Pugixml封装一个类重载=读取和写入节点属性
时间: 2024-04-15 11:31:12 浏览: 20
下面是一个示例类,演示了如何封装一个支持重载赋值运算符、读取和写入节点属性的类,使用Pugixml库:
```cpp
#include <iostream>
#include <string>
#include "pugixml.hpp"
class XMLNode {
public:
XMLNode(const std::string& filePath) {
document.load_file(filePath.c_str());
}
// 重载赋值运算符
XMLNode& operator=(const XMLNode& other) {
if (this != &other) {
document.reset();
document = other.document;
}
return *this;
}
template<typename T>
T getProperty(const std::string& nodeName, const std::string& attributeName) {
pugi::xml_node node = document.child(nodeName.c_str());
if (node) {
pugi::xml_attribute attr = node.attribute(attributeName.c_str());
if (attr) {
return attr.as_string();
}
}
// 返回默认值或抛出异常,根据需要自行决定
return T();
}
template<typename T>
void setProperty(const std::string& nodeName, const std::string& attributeName, const T& value) {
pugi::xml_node node = document.child(nodeName.c_str());
if (!node) {
// 创建新节点
node = document.append_child(nodeName.c_str());
}
node.append_attribute(attributeName.c_str()).set_value(value);
}
void save(const std::string& filePath) {
document.save_file(filePath.c_str());
}
private:
pugi::xml_document document;
};
int main() {
XMLNode xmlNode1("example.xml");
// 读取属性
std::string author = xmlNode1.getProperty<std::string>("book", "author");
int year = xmlNode1.getProperty<int>("book", "year");
std::cout << "Author: " << author << std::endl;
std::cout << "Year: " << year << std::endl;
// 创建新的XMLNode对象并赋值
XMLNode xmlNode2("another_example.xml");
xmlNode2 = xmlNode1;
// 修改属性
xmlNode2.setProperty("book", "author", "John Doe");
xmlNode2.setProperty("book", "year", 2022);
// 保存修改后的内容
xmlNode2.save("modified_example.xml");
return 0;
}
```
在这个示例中,XMLNode类封装了一个pugixml的xml_document对象,并提供了getProperty()和setProperty()成员函数来读取和写入节点属性。它还重载了赋值运算符,使得一个对象可以将其数据赋值给另一个对象。在main函数中,我们创建了两个XMLNode对象,分别加载了不同的XML文件,并演示了如何读取属性、修改属性、赋值和保存修改后的内容。
请注意,这只是一个简单的示例,你可以根据自己的需求对XMLNode类进行扩展和修改。同时,需要注意Pugixml的内存管理和异常处理等问题。
相关推荐
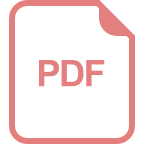
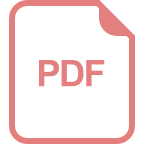
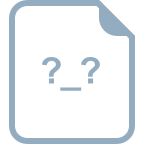








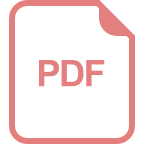
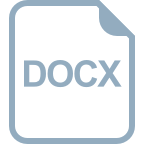
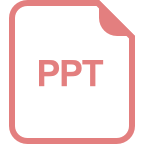
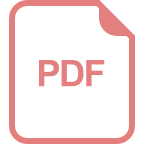
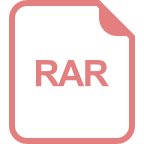
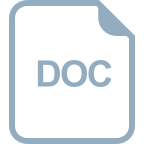
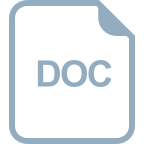
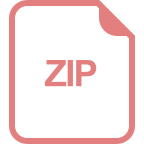