c++代码是什么
时间: 2023-07-31 09:10:12 浏览: 79
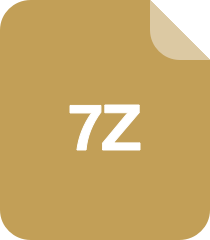
c++代码 c++代码 c++代码
以下是使用 C++ 语言实现的代码:
```cpp
#include <iostream>
#include <stack>
#include <vector>
#include <cstring>
using namespace std;
int main() {
char s[500];
cin.getline(s, 500);
int len = strlen(s);
stack<int> num; // 存放数字的栈
vector<int> ans; // 存放计算结果的数组
for (int i = 0; i < len; i++) {
if (s[i] >= '0' && s[i] <= '9') {
int x = 0;
while (i < len && s[i] >= '0' && s[i] <= '9') {
x = x * 10 + (s[i] - '0');
i++;
}
i--;
num.push(x);
} else if (s[i] == '+') {
int a = num.top();
num.pop();
int b = num.top();
num.pop();
num.push(b + a);
} else if (s[i] == '-') {
int a = num.top();
num.pop();
int b = num.top();
num.pop();
num.push(b - a);
} else if (s[i] == '*') {
int a = num.top();
num.pop();
int b = num.top();
num.pop();
num.push(b * a);
}
}
while (!num.empty()) {
ans.push_back(num.top());
num.pop();
}
for (int i = ans.size() - 1; i >= 0; i--) {
cout << ans[i] << endl;
}
return 0;
}
```
这个代码使用了 STL 中的栈和向量,实现了与 Python 版本相同的功能。需要注意的是,在 C++ 中,需要使用 `cin.getline()` 函数来读入带空格的字符串。读入后,对字符串进行遍历,如果遇到数字则将其转换为整数并入栈,否则就从栈中弹出两个数字进行运算,再将结果入栈。最终,将栈中剩余的数字依次保存到一个向量中,并倒序输出即可。
需要注意的是,由于题目中规定了运算符只有加、减、乘三种,因此这个代码只考虑了这三种运算符,如果需要支持更多运算符,可以在字典中添加对应的运算函数即可。
阅读全文
相关推荐















