写一个Python的烟花的程序
时间: 2023-10-07 22:10:24 浏览: 86
好的,以下是一个使用Python实现的简单烟花程序的示例代码。该程序使用pygame库实现图形界面,通过不断更新烟花粒子的位置和颜色,来模拟烟花的效果。
```python
import sys
import random
import pygame
# 初始化pygame
pygame.init()
# 设置窗口大小
size = width, height = 800, 600
# 创建窗口
screen = pygame.display.set_mode(size)
# 定义烟花粒子类
class Particle():
def __init__(self, x, y):
self.x = x
self.y = y
self.color = (random.randint(50, 255), random.randint(50, 255), random.randint(50, 255))
self.radius = 2
self.speed = random.randint(1, 5)
self.angle = random.uniform(0, 2 * math.pi)
self.vx = self.speed * math.cos(self.angle)
self.vy = self.speed * math.sin(self.angle)
self.gravity = 0.1
def move(self):
self.x += self.vx
self.y += self.vy
self.vy += self.gravity
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.radius)
# 定义烟花类
class Firework():
def __init__(self, x, y):
self.x = x
self.y = y
self.particles = []
self.exploded = False
self.color = (random.randint(50, 255), random.randint(50, 255), random.randint(50, 255))
for i in range(50):
self.particles.append(Particle(self.x, self.y))
def explode(self):
for particle in self.particles:
particle.gravity = 0.05
particle.speed = random.randint(1, 10)
particle.angle = random.uniform(0, 2 * math.pi)
particle.vx = particle.speed * math.cos(particle.angle)
particle.vy = particle.speed * math.sin(particle.angle)
def move(self):
if not self.exploded:
self.y -= 3
if self.y <= 300:
self.exploded = True
self.explode()
else:
all_dead = True
for particle in self.particles:
if particle.y < height:
all_dead = False
particle.move()
particle.draw()
if all_dead:
fireworks.remove(self)
def draw(self):
if not self.exploded:
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), 3)
else:
for particle in self.particles:
particle.draw()
# 创建烟花列表
fireworks = []
# 游戏循环
while True:
# 处理退出事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
# 产生新的烟花
if random.randint(1, 50) == 1:
fireworks.append(Firework(random.randint(50, width - 50), height))
# 更新烟花的位置
for firework in fireworks:
firework.move()
# 更新屏幕
screen.fill((0, 0, 0))
for firework in fireworks:
firework.draw()
pygame.display.flip()
```
运行该代码后,即可看到烟花效果。需要注意的是,该程序中使用的是pygame库,需要先安装才能运行。
阅读全文
相关推荐
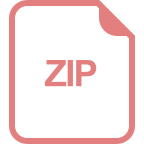
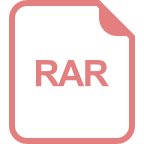
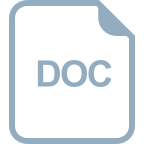











