c语言编写一个程序,当接收到一个句子时,识别并提取出所有连续出现两次及以上首字母为大写的单词(即被视为术语),然后输出每个术语的首字母组成的大写缩写,每个术语占一行。
时间: 2024-12-22 14:29:23 浏览: 17
要编写一个C语言程序来完成这个任务,首先需要对输入字符串进行分词处理,找出连续的大写字母,并记录它们构成的缩写。以下是一个简单的示例程序,它使用了`ctype.h`库来进行字符分类:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
// 辅助函数,判断是否为大写字母
int is_uppercase(char c) {
return isupper(c);
}
// 分割单词并检查是否连续的大写字母
void extract_terms(const char *str) {
int term_start = 0;
for (size_t i = 0; str[i]; i++) {
if (is_uppercase(str[i])) { // 遇到大写字母
if (i > 0 && is_uppercase(str[i - 1])) { // 检查前一个字符是否也是大写
// 记录起始位置和当前长度
term_start = i - 1;
int term_length = 1;
while (i + term_length < strlen(str) && is_uppercase(str[i + term_length])) {
term_length++;
}
// 提取术语并打印缩写
printf("%.*s\n", term_length, str + term_start);
} else {
term_start = i; // 一个新的术语开始
}
}
}
}
int main() {
char sentence[1000];
printf("请输入句子:");
fgets(sentence, sizeof(sentence), stdin); // 注意去掉末尾的换行符
sentence[strcspn(sentence, "\n")] = '\0'; // 去掉换行符
extract_terms(sentence);
return 0;
}
```
在这个程序中,`extract_terms`函数遍历输入的字符串,每当遇到一个大写字母并且上一个字符同样是大写字母时,就认为找到了一个术语。它会计算连续大写字母的数量并打印出来。
运行此程序时,按照提示输入句子,然后程序将输出每个找到的术语及其首字母组成的缩写,每行一个。
阅读全文
相关推荐
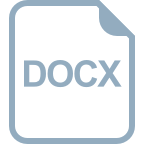
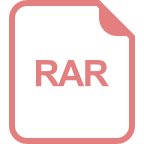
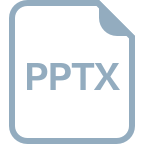
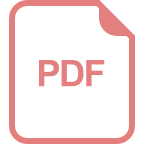
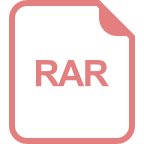
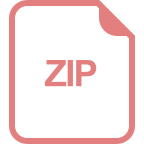
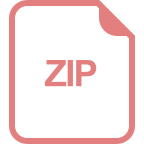