c++密室逃脱游戏代码
时间: 2024-08-12 09:06:19 浏览: 87
创建一个完整的C++密室逃脱游戏涉及到大量的编程工作,包括游戏逻辑、用户界面、场景设计等多个方面。由于这需要深入的知识和篇幅较长,我会提供一个简单的概念性的框架作为起点:
```cpp
#include <iostream>
#include <string>
class Room {
public:
std::string description;
std::string exit;
// 添加描述、查看描述等方法
void setDescription(const std::string& desc) { description = desc; }
void setExit(const std::string& e) { exit = e; }
virtual std::string play() {
return "You are in a room with the following description: " + description + ". The exit is at " + exit + ".";
}
};
// 子类实现特定房间
class EscapeRoom : public Room {
private:
bool keyFound;
public:
// 添加钥匙找寻功能
void findKey() { keyFound = true; }
// 如果找到钥匙,可以改变play方法的行为
std::string play() override {
if (keyFound) {
return "With the key, you can now escape through the door.";
} else {
return super::play();
}
}
};
int main() {
EscapeRoom room;
room.setDescription("A locked room with a mysterious object on the table.");
room.setExit("north");
std::cout << room.play() << std::endl;
// 检查并尝试打开门
if (!room.keyFound) {
room.findKey(); // 假设玩家找到了钥匙
}
std::cout << room.play() << std::endl;
return 0;
}
```
这是一个非常基础的例子,实际游戏中你会需要更复杂的设计,比如事件驱动系统、图形用户界面(如果需要)、谜题解决机制等等。要继续这个项目,你可以学习如何处理用户输入、使用类和对象管理状态、以及构建递归或循环结构以模拟解谜过程。
阅读全文
相关推荐
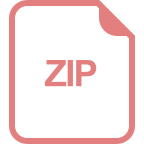
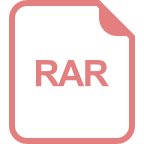
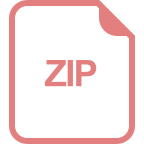
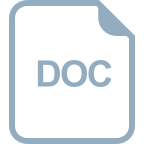
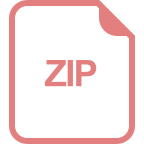
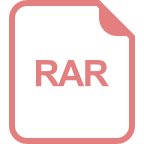
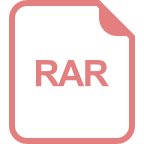
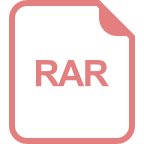
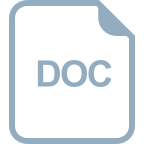
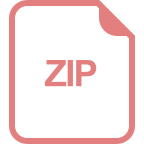
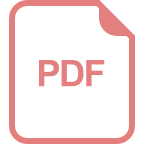
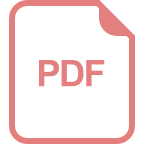
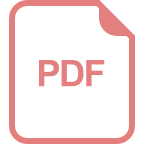
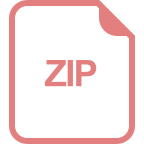
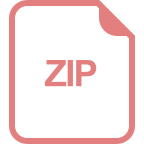
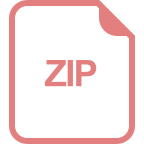