java 并发模拟 工具类
时间: 2024-01-09 11:05:05 浏览: 94
以下是Java并发模拟工具类的介绍和示例:
1. CountDownLatch(线程计数器)
CountDownLatch是一个同步辅助类,它允许一个或多个线程等待其他线程完成操作后再继续执行。它通过一个计数器来实现,计数器的初始值可以设置为线程的数量,每个线程完成任务时,计数器的值减1,当计数器的值为0时,等待的线程将被唤醒。
```java
import java.util.concurrent.CountDownLatch;
public class CountDownLatchDemo {
public static void main(String[] args) throws InterruptedException {
int threadCount = 5;
CountDownLatch latch = new CountDownLatch(threadCount);
for (int i = 0; i < threadCount; i++) {
Thread thread = new Thread(new Worker(latch));
thread.start();
}
latch.await(); // 等待所有线程完成任务
System.out.println("All threads have completed their tasks.");
}
static class Worker implements Runnable {
private final CountDownLatch latch;
public Worker(CountDownLatch latch) {
this.latch = latch;
}
@Override
public void run() {
// 模拟线程执行任务
try {
Thread.sleep(1000);
System.out.println("Thread " + Thread.currentThread().getId() + " has completed its task.");
} catch (InterruptedException e) {
e.printStackTrace();
}
latch.countDown(); // 完成任务后计数器减1
}
}
}
```
2. CyclicBarrier(回环栅栏)
CyclicBarrier是一个同步辅助类,它允许一组线程互相等待,直到所有线程都到达某个公共屏障点。当所有线程都到达屏障点后,屏障点上的任务将被执行。
```java
import java.util.concurrent.BrokenBarrierException;
import java.util.concurrent.CyclicBarrier;
public class CyclicBarrierDemo {
public static void main(String[] args) {
int threadCount = 3;
CyclicBarrier barrier = new CyclicBarrier(threadCount, () -> {
System.out.println("All threads have reached the barrier.");
});
for (int i = 0; i < threadCount; i++) {
Thread thread = new Thread(new Worker(barrier));
thread.start();
}
}
static class Worker implements Runnable {
private final CyclicBarrier barrier;
public Worker(CyclicBarrier barrier) {
this.barrier = barrier;
}
@Override
public void run() {
// 模拟线程执行任务
try {
Thread.sleep(1000);
System.out.println("Thread " + Thread.currentThread().getId() + " has reached the barrier.");
barrier.await(); // 等待其他线程到达屏障点
System.out.println("Thread " + Thread.currentThread().getId() + " continues to execute.");
} catch (InterruptedException | BrokenBarrierException e) {
e.printStackTrace();
}
}
}
}
```
阅读全文
相关推荐




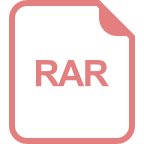













