1.运用urllib进行网络GET请求 2.运用urllib进行网络POST请求 3.运用urllib进行网络超时处理 4.运用urllib进行网络爬虫伪装绕过目标网页检测 5.对课堂讲解的爬虫程序异常捕获代码进行优化 6.随寻找一条新闻,抓取到该新闻的标题并输出结果
时间: 2024-10-08 09:27:12 浏览: 24
1. 使用Python的`urllib`库进行GET请求的基本步骤如下:
```python
import urllib.request
url = 'http://example.com'
response = urllib.request.urlopen(url)
data = response.read().decode('utf-8')
print(data) # 输出服务器返回的数据
```
2. 对于POST请求,需要利用`urllib.request.Request`来构建请求对象,然后传递给`urlopen`方法:
```python
data = {'key': 'value'}
post_data = urllib.parse.urlencode(data).encode('utf-8')
req = urllib.request.Request(url, post_data, method='POST')
with urllib.request.urlopen(req) as response:
print(response.read())
```
3. 超时处理通常通过设置`timeout`参数实现,如设置10秒超时:
```python
try:
response = urllib.request.urlopen(url, timeout=10)
except urllib.error.URLError as e:
if hasattr(e, "code"):
print(f"连接失败,错误码:{e.code}")
elif hasattr(e, "reason"):
print(f"连接失败,原因:{e.reason}")
```
4. 网络爬虫伪装可以通过修改User-Agent等头部信息来实现,例如:
```python
headers = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
request = urllib.request.Request(url, headers=headers)
```
5. 异常捕获优化,可以使用try-except-finally结构,确保资源关闭:
```python
try:
response = urllib.request.urlopen(url)
except Exception as e:
print("发生异常:", str(e))
finally:
response.close()
```
6. 抓取新闻标题的示例,这里假设我们已经解析了HTML文档找到标题元素:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
title_element = soup.find('h1') or soup.find('title') # 根据实际HTML结构找标题
news_title = title_element.text.strip() if title_element else None
print("新闻标题:", news_title)
```
相关推荐
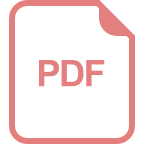
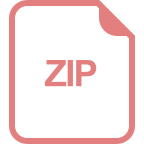


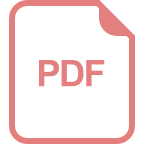
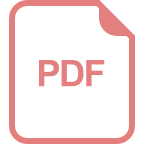
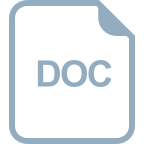
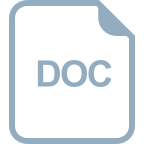
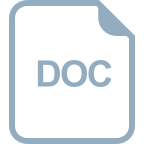
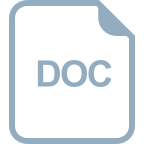
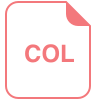
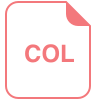
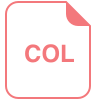



