用Python写一个烟花小程序
时间: 2024-04-12 17:23:00 浏览: 28
当然可以!下面是一个用Python编写的简单烟花小程序的示例:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("烟花小程序")
# 定义烟花类
class Firework:
def __init__(self, x, y):
self.x = x
self.y = y
self.color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
self.radius = 3
self.speed = random.randint(1, 5)
self.exploded = False
def move(self):
if not self.exploded:
self.y -= self.speed
def explode(self):
self.exploded = True
for _ in range(100):
sparks.append(Spark(self.x, self.y, self.color))
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
# 定义火花类
class Spark:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
self.radius = 2
self.angle = random.uniform(0, 2 * math.pi)
self.speed = random.uniform(1, 5)
def move(self):
self.x += math.cos(self.angle) * self.speed
self.y += math.sin(self.angle) * self.speed
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
# 创建烟花和火花列表
fireworks = []
sparks = []
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 生成新的烟花
if random.random() < 0.01:
fireworks.append(Firework(random.randint(0, screen_width), screen_height))
# 更新烟花和火花的位置
for firework in fireworks:
firework.move()
if firework.y < 200 and not firework.exploded:
firework.explode()
for spark in sparks:
spark.move()
# 绘制烟花和火花
screen.fill((0, 0, 0))
for firework in fireworks:
firework.draw()
for spark in sparks:
spark.draw()
# 移除已经消失的火花
sparks = [spark for spark in sparks if spark.radius > 0]
# 更新屏幕显示
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这个程序使用了Pygame库来创建窗口和处理图形绘制。它通过不断生成新的烟花对象,并在一定高度上爆炸成火花对象来模拟烟花效果。每个烟花和火花对象都有自己的位置、颜色、半径和速度等属性,通过不断更新和绘制它们来实现动画效果。
希望这个示例能帮助到你!如果你有任何相关问题,请随时提问。
相关推荐
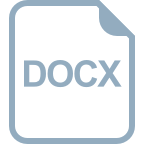
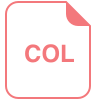
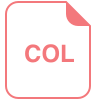
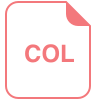
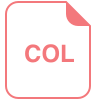
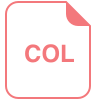









