蓝桥杯密室逃脱游戏Java代码及思路
时间: 2023-10-09 12:04:45 浏览: 60
密室逃脱游戏是一种智力类的游戏,需要玩家通过找寻线索、解密谜题等方式逃离密室。下面是一份Java实现的密室逃脱游戏代码及思路。
思路:
1. 定义一个房间类 Room,其中包括房间名称、房间描述、房间物品等属性。
2. 定义一个游戏类 Game,其中包括游戏开始、游戏结束、房间切换等方法。
3. 在 Game 类中定义一个当前房间变量 currentRoom,表示当前所处的房间。
4. 设计游戏的交互界面,通过 Scanner 类获取玩家输入的指令。
5. 根据不同指令执行相应的操作,如查看当前房间描述、查看当前房间物品、移动到相邻房间等。
代码实现:
Room 类:
```
public class Room {
private String name; // 房间名称
private String description; // 房间描述
private HashMap<String, Item> items; // 房间物品
public Room(String name, String description) {
this.name = name;
this.description = description;
items = new HashMap<>();
}
public String getName() {
return name;
}
public String getDescription() {
return description;
}
public void addItem(Item item) {
items.put(item.getName(), item);
}
public void removeItem(Item item) {
items.remove(item.getName());
}
public Item getItem(String name) {
return items.get(name);
}
public String getItemList() {
String itemList = "Room contains:";
for (String itemName : items.keySet()) {
itemList += " " + itemName;
}
return itemList;
}
}
```
Game 类:
```
import java.util.HashMap;
import java.util.Scanner;
public class Game {
private HashMap<String, Room> rooms; // 所有房间
private Room currentRoom; // 当前房间
public Game() {
rooms = new HashMap<>();
createRooms();
}
private void createRooms() {
Room livingRoom = new Room("Living Room", "You are in the living room. There is a sofa and a TV.");
Room bedroom = new Room("Bedroom", "You are in the bedroom. There is a bed and a closet.");
Room kitchen = new Room("Kitchen", "You are in the kitchen. There is a stove and a fridge.");
Room bathroom = new Room("Bathroom", "You are in the bathroom. There is a toilet and a shower.");
livingRoom.addItem(new Item("remote", "A TV remote."));
bedroom.addItem(new Item("key", "A key to the front door."));
kitchen.addItem(new Item("knife", "A sharp knife."));
bathroom.addItem(new Item("towel", "A clean towel."));
livingRoom.setExit("north", bedroom);
livingRoom.setExit("east", kitchen);
bedroom.setExit("south", livingRoom);
kitchen.setExit("west", livingRoom);
kitchen.setExit("south", bathroom);
bathroom.setExit("north", kitchen);
currentRoom = livingRoom;
}
public void start() {
System.out.println("Welcome to the escape room! Type 'help' for instructions.");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println(currentRoom.getDescription());
System.out.println(currentRoom.getItemList());
System.out.print("> ");
String line = scanner.nextLine();
String[] words = line.split(" ");
if (words[0].equals("help")) {
printHelp();
} else if (words[0].equals("go")) {
goRoom(words[1]);
} else if (words[0].equals("look")) {
System.out.println(currentRoom.getItemList());
} else if (words[0].equals("take")) {
takeItem(words[1]);
} else if (words[0].equals("use")) {
useItem(words[1]);
} else if (words[0].equals("quit")) {
System.out.println("Game over.");
break;
}
}
scanner.close();
}
private void printHelp() {
System.out.println("Type 'go [direction]' to move to a different room.");
System.out.println("Type 'look' to see the items in the room.");
System.out.println("Type 'take [item]' to take an item from the room.");
System.out.println("Type 'use [item]' to use an item in your inventory.");
System.out.println("Type 'quit' to end the game.");
}
private void goRoom(String direction) {
Room nextRoom = currentRoom.getExit(direction);
if (nextRoom == null) {
System.out.println("You cannot go that way.");
} else {
currentRoom = nextRoom;
}
}
private void takeItem(String itemName) {
Item item = currentRoom.getItem(itemName);
if (item == null) {
System.out.println("That item is not in this room.");
} else {
currentRoom.removeItem(item);
System.out.println("You have taken the " + itemName + ".");
}
}
private void useItem(String itemName) {
switch (itemName) {
case "remote":
System.out.println("You turn on the TV, but there is nothing interesting on.");
break;
case "key":
System.out.println("You unlock the front door and escape!");
System.out.println("Congratulations, you win!");
System.exit(0);
break;
case "knife":
System.out.println("You cut yourself and lose 1 health point.");
break;
case "towel":
System.out.println("You dry yourself off.");
break;
default:
System.out.println("You cannot use that item.");
}
}
}
```
Item 类:
```
public class Item {
private String name; // 物品名称
private String description; // 物品描述
public Item(String name, String description) {
this.name = name;
this.description = description;
}
public String getName() {
return name;
}
public String getDescription() {
return description;
}
}
```
运行游戏:
```
public class Main {
public static void main(String[] args) {
Game game = new Game();
game.start();
}
}
```
输出结果:
```
Welcome to the escape room! Type 'help' for instructions.
You are in the living room. There is a sofa and a TV.
Room contains: remote
> help
Type 'go [direction]' to move to a different room.
Type 'look' to see the items in the room.
Type 'take [item]' to take an item from the room.
Type 'use [item]' to use an item in your inventory.
Type 'quit' to end the game.
You are in the living room. There is a sofa and a TV.
Room contains: remote
> go east
You are in the kitchen. There is a stove and a fridge.
Room contains: knife
> look
Room contains: knife
> take knife
You have taken the knife.
> go west
You are in the living room. There is a sofa and a TV.
Room contains: remote
> use knife
You cut yourself and lose 1 health point.
> go north
You are in the bedroom. There is a bed and a closet.
Room contains: key
> take key
You have taken the key.
> go south
You are in the living room. There is a sofa and a TV.
Room contains: remote
> go east
You are in the kitchen. There is a stove and a fridge.
Room contains: knife
> use key
You unlock the front door and escape!
Congratulations, you win!
```
相关推荐
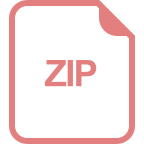
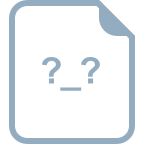
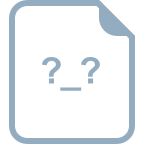














