gec6818开发板电子相册链表
时间: 2023-08-26 22:04:41 浏览: 204
要在GEC6818开发板上实现电子相册功能,可以使用链表数据结构来管理照片。以下是一个简单的示例代码,演示了如何使用链表来存储照片信息:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 照片结构体
typedef struct Photo {
char name[50];
char date[20];
struct Photo* next;
} Photo;
// 创建一个新的照片节点
Photo* createPhoto(char name[], char date[]) {
Photo* photo = (Photo*)malloc(sizeof(Photo));
strcpy(photo->name, name);
strcpy(photo->date, date);
photo->next = NULL;
return photo;
}
// 将照片添加到链表尾部
void addPhoto(Photo** head, char name[], char date[]) {
if (*head == NULL) {
*head = createPhoto(name, date);
return;
}
Photo* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = createPhoto(name, date);
}
// 打印链表中的所有照片信息
void printPhotos(Photo* head) {
Photo* current = head;
while (current != NULL) {
printf("照片名: %s\n", current->name);
printf("拍摄日期: %s\n", current->date);
printf("--------------------\n");
current = current->next;
}
}
int main() {
Photo* head = NULL;
// 添加几张照片
addPhoto(&head, "photo1.jpg", "2021-01-01");
addPhoto(&head, "photo2.jpg", "2021-02-15");
addPhoto(&head, "photo3.jpg", "2021-03-30");
// 打印照片信息
printPhotos(head);
// 释放链表内存
Photo* current = head;
while (current != NULL) {
Photo* next = current->next;
free(current);
current = next;
}
return 0;
}
```
请注意,这只是一个简单的示例,你可以根据实际需求进行扩展和修改。在实际的应用中,你可能需要添加更多的操作,比如删除照片、浏览照片等。同时,还需要考虑如何在开发板上显示照片和与用户交互的方式,这可能需要使用相关的图形库和输入设备驱动。
阅读全文
相关推荐
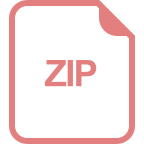

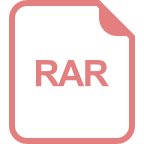
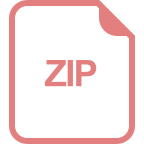
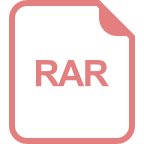
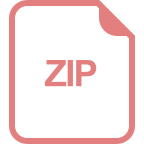
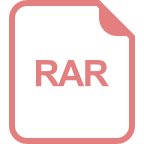
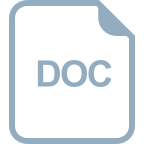
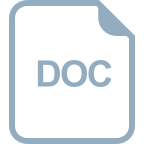

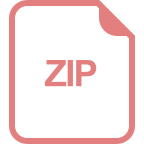
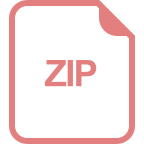