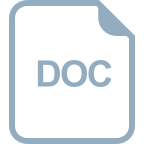


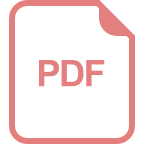
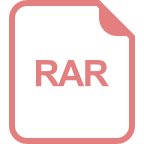
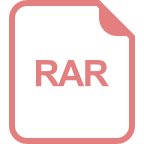
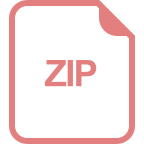
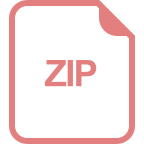
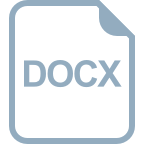