定义一个js函数,返回两个值,一个返回随机16进制的颜色,一个返回随机rgb颜色,只能使用一个函数返回,用数组接收
时间: 2024-09-26 15:16:04 浏览: 50
可以创建一个JavaScript函数,使用`Math.random()`生成随机数,并结合字符串处理来生成随机16进制颜色和RGB颜色。下面是一个示例函数,它将这两个值作为数组返回:
```javascript
function getRandomColor() {
// 随机16进制颜色
const hexColor = '#'+ Math.floor(Math.random()*16777215).toString(16);
// 将16进制转换为RGB
const rgbColor = `rgb(${Math.floor(Math.random() * 256), Math.floor(Math.random() * 256), Math.floor(Math.random() * 256)})`;
// 返回包含两个颜色的数组
return [hexColor, rgbColor];
}
```
当你调用这个函数时,例如 `const result = getRandomColor();`,`result` 就会是一个包含两个随机颜色的数组,如`['#abcdef', 'rgb(100, 200, 300)']`。
相关问题
定义一个js函数,返回两个值,一个返回随机16进制的颜色,一个返回随机rgb颜色
在JavaScript中,你可以创建一个函数,利用`Math.random()`生成随机数并转换为16进制或RGB颜色。下面是一个简单的示例:
```javascript
function getRandomColor() {
// 随机16进制颜色 (FF0000 - FF00FF)
function randomHexColor() {
return '#' + Math.floor(Math.random() * 16777215).toString(16);
}
// 随机RGB颜色 (0-255)
function randomRgbColor() {
return 'rgb(' +
Math.floor(Math.random() * 256) + ', ' +
Math.floor(Math.random() * 256) + ', ' +
Math.floor(Math.random() * 256) +
')';
}
// 返回随机16进制颜色和RGB颜色
const hexColor = randomHexColor();
const rgbColor = randomRgbColor();
return { hex: hexColor, rgb: rgbColor };
}
// 调用函数获取颜色
const colors = getRandomColor();
console.log(colors.hex); // 输出随机16进制颜色
console.log(colors.rgb); // 输出随机RGB颜色
16进制随机颜色, 题目描述:使用Math对象,制作一个16进制的随机颜色 题目提示:16进制包括 “ 0 1 2 3 4 5 6 7 8 9 A B C D E F ” 例: “#f23a4b”
在编程中,生成一个16进制随机颜色通常涉及创建一个包含随机数的字符串,其中每个字符代表RGB颜色空间的十六进制值。你可以按照以下步骤使用JavaScript(因为Math对象在JavaScript中可用于生成随机数)来完成这个任务:
```javascript
function getRandomColor() {
// 定义16进制字符集
const hexChars = '0123456789ABCDEF';
// 生成三个随机的1到15之间的整数,分别对应RGB的红、绿、蓝分量
let red = Math.floor(Math.random() * 16);
let green = Math.floor(Math.random() * 16);
let blue = Math.floor(Math.random() * 16);
// 将每个分量转换为两位的16进制字符串并拼接在一起
return '#' + hexChars[red] + hexChars[red] + // 红色
hexChars[green] + hexChars[green] + // 绿色
hexChars[blue] + hexChars[blue]; // 蓝色
}
// 调用函数获取随机颜色
let randomHexColor = getRandomColor();
console.log(randomHexColor);
```
阅读全文
相关推荐








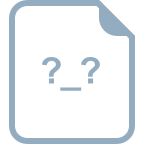







